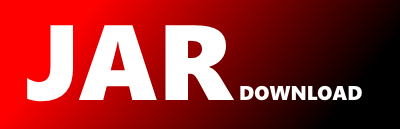
com.pulumi.awsnative.configuration.kotlin.ConfigRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.configuration.kotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.ComplianceProperties
import com.pulumi.awsnative.configuration.kotlin.outputs.ConfigRuleEvaluationModeConfiguration
import com.pulumi.awsnative.configuration.kotlin.outputs.ConfigRuleScope
import com.pulumi.awsnative.configuration.kotlin.outputs.ConfigRuleSource
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.configuration.kotlin.outputs.ComplianceProperties.Companion.toKotlin as compliancePropertiesToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.ConfigRuleEvaluationModeConfiguration.Companion.toKotlin as configRuleEvaluationModeConfigurationToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.ConfigRuleScope.Companion.toKotlin as configRuleScopeToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.ConfigRuleSource.Companion.toKotlin as configRuleSourceToKotlin
/**
* Builder for [ConfigRule].
*/
@PulumiTagMarker
public class ConfigRuleResourceBuilder internal constructor() {
public var name: String? = null
public var args: ConfigRuleArgs = ConfigRuleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ConfigRuleArgsBuilder.() -> Unit) {
val builder = ConfigRuleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ConfigRule {
val builtJavaResource = com.pulumi.awsnative.configuration.ConfigRule(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ConfigRule(builtJavaResource)
}
}
/**
* You must first create and start the CC configuration recorder in order to create CC managed rules with CFNlong. For more information, see [Managing the Configuration Recorder](https://docs.aws.amazon.com/config/latest/developerguide/stop-start-recorder.html).
* Adds or updates an CC rule to evaluate if your AWS resources comply with your desired configurations. For information on how many CC rules you can have per account, see [Service Limits](https://docs.aws.amazon.com/config/latest/developerguide/configlimits.html) in the *Developer Guide*.
* There are two types of rules: *Managed Rules* and *Custom Rules*. You can use the ``ConfigRule`` resource to create both CC Managed Rules and CC Custom Rules.
* CC Managed Rules are predefined, customizable rules created by CC. For a list of managed rules, see [List of Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html). If you are adding an CC managed rule, you must specify the rule's identifier for the ``SourceIdentifier`` key.
* CC Custom Rules are rules that you create from scratch. There are two ways to create CC custom rules: with Lambda functions ([Developer Guide](https://docs.aws.amazon.com/config/latest/developerguide/gettingstarted-concepts.html#gettingstarted-concepts-function)) and with CFNGUARDshort ([Guard GitHub Repository](https://docs.aws.amazon.com/https://github.com/aws-cloudformation/cloudformation-guard)), a policy-as-code language. CC custom rules created with LAMlong are called *Custom Lambda Rules* and CC custom rules created with CFNGUARDshort are called *Custom Policy Rules*.
* If you are adding a new CC Custom LAM rule, you first need to create an LAMlong function that the rule invokes to evaluate your resources. When you use the ``ConfigRule`` resource to add a Custom LAM rule to CC, you must specify the Amazon Resource Name (ARN) that LAMlong assigns to the function. You specify the ARN in the ``SourceIdentifier`` key. This key is part of the ``Source`` object, which is part of the ``ConfigRule`` object.
* For any new CC rule that you add, specify the ``ConfigRuleName`` in the ``ConfigRule`` object. Do not specify the ``ConfigRuleArn`` or the ``ConfigRuleId``. These values are generated by CC for new rules.
* If you are updating a rule that you added previously, you can specify the rule by ``ConfigRuleName``, ``ConfigRuleId``, or ``ConfigRuleArn`` in the ``ConfigRule`` data type that you use in this request.
* For more information about developing and using CC rules, see [Evaluating Resources with Rules](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config.html) in the *Developer Guide*.
*/
public class ConfigRule internal constructor(
override val javaResource: com.pulumi.awsnative.configuration.ConfigRule,
) : KotlinCustomResource(javaResource, ConfigRuleMapper) {
/**
* The Amazon Resource Name (ARN) of the AWS Config rule, such as `arn:aws:config:us-east-1:123456789012:config-rule/config-rule-a1bzhi` .
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Indicates whether an AWS resource or CC rule is compliant and provides the number of contributors that affect the compliance.
*/
public val compliance: Output?
get() = javaResource.compliance().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
compliancePropertiesToKotlin(args0)
})
}).orElse(null)
})
/**
* The ID of the AWS Config rule, such as `config-rule-a1bzhi` .
*/
public val configRuleId: Output
get() = javaResource.configRuleId().applyValue({ args0 -> args0 })
/**
* A name for the CC rule. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the rule name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
*/
public val configRuleName: Output?
get() = javaResource.configRuleName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The description that you provide for the CC rule.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The modes the CC rule can be evaluated in. The valid values are distinct objects. By default, the value is Detective evaluation mode only.
*/
public val evaluationModes: Output>?
get() = javaResource.evaluationModes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
configRuleEvaluationModeConfigurationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* A string, in JSON format, that is passed to the CC rule Lambda function.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::Config::ConfigRule` for more information about the expected schema for this property.
*/
public val inputParameters: Output?
get() = javaResource.inputParameters().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum frequency with which CC runs evaluations for a rule. You can specify a value for ``MaximumExecutionFrequency`` when:
* + You are using an AWS managed rule that is triggered at a periodic frequency.
* + Your custom rule is triggered when CC delivers the configuration snapshot. For more information, see [ConfigSnapshotDeliveryProperties](https://docs.aws.amazon.com/config/latest/APIReference/API_ConfigSnapshotDeliveryProperties.html).
* By default, rules with a periodic trigger are evaluated every 24 hours. To change the frequency, specify a valid value for the ``MaximumExecutionFrequency`` parameter.
*/
public val maximumExecutionFrequency: Output?
get() = javaResource.maximumExecutionFrequency().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Defines which resources can trigger an evaluation for the rule. The scope can include one or more resource types, a combination of one resource type and one resource ID, or a combination of a tag key and value. Specify a scope to constrain the resources that can trigger an evaluation for the rule. If you do not specify a scope, evaluations are triggered when any resource in the recording group changes.
* The scope can be empty.
*/
public val scope: Output?
get() = javaResource.scope().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
configRuleScopeToKotlin(args0)
})
}).orElse(null)
})
/**
* Provides the rule owner (```` for managed rules, ``CUSTOM_POLICY`` for Custom Policy rules, and ``CUSTOM_LAMBDA`` for Custom Lambda rules), the rule identifier, and the notifications that cause the function to evaluate your AWS resources.
*/
public val source: Output
get() = javaResource.source().applyValue({ args0 ->
args0.let({ args0 ->
configRuleSourceToKotlin(args0)
})
})
}
public object ConfigRuleMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.configuration.ConfigRule::class == javaResource::class
override fun map(javaResource: Resource): ConfigRule = ConfigRule(
javaResource as
com.pulumi.awsnative.configuration.ConfigRule,
)
}
/**
* @see [ConfigRule].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ConfigRule].
*/
public suspend fun configRule(name: String, block: suspend ConfigRuleResourceBuilder.() -> Unit): ConfigRule {
val builder = ConfigRuleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ConfigRule].
* @param name The _unique_ name of the resulting resource.
*/
public fun configRule(name: String): ConfigRule {
val builder = ConfigRuleResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy