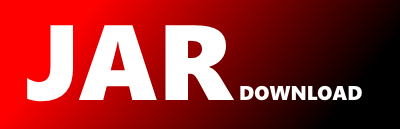
com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleSourceDetailArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.configuration.kotlin.inputs
import com.pulumi.awsnative.configuration.inputs.ConfigRuleSourceDetailArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides the source and the message types that trigger CC to evaluate your AWS resources against a rule. It also provides the frequency with which you want CC to run evaluations for the rule if the trigger type is periodic. You can specify the parameter values for ``SourceDetail`` only for custom rules.
* @property eventSource The source of the event, such as an AWS service, that triggers CC to evaluate your AWS resources.
* @property maximumExecutionFrequency The frequency at which you want CC to run evaluations for a custom rule with a periodic trigger. If you specify a value for ``MaximumExecutionFrequency``, then ``MessageType`` must use the ``ScheduledNotification`` value.
* By default, rules with a periodic trigger are evaluated every 24 hours. To change the frequency, specify a valid value for the ``MaximumExecutionFrequency`` parameter.
* Based on the valid value you choose, CC runs evaluations once for each valid value. For example, if you choose ``Three_Hours``, CC runs evaluations once every three hours. In this case, ``Three_Hours`` is the frequency of this rule.
* @property messageType The type of notification that triggers CC to run an evaluation for a rule. You can specify the following notification types:
* + ``ConfigurationItemChangeNotification`` - Triggers an evaluation when CC delivers a configuration item as a result of a resource change.
* + ``OversizedConfigurationItemChangeNotification`` - Triggers an evaluation when CC delivers an oversized configuration item. CC may generate this notification type when a resource changes and the notification exceeds the maximum size allowed by Amazon SNS.
* + ``ScheduledNotification`` - Triggers a periodic evaluation at the frequency specified for ``MaximumExecutionFrequency``.
* + ``ConfigurationSnapshotDeliveryCompleted`` - Triggers a periodic evaluation when CC delivers a configuration snapshot.
* If you want your custom rule to be triggered by configuration changes, specify two SourceDetail objects, one for ``ConfigurationItemChangeNotification`` and one for ``OversizedConfigurationItemChangeNotification``.
*/
public data class ConfigRuleSourceDetailArgs(
public val eventSource: Output,
public val maximumExecutionFrequency: Output? = null,
public val messageType: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.configuration.inputs.ConfigRuleSourceDetailArgs =
com.pulumi.awsnative.configuration.inputs.ConfigRuleSourceDetailArgs.builder()
.eventSource(eventSource.applyValue({ args0 -> args0 }))
.maximumExecutionFrequency(maximumExecutionFrequency?.applyValue({ args0 -> args0 }))
.messageType(messageType.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConfigRuleSourceDetailArgs].
*/
@PulumiTagMarker
public class ConfigRuleSourceDetailArgsBuilder internal constructor() {
private var eventSource: Output? = null
private var maximumExecutionFrequency: Output? = null
private var messageType: Output? = null
/**
* @param value The source of the event, such as an AWS service, that triggers CC to evaluate your AWS resources.
*/
@JvmName("wkancokmydnbjkin")
public suspend fun eventSource(`value`: Output) {
this.eventSource = value
}
/**
* @param value The frequency at which you want CC to run evaluations for a custom rule with a periodic trigger. If you specify a value for ``MaximumExecutionFrequency``, then ``MessageType`` must use the ``ScheduledNotification`` value.
* By default, rules with a periodic trigger are evaluated every 24 hours. To change the frequency, specify a valid value for the ``MaximumExecutionFrequency`` parameter.
* Based on the valid value you choose, CC runs evaluations once for each valid value. For example, if you choose ``Three_Hours``, CC runs evaluations once every three hours. In this case, ``Three_Hours`` is the frequency of this rule.
*/
@JvmName("mxxirqqingwnclge")
public suspend fun maximumExecutionFrequency(`value`: Output) {
this.maximumExecutionFrequency = value
}
/**
* @param value The type of notification that triggers CC to run an evaluation for a rule. You can specify the following notification types:
* + ``ConfigurationItemChangeNotification`` - Triggers an evaluation when CC delivers a configuration item as a result of a resource change.
* + ``OversizedConfigurationItemChangeNotification`` - Triggers an evaluation when CC delivers an oversized configuration item. CC may generate this notification type when a resource changes and the notification exceeds the maximum size allowed by Amazon SNS.
* + ``ScheduledNotification`` - Triggers a periodic evaluation at the frequency specified for ``MaximumExecutionFrequency``.
* + ``ConfigurationSnapshotDeliveryCompleted`` - Triggers a periodic evaluation when CC delivers a configuration snapshot.
* If you want your custom rule to be triggered by configuration changes, specify two SourceDetail objects, one for ``ConfigurationItemChangeNotification`` and one for ``OversizedConfigurationItemChangeNotification``.
*/
@JvmName("jmvnkhycvjxhqmmh")
public suspend fun messageType(`value`: Output) {
this.messageType = value
}
/**
* @param value The source of the event, such as an AWS service, that triggers CC to evaluate your AWS resources.
*/
@JvmName("hicyuaaetbciekug")
public suspend fun eventSource(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.eventSource = mapped
}
/**
* @param value The frequency at which you want CC to run evaluations for a custom rule with a periodic trigger. If you specify a value for ``MaximumExecutionFrequency``, then ``MessageType`` must use the ``ScheduledNotification`` value.
* By default, rules with a periodic trigger are evaluated every 24 hours. To change the frequency, specify a valid value for the ``MaximumExecutionFrequency`` parameter.
* Based on the valid value you choose, CC runs evaluations once for each valid value. For example, if you choose ``Three_Hours``, CC runs evaluations once every three hours. In this case, ``Three_Hours`` is the frequency of this rule.
*/
@JvmName("tercgblquyporgnl")
public suspend fun maximumExecutionFrequency(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumExecutionFrequency = mapped
}
/**
* @param value The type of notification that triggers CC to run an evaluation for a rule. You can specify the following notification types:
* + ``ConfigurationItemChangeNotification`` - Triggers an evaluation when CC delivers a configuration item as a result of a resource change.
* + ``OversizedConfigurationItemChangeNotification`` - Triggers an evaluation when CC delivers an oversized configuration item. CC may generate this notification type when a resource changes and the notification exceeds the maximum size allowed by Amazon SNS.
* + ``ScheduledNotification`` - Triggers a periodic evaluation at the frequency specified for ``MaximumExecutionFrequency``.
* + ``ConfigurationSnapshotDeliveryCompleted`` - Triggers a periodic evaluation when CC delivers a configuration snapshot.
* If you want your custom rule to be triggered by configuration changes, specify two SourceDetail objects, one for ``ConfigurationItemChangeNotification`` and one for ``OversizedConfigurationItemChangeNotification``.
*/
@JvmName("drcyvpsimqqtfqdm")
public suspend fun messageType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.messageType = mapped
}
internal fun build(): ConfigRuleSourceDetailArgs = ConfigRuleSourceDetailArgs(
eventSource = eventSource ?: throw PulumiNullFieldException("eventSource"),
maximumExecutionFrequency = maximumExecutionFrequency,
messageType = messageType ?: throw PulumiNullFieldException("messageType"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy