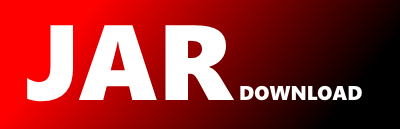
com.pulumi.awsnative.customerprofiles.kotlin.inputs.DomainAutoMergingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.customerprofiles.kotlin.inputs
import com.pulumi.awsnative.customerprofiles.inputs.DomainAutoMergingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Configuration information about the auto-merging process.
* @property conflictResolution Determines how the auto-merging process should resolve conflicts between different profiles. For example, if Profile A and Profile B have the same `FirstName` and `LastName` , `ConflictResolution` specifies which `EmailAddress` should be used.
* @property consolidation A list of matching attributes that represent matching criteria. If two profiles meet at least one of the requirements in the matching attributes list, they will be merged.
* @property enabled The flag that enables the auto-merging of duplicate profiles.
* @property minAllowedConfidenceScoreForMerging A number between 0 and 1 that represents the minimum confidence score required for profiles within a matching group to be merged during the auto-merge process. A higher score means higher similarity required to merge profiles.
*/
public data class DomainAutoMergingArgs(
public val conflictResolution: Output? = null,
public val consolidation: Output? = null,
public val enabled: Output,
public val minAllowedConfidenceScoreForMerging: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.customerprofiles.inputs.DomainAutoMergingArgs =
com.pulumi.awsnative.customerprofiles.inputs.DomainAutoMergingArgs.builder()
.conflictResolution(
conflictResolution?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.consolidation(consolidation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.enabled(enabled.applyValue({ args0 -> args0 }))
.minAllowedConfidenceScoreForMerging(
minAllowedConfidenceScoreForMerging?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [DomainAutoMergingArgs].
*/
@PulumiTagMarker
public class DomainAutoMergingArgsBuilder internal constructor() {
private var conflictResolution: Output? = null
private var consolidation: Output? = null
private var enabled: Output? = null
private var minAllowedConfidenceScoreForMerging: Output? = null
/**
* @param value Determines how the auto-merging process should resolve conflicts between different profiles. For example, if Profile A and Profile B have the same `FirstName` and `LastName` , `ConflictResolution` specifies which `EmailAddress` should be used.
*/
@JvmName("hodmimxslbvjwilm")
public suspend fun conflictResolution(`value`: Output) {
this.conflictResolution = value
}
/**
* @param value A list of matching attributes that represent matching criteria. If two profiles meet at least one of the requirements in the matching attributes list, they will be merged.
*/
@JvmName("qrjdnhurmpswgkqv")
public suspend fun consolidation(`value`: Output) {
this.consolidation = value
}
/**
* @param value The flag that enables the auto-merging of duplicate profiles.
*/
@JvmName("kplyfydkbygspvtm")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value A number between 0 and 1 that represents the minimum confidence score required for profiles within a matching group to be merged during the auto-merge process. A higher score means higher similarity required to merge profiles.
*/
@JvmName("ytuqbpmyyelpurmt")
public suspend fun minAllowedConfidenceScoreForMerging(`value`: Output) {
this.minAllowedConfidenceScoreForMerging = value
}
/**
* @param value Determines how the auto-merging process should resolve conflicts between different profiles. For example, if Profile A and Profile B have the same `FirstName` and `LastName` , `ConflictResolution` specifies which `EmailAddress` should be used.
*/
@JvmName("tdksulhiopvgrbtg")
public suspend fun conflictResolution(`value`: DomainConflictResolutionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conflictResolution = mapped
}
/**
* @param argument Determines how the auto-merging process should resolve conflicts between different profiles. For example, if Profile A and Profile B have the same `FirstName` and `LastName` , `ConflictResolution` specifies which `EmailAddress` should be used.
*/
@JvmName("mupmcwemxpitywon")
public suspend fun conflictResolution(argument: suspend DomainConflictResolutionArgsBuilder.() -> Unit) {
val toBeMapped = DomainConflictResolutionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.conflictResolution = mapped
}
/**
* @param value A list of matching attributes that represent matching criteria. If two profiles meet at least one of the requirements in the matching attributes list, they will be merged.
*/
@JvmName("xodenttsvcmyxida")
public suspend fun consolidation(`value`: DomainConsolidationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consolidation = mapped
}
/**
* @param argument A list of matching attributes that represent matching criteria. If two profiles meet at least one of the requirements in the matching attributes list, they will be merged.
*/
@JvmName("pctjmyrpoygsaehy")
public suspend fun consolidation(argument: suspend DomainConsolidationArgsBuilder.() -> Unit) {
val toBeMapped = DomainConsolidationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.consolidation = mapped
}
/**
* @param value The flag that enables the auto-merging of duplicate profiles.
*/
@JvmName("ayhptrlckgcfwcge")
public suspend fun enabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value A number between 0 and 1 that represents the minimum confidence score required for profiles within a matching group to be merged during the auto-merge process. A higher score means higher similarity required to merge profiles.
*/
@JvmName("tgtgoilystpimfac")
public suspend fun minAllowedConfidenceScoreForMerging(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minAllowedConfidenceScoreForMerging = mapped
}
internal fun build(): DomainAutoMergingArgs = DomainAutoMergingArgs(
conflictResolution = conflictResolution,
consolidation = consolidation,
enabled = enabled ?: throw PulumiNullFieldException("enabled"),
minAllowedConfidenceScoreForMerging = minAllowedConfidenceScoreForMerging,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy