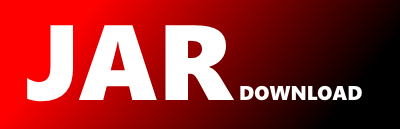
com.pulumi.awsnative.datasync.kotlin.inputs.TaskOptionsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.datasync.kotlin.inputs
import com.pulumi.awsnative.datasync.inputs.TaskOptionsArgs.builder
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsAtime
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsGid
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsLogLevel
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsMtime
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsObjectTags
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsOverwriteMode
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsPosixPermissions
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsPreserveDeletedFiles
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsPreserveDevices
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsSecurityDescriptorCopyFlags
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsTaskQueueing
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsTransferMode
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsUid
import com.pulumi.awsnative.datasync.kotlin.enums.TaskOptionsVerifyMode
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Represents the options that are available to control the behavior of a StartTaskExecution operation.
* @property atime A file metadata value that shows the last time a file was accessed (that is, when the file was read or written to).
* @property bytesPerSecond A value that limits the bandwidth used by AWS DataSync.
* @property gid The group ID (GID) of the file's owners.
* @property logLevel A value that determines the types of logs that DataSync publishes to a log stream in the Amazon CloudWatch log group that you provide.
* @property mtime A value that indicates the last time that a file was modified (that is, a file was written to) before the PREPARING phase.
* @property objectTags A value that determines whether object tags should be read from the source object store and written to the destination object store.
* @property overwriteMode A value that determines whether files at the destination should be overwritten or preserved when copying files.
* @property posixPermissions A value that determines which users or groups can access a file for a specific purpose such as reading, writing, or execution of the file.
* @property preserveDeletedFiles A value that specifies whether files in the destination that don't exist in the source file system should be preserved.
* @property preserveDevices A value that determines whether AWS DataSync should preserve the metadata of block and character devices in the source file system, and recreate the files with that device name and metadata on the destination.
* @property securityDescriptorCopyFlags A value that determines which components of the SMB security descriptor are copied during transfer.
* @property taskQueueing A value that determines whether tasks should be queued before executing the tasks.
* @property transferMode A value that determines whether DataSync transfers only the data and metadata that differ between the source and the destination location, or whether DataSync transfers all the content from the source, without comparing to the destination location.
* @property uid The user ID (UID) of the file's owner.
* @property verifyMode A value that determines whether a data integrity verification should be performed at the end of a task execution after all data and metadata have been transferred.
*/
public data class TaskOptionsArgs(
public val atime: Output? = null,
public val bytesPerSecond: Output? = null,
public val gid: Output? = null,
public val logLevel: Output? = null,
public val mtime: Output? = null,
public val objectTags: Output? = null,
public val overwriteMode: Output? = null,
public val posixPermissions: Output? = null,
public val preserveDeletedFiles: Output? = null,
public val preserveDevices: Output? = null,
public val securityDescriptorCopyFlags: Output? = null,
public val taskQueueing: Output? = null,
public val transferMode: Output? = null,
public val uid: Output? = null,
public val verifyMode: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.datasync.inputs.TaskOptionsArgs =
com.pulumi.awsnative.datasync.inputs.TaskOptionsArgs.builder()
.atime(atime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.bytesPerSecond(bytesPerSecond?.applyValue({ args0 -> args0 }))
.gid(gid?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.logLevel(logLevel?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.mtime(mtime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.objectTags(objectTags?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.overwriteMode(overwriteMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.posixPermissions(posixPermissions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.preserveDeletedFiles(
preserveDeletedFiles?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.preserveDevices(preserveDevices?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.securityDescriptorCopyFlags(
securityDescriptorCopyFlags?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.taskQueueing(taskQueueing?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.transferMode(transferMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.uid(uid?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.verifyMode(verifyMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TaskOptionsArgs].
*/
@PulumiTagMarker
public class TaskOptionsArgsBuilder internal constructor() {
private var atime: Output? = null
private var bytesPerSecond: Output? = null
private var gid: Output? = null
private var logLevel: Output? = null
private var mtime: Output? = null
private var objectTags: Output? = null
private var overwriteMode: Output? = null
private var posixPermissions: Output? = null
private var preserveDeletedFiles: Output? = null
private var preserveDevices: Output? = null
private var securityDescriptorCopyFlags: Output? = null
private var taskQueueing: Output? = null
private var transferMode: Output? = null
private var uid: Output? = null
private var verifyMode: Output? = null
/**
* @param value A file metadata value that shows the last time a file was accessed (that is, when the file was read or written to).
*/
@JvmName("fcshusienengdhtg")
public suspend fun atime(`value`: Output) {
this.atime = value
}
/**
* @param value A value that limits the bandwidth used by AWS DataSync.
*/
@JvmName("qgnfnhtclyqefmgj")
public suspend fun bytesPerSecond(`value`: Output) {
this.bytesPerSecond = value
}
/**
* @param value The group ID (GID) of the file's owners.
*/
@JvmName("vbmmxsxwlnwskfsj")
public suspend fun gid(`value`: Output) {
this.gid = value
}
/**
* @param value A value that determines the types of logs that DataSync publishes to a log stream in the Amazon CloudWatch log group that you provide.
*/
@JvmName("jsbnxhvhrcmlabvw")
public suspend fun logLevel(`value`: Output) {
this.logLevel = value
}
/**
* @param value A value that indicates the last time that a file was modified (that is, a file was written to) before the PREPARING phase.
*/
@JvmName("sayasixwpchiadnq")
public suspend fun mtime(`value`: Output) {
this.mtime = value
}
/**
* @param value A value that determines whether object tags should be read from the source object store and written to the destination object store.
*/
@JvmName("gabgvxmgiilqgumi")
public suspend fun objectTags(`value`: Output) {
this.objectTags = value
}
/**
* @param value A value that determines whether files at the destination should be overwritten or preserved when copying files.
*/
@JvmName("vlxvdmlftpequhxd")
public suspend fun overwriteMode(`value`: Output) {
this.overwriteMode = value
}
/**
* @param value A value that determines which users or groups can access a file for a specific purpose such as reading, writing, or execution of the file.
*/
@JvmName("dbcpsgqxjmvkwxsq")
public suspend fun posixPermissions(`value`: Output) {
this.posixPermissions = value
}
/**
* @param value A value that specifies whether files in the destination that don't exist in the source file system should be preserved.
*/
@JvmName("ybtyxqrwghctlrme")
public suspend fun preserveDeletedFiles(`value`: Output) {
this.preserveDeletedFiles = value
}
/**
* @param value A value that determines whether AWS DataSync should preserve the metadata of block and character devices in the source file system, and recreate the files with that device name and metadata on the destination.
*/
@JvmName("dcpkewbgcylfdqyx")
public suspend fun preserveDevices(`value`: Output) {
this.preserveDevices = value
}
/**
* @param value A value that determines which components of the SMB security descriptor are copied during transfer.
*/
@JvmName("klhwckhqrtwbcmpr")
public suspend fun securityDescriptorCopyFlags(`value`: Output) {
this.securityDescriptorCopyFlags = value
}
/**
* @param value A value that determines whether tasks should be queued before executing the tasks.
*/
@JvmName("adsyfnpruqlqqwvg")
public suspend fun taskQueueing(`value`: Output) {
this.taskQueueing = value
}
/**
* @param value A value that determines whether DataSync transfers only the data and metadata that differ between the source and the destination location, or whether DataSync transfers all the content from the source, without comparing to the destination location.
*/
@JvmName("gerorhuheoxmpyxm")
public suspend fun transferMode(`value`: Output) {
this.transferMode = value
}
/**
* @param value The user ID (UID) of the file's owner.
*/
@JvmName("prqldansvnnbucvs")
public suspend fun uid(`value`: Output) {
this.uid = value
}
/**
* @param value A value that determines whether a data integrity verification should be performed at the end of a task execution after all data and metadata have been transferred.
*/
@JvmName("ukteqxkrvwayveqw")
public suspend fun verifyMode(`value`: Output) {
this.verifyMode = value
}
/**
* @param value A file metadata value that shows the last time a file was accessed (that is, when the file was read or written to).
*/
@JvmName("cvhcxupbcmpwaeny")
public suspend fun atime(`value`: TaskOptionsAtime?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.atime = mapped
}
/**
* @param value A value that limits the bandwidth used by AWS DataSync.
*/
@JvmName("hyebtjtireqpmriv")
public suspend fun bytesPerSecond(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bytesPerSecond = mapped
}
/**
* @param value The group ID (GID) of the file's owners.
*/
@JvmName("hdtcgcdixpiapqlx")
public suspend fun gid(`value`: TaskOptionsGid?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gid = mapped
}
/**
* @param value A value that determines the types of logs that DataSync publishes to a log stream in the Amazon CloudWatch log group that you provide.
*/
@JvmName("bmlqgcknswbwownv")
public suspend fun logLevel(`value`: TaskOptionsLogLevel?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logLevel = mapped
}
/**
* @param value A value that indicates the last time that a file was modified (that is, a file was written to) before the PREPARING phase.
*/
@JvmName("sxfkfpoyhsqlnrmh")
public suspend fun mtime(`value`: TaskOptionsMtime?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mtime = mapped
}
/**
* @param value A value that determines whether object tags should be read from the source object store and written to the destination object store.
*/
@JvmName("vbmvukdhndptnqdf")
public suspend fun objectTags(`value`: TaskOptionsObjectTags?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.objectTags = mapped
}
/**
* @param value A value that determines whether files at the destination should be overwritten or preserved when copying files.
*/
@JvmName("cmphrnnuxqwrlpmi")
public suspend fun overwriteMode(`value`: TaskOptionsOverwriteMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.overwriteMode = mapped
}
/**
* @param value A value that determines which users or groups can access a file for a specific purpose such as reading, writing, or execution of the file.
*/
@JvmName("pqsbdmrdmsaagnan")
public suspend fun posixPermissions(`value`: TaskOptionsPosixPermissions?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.posixPermissions = mapped
}
/**
* @param value A value that specifies whether files in the destination that don't exist in the source file system should be preserved.
*/
@JvmName("himcbujuyhmiporh")
public suspend fun preserveDeletedFiles(`value`: TaskOptionsPreserveDeletedFiles?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preserveDeletedFiles = mapped
}
/**
* @param value A value that determines whether AWS DataSync should preserve the metadata of block and character devices in the source file system, and recreate the files with that device name and metadata on the destination.
*/
@JvmName("mxkokvinsxskeggo")
public suspend fun preserveDevices(`value`: TaskOptionsPreserveDevices?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preserveDevices = mapped
}
/**
* @param value A value that determines which components of the SMB security descriptor are copied during transfer.
*/
@JvmName("axekfhojtwepplwm")
public suspend fun securityDescriptorCopyFlags(`value`: TaskOptionsSecurityDescriptorCopyFlags?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securityDescriptorCopyFlags = mapped
}
/**
* @param value A value that determines whether tasks should be queued before executing the tasks.
*/
@JvmName("uvduqqugnlopvsaf")
public suspend fun taskQueueing(`value`: TaskOptionsTaskQueueing?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.taskQueueing = mapped
}
/**
* @param value A value that determines whether DataSync transfers only the data and metadata that differ between the source and the destination location, or whether DataSync transfers all the content from the source, without comparing to the destination location.
*/
@JvmName("jxhxnmpvpvsjbynr")
public suspend fun transferMode(`value`: TaskOptionsTransferMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transferMode = mapped
}
/**
* @param value The user ID (UID) of the file's owner.
*/
@JvmName("vbmopcxwuyfxfwuj")
public suspend fun uid(`value`: TaskOptionsUid?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uid = mapped
}
/**
* @param value A value that determines whether a data integrity verification should be performed at the end of a task execution after all data and metadata have been transferred.
*/
@JvmName("sxhamanxaqttocns")
public suspend fun verifyMode(`value`: TaskOptionsVerifyMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verifyMode = mapped
}
internal fun build(): TaskOptionsArgs = TaskOptionsArgs(
atime = atime,
bytesPerSecond = bytesPerSecond,
gid = gid,
logLevel = logLevel,
mtime = mtime,
objectTags = objectTags,
overwriteMode = overwriteMode,
posixPermissions = posixPermissions,
preserveDeletedFiles = preserveDeletedFiles,
preserveDevices = preserveDevices,
securityDescriptorCopyFlags = securityDescriptorCopyFlags,
taskQueueing = taskQueueing,
transferMode = transferMode,
uid = uid,
verifyMode = verifyMode,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy