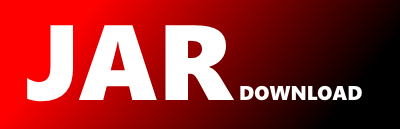
com.pulumi.awsnative.devicefarm.kotlin.DevicefarmFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.devicefarm.kotlin
import com.pulumi.awsnative.devicefarm.DevicefarmFunctions.getDevicePoolPlain
import com.pulumi.awsnative.devicefarm.DevicefarmFunctions.getInstanceProfilePlain
import com.pulumi.awsnative.devicefarm.DevicefarmFunctions.getNetworkProfilePlain
import com.pulumi.awsnative.devicefarm.DevicefarmFunctions.getProjectPlain
import com.pulumi.awsnative.devicefarm.DevicefarmFunctions.getTestGridProjectPlain
import com.pulumi.awsnative.devicefarm.DevicefarmFunctions.getVpceConfigurationPlain
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetDevicePoolPlainArgs
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetDevicePoolPlainArgsBuilder
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetInstanceProfilePlainArgs
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetInstanceProfilePlainArgsBuilder
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetNetworkProfilePlainArgs
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetNetworkProfilePlainArgsBuilder
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetProjectPlainArgs
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetProjectPlainArgsBuilder
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetTestGridProjectPlainArgs
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetTestGridProjectPlainArgsBuilder
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetVpceConfigurationPlainArgs
import com.pulumi.awsnative.devicefarm.kotlin.inputs.GetVpceConfigurationPlainArgsBuilder
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetDevicePoolResult
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetInstanceProfileResult
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetNetworkProfileResult
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetProjectResult
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetTestGridProjectResult
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetVpceConfigurationResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetDevicePoolResult.Companion.toKotlin as getDevicePoolResultToKotlin
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetInstanceProfileResult.Companion.toKotlin as getInstanceProfileResultToKotlin
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetNetworkProfileResult.Companion.toKotlin as getNetworkProfileResultToKotlin
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetProjectResult.Companion.toKotlin as getProjectResultToKotlin
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetTestGridProjectResult.Companion.toKotlin as getTestGridProjectResultToKotlin
import com.pulumi.awsnative.devicefarm.kotlin.outputs.GetVpceConfigurationResult.Companion.toKotlin as getVpceConfigurationResultToKotlin
public object DevicefarmFunctions {
/**
* AWS::DeviceFarm::DevicePool creates a new Device Pool for a given DF Project
* @param argument null
* @return null
*/
public suspend fun getDevicePool(argument: GetDevicePoolPlainArgs): GetDevicePoolResult =
getDevicePoolResultToKotlin(getDevicePoolPlain(argument.toJava()).await())
/**
* @see [getDevicePool].
* @param arn The Amazon Resource Name (ARN) of the device pool. See [Amazon resource names](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference guide* .
* @return null
*/
public suspend fun getDevicePool(arn: String): GetDevicePoolResult {
val argument = GetDevicePoolPlainArgs(
arn = arn,
)
return getDevicePoolResultToKotlin(getDevicePoolPlain(argument.toJava()).await())
}
/**
* @see [getDevicePool].
* @param argument Builder for [com.pulumi.awsnative.devicefarm.kotlin.inputs.GetDevicePoolPlainArgs].
* @return null
*/
public suspend fun getDevicePool(argument: suspend GetDevicePoolPlainArgsBuilder.() -> Unit): GetDevicePoolResult {
val builder = GetDevicePoolPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDevicePoolResultToKotlin(getDevicePoolPlain(builtArgument.toJava()).await())
}
/**
* AWS::DeviceFarm::InstanceProfile creates a new Device Farm Instance Profile
* @param argument null
* @return null
*/
public suspend fun getInstanceProfile(argument: GetInstanceProfilePlainArgs): GetInstanceProfileResult =
getInstanceProfileResultToKotlin(getInstanceProfilePlain(argument.toJava()).await())
/**
* @see [getInstanceProfile].
* @param arn The Amazon Resource Name (ARN) of the instance profile. See [Amazon resource names](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference guide* .
* @return null
*/
public suspend fun getInstanceProfile(arn: String): GetInstanceProfileResult {
val argument = GetInstanceProfilePlainArgs(
arn = arn,
)
return getInstanceProfileResultToKotlin(getInstanceProfilePlain(argument.toJava()).await())
}
/**
* @see [getInstanceProfile].
* @param argument Builder for [com.pulumi.awsnative.devicefarm.kotlin.inputs.GetInstanceProfilePlainArgs].
* @return null
*/
public suspend fun getInstanceProfile(argument: suspend GetInstanceProfilePlainArgsBuilder.() -> Unit): GetInstanceProfileResult {
val builder = GetInstanceProfilePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getInstanceProfileResultToKotlin(getInstanceProfilePlain(builtArgument.toJava()).await())
}
/**
* AWS::DeviceFarm::NetworkProfile creates a new DF Network Profile
* @param argument null
* @return null
*/
public suspend fun getNetworkProfile(argument: GetNetworkProfilePlainArgs): GetNetworkProfileResult =
getNetworkProfileResultToKotlin(getNetworkProfilePlain(argument.toJava()).await())
/**
* @see [getNetworkProfile].
* @param arn The Amazon Resource Name (ARN) of the network profile. See [Amazon resource names](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference guide* .
* @return null
*/
public suspend fun getNetworkProfile(arn: String): GetNetworkProfileResult {
val argument = GetNetworkProfilePlainArgs(
arn = arn,
)
return getNetworkProfileResultToKotlin(getNetworkProfilePlain(argument.toJava()).await())
}
/**
* @see [getNetworkProfile].
* @param argument Builder for [com.pulumi.awsnative.devicefarm.kotlin.inputs.GetNetworkProfilePlainArgs].
* @return null
*/
public suspend fun getNetworkProfile(argument: suspend GetNetworkProfilePlainArgsBuilder.() -> Unit): GetNetworkProfileResult {
val builder = GetNetworkProfilePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNetworkProfileResultToKotlin(getNetworkProfilePlain(builtArgument.toJava()).await())
}
/**
* AWS::DeviceFarm::Project creates a new Device Farm Project
* @param argument null
* @return null
*/
public suspend fun getProject(argument: GetProjectPlainArgs): GetProjectResult =
getProjectResultToKotlin(getProjectPlain(argument.toJava()).await())
/**
* @see [getProject].
* @param arn The Amazon Resource Name (ARN) of the project. See [Amazon resource names](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference guide* .
* @return null
*/
public suspend fun getProject(arn: String): GetProjectResult {
val argument = GetProjectPlainArgs(
arn = arn,
)
return getProjectResultToKotlin(getProjectPlain(argument.toJava()).await())
}
/**
* @see [getProject].
* @param argument Builder for [com.pulumi.awsnative.devicefarm.kotlin.inputs.GetProjectPlainArgs].
* @return null
*/
public suspend fun getProject(argument: suspend GetProjectPlainArgsBuilder.() -> Unit): GetProjectResult {
val builder = GetProjectPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProjectResultToKotlin(getProjectPlain(builtArgument.toJava()).await())
}
/**
* AWS::DeviceFarm::TestGridProject creates a new TestGrid Project
* @param argument null
* @return null
*/
public suspend fun getTestGridProject(argument: GetTestGridProjectPlainArgs): GetTestGridProjectResult =
getTestGridProjectResultToKotlin(getTestGridProjectPlain(argument.toJava()).await())
/**
* @see [getTestGridProject].
* @param arn The Amazon Resource Name (ARN) of the `TestGrid` project. See [Amazon resource names](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference guide* .
* @return null
*/
public suspend fun getTestGridProject(arn: String): GetTestGridProjectResult {
val argument = GetTestGridProjectPlainArgs(
arn = arn,
)
return getTestGridProjectResultToKotlin(getTestGridProjectPlain(argument.toJava()).await())
}
/**
* @see [getTestGridProject].
* @param argument Builder for [com.pulumi.awsnative.devicefarm.kotlin.inputs.GetTestGridProjectPlainArgs].
* @return null
*/
public suspend fun getTestGridProject(argument: suspend GetTestGridProjectPlainArgsBuilder.() -> Unit): GetTestGridProjectResult {
val builder = GetTestGridProjectPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTestGridProjectResultToKotlin(getTestGridProjectPlain(builtArgument.toJava()).await())
}
/**
* AWS::DeviceFarm::VPCEConfiguration creates a new Device Farm VPCE Configuration
* @param argument null
* @return null
*/
public suspend fun getVpceConfiguration(argument: GetVpceConfigurationPlainArgs): GetVpceConfigurationResult =
getVpceConfigurationResultToKotlin(getVpceConfigurationPlain(argument.toJava()).await())
/**
* @see [getVpceConfiguration].
* @param arn The Amazon Resource Name (ARN) of the VPC endpoint. See [Amazon resource names](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference guide* .
* @return null
*/
public suspend fun getVpceConfiguration(arn: String): GetVpceConfigurationResult {
val argument = GetVpceConfigurationPlainArgs(
arn = arn,
)
return getVpceConfigurationResultToKotlin(getVpceConfigurationPlain(argument.toJava()).await())
}
/**
* @see [getVpceConfiguration].
* @param argument Builder for [com.pulumi.awsnative.devicefarm.kotlin.inputs.GetVpceConfigurationPlainArgs].
* @return null
*/
public suspend fun getVpceConfiguration(argument: suspend GetVpceConfigurationPlainArgsBuilder.() -> Unit): GetVpceConfigurationResult {
val builder = GetVpceConfigurationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVpceConfigurationResultToKotlin(getVpceConfigurationPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy