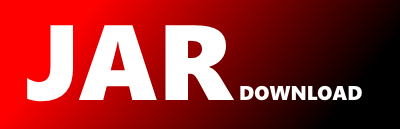
com.pulumi.awsnative.dms.kotlin.ReplicationConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.dms.kotlin
import com.pulumi.awsnative.dms.ReplicationConfigArgs.builder
import com.pulumi.awsnative.dms.kotlin.enums.ReplicationConfigReplicationType
import com.pulumi.awsnative.dms.kotlin.inputs.ReplicationConfigComputeConfigArgs
import com.pulumi.awsnative.dms.kotlin.inputs.ReplicationConfigComputeConfigArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A replication configuration that you later provide to configure and start a AWS DMS Serverless replication
* @property computeConfig Configuration parameters for provisioning an AWS DMS Serverless replication.
* @property replicationConfigIdentifier A unique identifier of replication configuration
* @property replicationSettings JSON settings for Servereless replications that are provisioned using this replication configuration
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::DMS::ReplicationConfig` for more information about the expected schema for this property.
* @property replicationType The type of AWS DMS Serverless replication to provision using this replication configuration
* @property resourceIdentifier A unique value or name that you get set for a given resource that can be used to construct an Amazon Resource Name (ARN) for that resource
* @property sourceEndpointArn The Amazon Resource Name (ARN) of the source endpoint for this AWS DMS Serverless replication configuration
* @property supplementalSettings JSON settings for specifying supplemental data
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::DMS::ReplicationConfig` for more information about the expected schema for this property.
* @property tableMappings JSON table mappings for AWS DMS Serverless replications that are provisioned using this replication configuration
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::DMS::ReplicationConfig` for more information about the expected schema for this property.
* @property tags Contains a map of the key-value pairs for the resource tag or tags assigned to the dataset.
* @property targetEndpointArn The Amazon Resource Name (ARN) of the target endpoint for this AWS DMS Serverless replication configuration
*/
public data class ReplicationConfigArgs(
public val computeConfig: Output? = null,
public val replicationConfigIdentifier: Output? = null,
public val replicationSettings: Output? = null,
public val replicationType: Output? = null,
public val resourceIdentifier: Output? = null,
public val sourceEndpointArn: Output? = null,
public val supplementalSettings: Output? = null,
public val tableMappings: Output? = null,
public val tags: Output>? = null,
public val targetEndpointArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.dms.ReplicationConfigArgs =
com.pulumi.awsnative.dms.ReplicationConfigArgs.builder()
.computeConfig(computeConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.replicationConfigIdentifier(replicationConfigIdentifier?.applyValue({ args0 -> args0 }))
.replicationSettings(replicationSettings?.applyValue({ args0 -> args0 }))
.replicationType(replicationType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceIdentifier(resourceIdentifier?.applyValue({ args0 -> args0 }))
.sourceEndpointArn(sourceEndpointArn?.applyValue({ args0 -> args0 }))
.supplementalSettings(supplementalSettings?.applyValue({ args0 -> args0 }))
.tableMappings(tableMappings?.applyValue({ args0 -> args0 }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.targetEndpointArn(targetEndpointArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ReplicationConfigArgs].
*/
@PulumiTagMarker
public class ReplicationConfigArgsBuilder internal constructor() {
private var computeConfig: Output? = null
private var replicationConfigIdentifier: Output? = null
private var replicationSettings: Output? = null
private var replicationType: Output? = null
private var resourceIdentifier: Output? = null
private var sourceEndpointArn: Output? = null
private var supplementalSettings: Output? = null
private var tableMappings: Output? = null
private var tags: Output>? = null
private var targetEndpointArn: Output? = null
/**
* @param value Configuration parameters for provisioning an AWS DMS Serverless replication.
*/
@JvmName("qlvsbbglvoovtypd")
public suspend fun computeConfig(`value`: Output) {
this.computeConfig = value
}
/**
* @param value A unique identifier of replication configuration
*/
@JvmName("poxexedratykvjtm")
public suspend fun replicationConfigIdentifier(`value`: Output) {
this.replicationConfigIdentifier = value
}
/**
* @param value JSON settings for Servereless replications that are provisioned using this replication configuration
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::DMS::ReplicationConfig` for more information about the expected schema for this property.
*/
@JvmName("kailbnybarvesxpy")
public suspend fun replicationSettings(`value`: Output) {
this.replicationSettings = value
}
/**
* @param value The type of AWS DMS Serverless replication to provision using this replication configuration
*/
@JvmName("ffsnthquteagxeey")
public suspend fun replicationType(`value`: Output) {
this.replicationType = value
}
/**
* @param value A unique value or name that you get set for a given resource that can be used to construct an Amazon Resource Name (ARN) for that resource
*/
@JvmName("bydbkhdesnjtvdfg")
public suspend fun resourceIdentifier(`value`: Output) {
this.resourceIdentifier = value
}
/**
* @param value The Amazon Resource Name (ARN) of the source endpoint for this AWS DMS Serverless replication configuration
*/
@JvmName("bbfbgleulfxroasr")
public suspend fun sourceEndpointArn(`value`: Output) {
this.sourceEndpointArn = value
}
/**
* @param value JSON settings for specifying supplemental data
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::DMS::ReplicationConfig` for more information about the expected schema for this property.
*/
@JvmName("qncogkcowjjegqdj")
public suspend fun supplementalSettings(`value`: Output) {
this.supplementalSettings = value
}
/**
* @param value JSON table mappings for AWS DMS Serverless replications that are provisioned using this replication configuration
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::DMS::ReplicationConfig` for more information about the expected schema for this property.
*/
@JvmName("hdqqtlcpclukfjco")
public suspend fun tableMappings(`value`: Output) {
this.tableMappings = value
}
/**
* @param value Contains a map of the key-value pairs for the resource tag or tags assigned to the dataset.
*/
@JvmName("nbnwpxonmltqkxsg")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("qsmopowsbxqksdde")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values Contains a map of the key-value pairs for the resource tag or tags assigned to the dataset.
*/
@JvmName("lbgovsrnmnumwdkh")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy