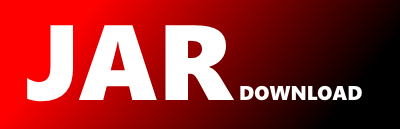
com.pulumi.awsnative.docdbelastic.kotlin.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.docdbelastic.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource = com.pulumi.awsnative.docdbelastic.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* The AWS::DocDBElastic::Cluster Amazon DocumentDB (with MongoDB compatibility) Elastic Scale resource describes a Cluster
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.awsnative.docdbelastic.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* The name of the Amazon DocumentDB elastic clusters administrator.
* *Constraints* :
* - Must be from 1 to 63 letters or numbers.
* - The first character must be a letter.
* - Cannot be a reserved word.
*/
public val adminUserName: Output
get() = javaResource.adminUserName().applyValue({ args0 -> args0 })
/**
* The password for the Elastic DocumentDB cluster administrator and can contain any printable ASCII characters.
* *Constraints* :
* - Must contain from 8 to 100 characters.
* - Cannot contain a forward slash (/), double quote ("), or the "at" symbol (@).
* - A valid `AdminUserName` entry is also required.
*/
public val adminUserPassword: Output?
get() = javaResource.adminUserPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The authentication type used to determine where to fetch the password used for accessing the elastic cluster. Valid types are `PLAIN_TEXT` or `SECRET_ARN` .
*/
public val authType: Output
get() = javaResource.authType().applyValue({ args0 -> args0 })
/**
* The number of days for which automatic snapshots are retained.
*/
public val backupRetentionPeriod: Output?
get() = javaResource.backupRetentionPeriod().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val clusterArn: Output
get() = javaResource.clusterArn().applyValue({ args0 -> args0 })
/**
* The URL used to connect to the elastic cluster.
*/
public val clusterEndpoint: Output
get() = javaResource.clusterEndpoint().applyValue({ args0 -> args0 })
/**
* The name of the new elastic cluster. This parameter is stored as a lowercase string.
* *Constraints* :
* - Must contain from 1 to 63 letters, numbers, or hyphens.
* - The first character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
* *Example* : `my-cluster`
*/
public val clusterName: Output
get() = javaResource.clusterName().applyValue({ args0 -> args0 })
/**
* The KMS key identifier to use to encrypt the new elastic cluster.
* The KMS key identifier is the Amazon Resource Name (ARN) for the KMS encryption key. If you are creating a cluster using the same Amazon account that owns this KMS encryption key, you can use the KMS key alias instead of the ARN as the KMS encryption key.
* If an encryption key is not specified, Amazon DocumentDB uses the default encryption key that KMS creates for your account. Your account has a different default encryption key for each Amazon Region.
*/
public val kmsKeyId: Output?
get() = javaResource.kmsKeyId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The daily time range during which automated backups are created if automated backups are enabled, as determined by `backupRetentionPeriod` .
*/
public val preferredBackupWindow: Output?
get() = javaResource.preferredBackupWindow().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
* *Format* : `ddd:hh24:mi-ddd:hh24:mi`
* *Default* : a 30-minute window selected at random from an 8-hour block of time for each AWS Region , occurring on a random day of the week.
* *Valid days* : Mon, Tue, Wed, Thu, Fri, Sat, Sun
* *Constraints* : Minimum 30-minute window.
*/
public val preferredMaintenanceWindow: Output?
get() = javaResource.preferredMaintenanceWindow().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The number of vCPUs assigned to each elastic cluster shard. Maximum is 64. Allowed values are 2, 4, 8, 16, 32, 64.
*/
public val shardCapacity: Output
get() = javaResource.shardCapacity().applyValue({ args0 -> args0 })
/**
* The number of shards assigned to the elastic cluster. Maximum is 32.
*/
public val shardCount: Output
get() = javaResource.shardCount().applyValue({ args0 -> args0 })
/**
* The number of replica instances applying to all shards in the cluster. A `shardInstanceCount` value of 1 means there is one writer instance, and any additional instances are replicas that can be used for reads and to improve availability.
*/
public val shardInstanceCount: Output?
get() = javaResource.shardInstanceCount().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon EC2 subnet IDs for the new elastic cluster.
*/
public val subnetIds: Output>?
get() = javaResource.subnetIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The tags to be assigned to the new elastic cluster.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
})
}).orElse(null)
})
/**
* A list of EC2 VPC security groups to associate with the new elastic cluster.
*/
public val vpcSecurityGroupIds: Output>?
get() = javaResource.vpcSecurityGroupIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
}
public object ClusterMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.docdbelastic.Cluster::class == javaResource::class
override fun map(javaResource: Resource): Cluster = Cluster(
javaResource as
com.pulumi.awsnative.docdbelastic.Cluster,
)
}
/**
* @see [Cluster].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Cluster].
*/
public suspend fun cluster(name: String, block: suspend ClusterResourceBuilder.() -> Unit): Cluster {
val builder = ClusterResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Cluster].
* @param name The _unique_ name of the resulting resource.
*/
public fun cluster(name: String): Cluster {
val builder = ClusterResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy