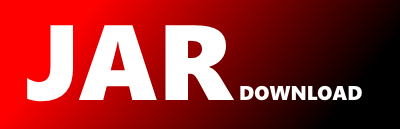
com.pulumi.awsnative.dynamodb.kotlin.inputs.TableStreamSpecificationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.dynamodb.kotlin.inputs
import com.pulumi.awsnative.dynamodb.inputs.TableStreamSpecificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Represents the DynamoDB Streams configuration for a table in DynamoDB.
* @property resourcePolicy Creates or updates a resource-based policy document that contains the permissions for DDB resources, such as a table's streams. Resource-based policies let you define access permissions by specifying who has access to each resource, and the actions they are allowed to perform on each resource.
* In a CFNshort template, you can provide the policy in JSON or YAML format because CFNshort converts YAML to JSON before submitting it to DDB. For more information about resource-based policies, see [Using resource-based policies for](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/access-control-resource-based.html) and [Resource-based policy examples](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/rbac-examples.html).
* @property streamViewType When an item in the table is modified, ``StreamViewType`` determines what information is written to the stream for this table. Valid values for ``StreamViewType`` are:
* + ``KEYS_ONLY`` - Only the key attributes of the modified item are written to the stream.
* + ``NEW_IMAGE`` - The entire item, as it appears after it was modified, is written to the stream.
* + ``OLD_IMAGE`` - The entire item, as it appeared before it was modified, is written to the stream.
* + ``NEW_AND_OLD_IMAGES`` - Both the new and the old item images of the item are written to the stream.
*/
public data class TableStreamSpecificationArgs(
public val resourcePolicy: Output? = null,
public val streamViewType: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.dynamodb.inputs.TableStreamSpecificationArgs =
com.pulumi.awsnative.dynamodb.inputs.TableStreamSpecificationArgs.builder()
.resourcePolicy(resourcePolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.streamViewType(streamViewType.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TableStreamSpecificationArgs].
*/
@PulumiTagMarker
public class TableStreamSpecificationArgsBuilder internal constructor() {
private var resourcePolicy: Output? = null
private var streamViewType: Output? = null
/**
* @param value Creates or updates a resource-based policy document that contains the permissions for DDB resources, such as a table's streams. Resource-based policies let you define access permissions by specifying who has access to each resource, and the actions they are allowed to perform on each resource.
* In a CFNshort template, you can provide the policy in JSON or YAML format because CFNshort converts YAML to JSON before submitting it to DDB. For more information about resource-based policies, see [Using resource-based policies for](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/access-control-resource-based.html) and [Resource-based policy examples](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/rbac-examples.html).
*/
@JvmName("sbjppfihgmfqbkkj")
public suspend fun resourcePolicy(`value`: Output) {
this.resourcePolicy = value
}
/**
* @param value When an item in the table is modified, ``StreamViewType`` determines what information is written to the stream for this table. Valid values for ``StreamViewType`` are:
* + ``KEYS_ONLY`` - Only the key attributes of the modified item are written to the stream.
* + ``NEW_IMAGE`` - The entire item, as it appears after it was modified, is written to the stream.
* + ``OLD_IMAGE`` - The entire item, as it appeared before it was modified, is written to the stream.
* + ``NEW_AND_OLD_IMAGES`` - Both the new and the old item images of the item are written to the stream.
*/
@JvmName("cjgpbhovomtkfbkc")
public suspend fun streamViewType(`value`: Output) {
this.streamViewType = value
}
/**
* @param value Creates or updates a resource-based policy document that contains the permissions for DDB resources, such as a table's streams. Resource-based policies let you define access permissions by specifying who has access to each resource, and the actions they are allowed to perform on each resource.
* In a CFNshort template, you can provide the policy in JSON or YAML format because CFNshort converts YAML to JSON before submitting it to DDB. For more information about resource-based policies, see [Using resource-based policies for](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/access-control-resource-based.html) and [Resource-based policy examples](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/rbac-examples.html).
*/
@JvmName("fppndbhehsaalxpe")
public suspend fun resourcePolicy(`value`: TableResourcePolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePolicy = mapped
}
/**
* @param argument Creates or updates a resource-based policy document that contains the permissions for DDB resources, such as a table's streams. Resource-based policies let you define access permissions by specifying who has access to each resource, and the actions they are allowed to perform on each resource.
* In a CFNshort template, you can provide the policy in JSON or YAML format because CFNshort converts YAML to JSON before submitting it to DDB. For more information about resource-based policies, see [Using resource-based policies for](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/access-control-resource-based.html) and [Resource-based policy examples](https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/rbac-examples.html).
*/
@JvmName("rkwjqbvljppkoidn")
public suspend fun resourcePolicy(argument: suspend TableResourcePolicyArgsBuilder.() -> Unit) {
val toBeMapped = TableResourcePolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.resourcePolicy = mapped
}
/**
* @param value When an item in the table is modified, ``StreamViewType`` determines what information is written to the stream for this table. Valid values for ``StreamViewType`` are:
* + ``KEYS_ONLY`` - Only the key attributes of the modified item are written to the stream.
* + ``NEW_IMAGE`` - The entire item, as it appears after it was modified, is written to the stream.
* + ``OLD_IMAGE`` - The entire item, as it appeared before it was modified, is written to the stream.
* + ``NEW_AND_OLD_IMAGES`` - Both the new and the old item images of the item are written to the stream.
*/
@JvmName("nufjbidpxqlstdfw")
public suspend fun streamViewType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.streamViewType = mapped
}
internal fun build(): TableStreamSpecificationArgs = TableStreamSpecificationArgs(
resourcePolicy = resourcePolicy,
streamViewType = streamViewType ?: throw PulumiNullFieldException("streamViewType"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy