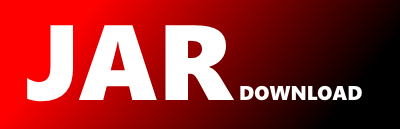
com.pulumi.awsnative.ec2.kotlin.inputs.InstanceNetworkInterfaceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ec2.kotlin.inputs
import com.pulumi.awsnative.ec2.inputs.InstanceNetworkInterfaceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property associateCarrierIpAddress Not currently supported by AWS CloudFormation.
* @property associatePublicIpAddress Indicates whether to assign a public IPv4 address to an instance you launch in a VPC.
* @property deleteOnTermination If set to true, the interface is deleted when the instance is terminated.
* @property description The description of the network interface.
* @property deviceIndex The position of the network interface in the attachment order. A primary network interface has a device index of 0.
* @property groupSet The IDs of the security groups for the network interface.
* @property ipv6AddressCount A number of IPv6 addresses to assign to the network interface.
* @property ipv6Addresses The IPv6 addresses associated with the network interface.
* @property networkInterfaceId The ID of the network interface.
* @property privateIpAddress The private IPv4 address of the network interface.
* @property privateIpAddresses One or more private IPv4 addresses to assign to the network interface.
* @property secondaryPrivateIpAddressCount The number of secondary private IPv4 addresses.
* @property subnetId The ID of the subnet.
*/
public data class InstanceNetworkInterfaceArgs(
public val associateCarrierIpAddress: Output? = null,
public val associatePublicIpAddress: Output? = null,
public val deleteOnTermination: Output? = null,
public val description: Output? = null,
public val deviceIndex: Output,
public val groupSet: Output>? = null,
public val ipv6AddressCount: Output? = null,
public val ipv6Addresses: Output>? = null,
public val networkInterfaceId: Output? = null,
public val privateIpAddress: Output? = null,
public val privateIpAddresses: Output>? = null,
public val secondaryPrivateIpAddressCount: Output? = null,
public val subnetId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ec2.inputs.InstanceNetworkInterfaceArgs =
com.pulumi.awsnative.ec2.inputs.InstanceNetworkInterfaceArgs.builder()
.associateCarrierIpAddress(associateCarrierIpAddress?.applyValue({ args0 -> args0 }))
.associatePublicIpAddress(associatePublicIpAddress?.applyValue({ args0 -> args0 }))
.deleteOnTermination(deleteOnTermination?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.deviceIndex(deviceIndex.applyValue({ args0 -> args0 }))
.groupSet(groupSet?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.ipv6AddressCount(ipv6AddressCount?.applyValue({ args0 -> args0 }))
.ipv6Addresses(
ipv6Addresses?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.networkInterfaceId(networkInterfaceId?.applyValue({ args0 -> args0 }))
.privateIpAddress(privateIpAddress?.applyValue({ args0 -> args0 }))
.privateIpAddresses(
privateIpAddresses?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.secondaryPrivateIpAddressCount(secondaryPrivateIpAddressCount?.applyValue({ args0 -> args0 }))
.subnetId(subnetId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceNetworkInterfaceArgs].
*/
@PulumiTagMarker
public class InstanceNetworkInterfaceArgsBuilder internal constructor() {
private var associateCarrierIpAddress: Output? = null
private var associatePublicIpAddress: Output? = null
private var deleteOnTermination: Output? = null
private var description: Output? = null
private var deviceIndex: Output? = null
private var groupSet: Output>? = null
private var ipv6AddressCount: Output? = null
private var ipv6Addresses: Output>? = null
private var networkInterfaceId: Output? = null
private var privateIpAddress: Output? = null
private var privateIpAddresses: Output>? = null
private var secondaryPrivateIpAddressCount: Output? = null
private var subnetId: Output? = null
/**
* @param value Not currently supported by AWS CloudFormation.
*/
@JvmName("oxadwrtkmlwgxmib")
public suspend fun associateCarrierIpAddress(`value`: Output) {
this.associateCarrierIpAddress = value
}
/**
* @param value Indicates whether to assign a public IPv4 address to an instance you launch in a VPC.
*/
@JvmName("jqgtdhruwiuyplwy")
public suspend fun associatePublicIpAddress(`value`: Output) {
this.associatePublicIpAddress = value
}
/**
* @param value If set to true, the interface is deleted when the instance is terminated.
*/
@JvmName("tpnraktxsjngiujg")
public suspend fun deleteOnTermination(`value`: Output) {
this.deleteOnTermination = value
}
/**
* @param value The description of the network interface.
*/
@JvmName("uedvqurbeehlpong")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The position of the network interface in the attachment order. A primary network interface has a device index of 0.
*/
@JvmName("xxmnwaaxxdltrxbo")
public suspend fun deviceIndex(`value`: Output) {
this.deviceIndex = value
}
/**
* @param value The IDs of the security groups for the network interface.
*/
@JvmName("xexbmnvvbeypwikd")
public suspend fun groupSet(`value`: Output>) {
this.groupSet = value
}
@JvmName("ofosnndrywvvrrvx")
public suspend fun groupSet(vararg values: Output) {
this.groupSet = Output.all(values.asList())
}
/**
* @param values The IDs of the security groups for the network interface.
*/
@JvmName("dyyrwbqrlygmkhpx")
public suspend fun groupSet(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy