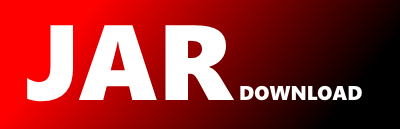
com.pulumi.awsnative.ecs.kotlin.CapacityProviderArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ecs.kotlin
import com.pulumi.awsnative.ecs.CapacityProviderArgs.builder
import com.pulumi.awsnative.ecs.kotlin.inputs.CapacityProviderAutoScalingGroupProviderArgs
import com.pulumi.awsnative.ecs.kotlin.inputs.CapacityProviderAutoScalingGroupProviderArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::ECS::CapacityProvider.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property autoScalingGroupProvider The Auto Scaling group settings for the capacity provider.
* @property name The name of the capacity provider. If a name is specified, it cannot start with `aws` , `ecs` , or `fargate` . If no name is specified, a default name in the `CFNStackName-CFNResourceName-RandomString` format is used.
* @property tags The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of a key and an optional value. You define both.
* The following basic restrictions apply to tags:
* - Maximum number of tags per resource - 50
* - For each resource, each tag key must be unique, and each tag key can have only one value.
* - Maximum key length - 128 Unicode characters in UTF-8
* - Maximum value length - 256 Unicode characters in UTF-8
* - If your tagging schema is used across multiple services and resources, remember that other services may have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable in UTF-8, and the following characters: + - = . _ : / @.
* - Tag keys and values are case-sensitive.
* - Do not use `aws:` , `AWS:` , or any upper or lowercase combination of such as a prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*/
public data class CapacityProviderArgs(
public val autoScalingGroupProvider: Output? = null,
public val name: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ecs.CapacityProviderArgs =
com.pulumi.awsnative.ecs.CapacityProviderArgs.builder()
.autoScalingGroupProvider(
autoScalingGroupProvider?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [CapacityProviderArgs].
*/
@PulumiTagMarker
public class CapacityProviderArgsBuilder internal constructor() {
private var autoScalingGroupProvider: Output? = null
private var name: Output? = null
private var tags: Output>? = null
/**
* @param value The Auto Scaling group settings for the capacity provider.
*/
@JvmName("aqepddppubtflyhp")
public suspend fun autoScalingGroupProvider(`value`: Output) {
this.autoScalingGroupProvider = value
}
/**
* @param value The name of the capacity provider. If a name is specified, it cannot start with `aws` , `ecs` , or `fargate` . If no name is specified, a default name in the `CFNStackName-CFNResourceName-RandomString` format is used.
*/
@JvmName("virmwisroltvgkin")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of a key and an optional value. You define both.
* The following basic restrictions apply to tags:
* - Maximum number of tags per resource - 50
* - For each resource, each tag key must be unique, and each tag key can have only one value.
* - Maximum key length - 128 Unicode characters in UTF-8
* - Maximum value length - 256 Unicode characters in UTF-8
* - If your tagging schema is used across multiple services and resources, remember that other services may have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable in UTF-8, and the following characters: + - = . _ : / @.
* - Tag keys and values are case-sensitive.
* - Do not use `aws:` , `AWS:` , or any upper or lowercase combination of such as a prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*/
@JvmName("bdqlcsfmubyvwdyv")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("naurctpufldecoxr")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of a key and an optional value. You define both.
* The following basic restrictions apply to tags:
* - Maximum number of tags per resource - 50
* - For each resource, each tag key must be unique, and each tag key can have only one value.
* - Maximum key length - 128 Unicode characters in UTF-8
* - Maximum value length - 256 Unicode characters in UTF-8
* - If your tagging schema is used across multiple services and resources, remember that other services may have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable in UTF-8, and the following characters: + - = . _ : / @.
* - Tag keys and values are case-sensitive.
* - Do not use `aws:` , `AWS:` , or any upper or lowercase combination of such as a prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*/
@JvmName("werfgmkiqgnfwfmi")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy