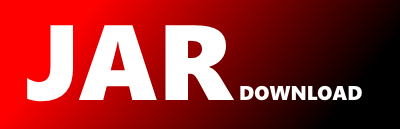
com.pulumi.awsnative.elasticache.kotlin.ElasticacheFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.elasticache.kotlin
import com.pulumi.awsnative.elasticache.ElasticacheFunctions.getGlobalReplicationGroupPlain
import com.pulumi.awsnative.elasticache.ElasticacheFunctions.getParameterGroupPlain
import com.pulumi.awsnative.elasticache.ElasticacheFunctions.getServerlessCachePlain
import com.pulumi.awsnative.elasticache.ElasticacheFunctions.getSubnetGroupPlain
import com.pulumi.awsnative.elasticache.ElasticacheFunctions.getUserGroupPlain
import com.pulumi.awsnative.elasticache.ElasticacheFunctions.getUserPlain
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetGlobalReplicationGroupPlainArgs
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetGlobalReplicationGroupPlainArgsBuilder
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetParameterGroupPlainArgs
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetParameterGroupPlainArgsBuilder
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetServerlessCachePlainArgs
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetServerlessCachePlainArgsBuilder
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetSubnetGroupPlainArgs
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetSubnetGroupPlainArgsBuilder
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetUserGroupPlainArgs
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetUserGroupPlainArgsBuilder
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetUserPlainArgs
import com.pulumi.awsnative.elasticache.kotlin.inputs.GetUserPlainArgsBuilder
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetGlobalReplicationGroupResult
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetParameterGroupResult
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetServerlessCacheResult
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetSubnetGroupResult
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetUserGroupResult
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetUserResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetGlobalReplicationGroupResult.Companion.toKotlin as getGlobalReplicationGroupResultToKotlin
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetParameterGroupResult.Companion.toKotlin as getParameterGroupResultToKotlin
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetServerlessCacheResult.Companion.toKotlin as getServerlessCacheResultToKotlin
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetSubnetGroupResult.Companion.toKotlin as getSubnetGroupResultToKotlin
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetUserGroupResult.Companion.toKotlin as getUserGroupResultToKotlin
import com.pulumi.awsnative.elasticache.kotlin.outputs.GetUserResult.Companion.toKotlin as getUserResultToKotlin
public object ElasticacheFunctions {
/**
* The AWS::ElastiCache::GlobalReplicationGroup resource creates an Amazon ElastiCache Global Replication Group.
* @param argument null
* @return null
*/
public suspend fun getGlobalReplicationGroup(argument: GetGlobalReplicationGroupPlainArgs): GetGlobalReplicationGroupResult =
getGlobalReplicationGroupResultToKotlin(getGlobalReplicationGroupPlain(argument.toJava()).await())
/**
* @see [getGlobalReplicationGroup].
* @param globalReplicationGroupId The name of the Global Datastore, it is generated by ElastiCache adding a prefix to GlobalReplicationGroupIdSuffix.
* @return null
*/
public suspend fun getGlobalReplicationGroup(globalReplicationGroupId: String): GetGlobalReplicationGroupResult {
val argument = GetGlobalReplicationGroupPlainArgs(
globalReplicationGroupId = globalReplicationGroupId,
)
return getGlobalReplicationGroupResultToKotlin(getGlobalReplicationGroupPlain(argument.toJava()).await())
}
/**
* @see [getGlobalReplicationGroup].
* @param argument Builder for [com.pulumi.awsnative.elasticache.kotlin.inputs.GetGlobalReplicationGroupPlainArgs].
* @return null
*/
public suspend fun getGlobalReplicationGroup(argument: suspend GetGlobalReplicationGroupPlainArgsBuilder.() -> Unit): GetGlobalReplicationGroupResult {
val builder = GetGlobalReplicationGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGlobalReplicationGroupResultToKotlin(getGlobalReplicationGroupPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::ElastiCache::ParameterGroup
* @param argument null
* @return null
*/
public suspend fun getParameterGroup(argument: GetParameterGroupPlainArgs): GetParameterGroupResult =
getParameterGroupResultToKotlin(getParameterGroupPlain(argument.toJava()).await())
/**
* @see [getParameterGroup].
* @param cacheParameterGroupName The name of the Cache Parameter Group.
* @return null
*/
public suspend fun getParameterGroup(cacheParameterGroupName: String): GetParameterGroupResult {
val argument = GetParameterGroupPlainArgs(
cacheParameterGroupName = cacheParameterGroupName,
)
return getParameterGroupResultToKotlin(getParameterGroupPlain(argument.toJava()).await())
}
/**
* @see [getParameterGroup].
* @param argument Builder for [com.pulumi.awsnative.elasticache.kotlin.inputs.GetParameterGroupPlainArgs].
* @return null
*/
public suspend fun getParameterGroup(argument: suspend GetParameterGroupPlainArgsBuilder.() -> Unit): GetParameterGroupResult {
val builder = GetParameterGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getParameterGroupResultToKotlin(getParameterGroupPlain(builtArgument.toJava()).await())
}
/**
* The AWS::ElastiCache::ServerlessCache resource creates an Amazon ElastiCache Serverless Cache.
* @param argument null
* @return null
*/
public suspend fun getServerlessCache(argument: GetServerlessCachePlainArgs): GetServerlessCacheResult =
getServerlessCacheResultToKotlin(getServerlessCachePlain(argument.toJava()).await())
/**
* @see [getServerlessCache].
* @param serverlessCacheName The name of the Serverless Cache. This value must be unique.
* @return null
*/
public suspend fun getServerlessCache(serverlessCacheName: String): GetServerlessCacheResult {
val argument = GetServerlessCachePlainArgs(
serverlessCacheName = serverlessCacheName,
)
return getServerlessCacheResultToKotlin(getServerlessCachePlain(argument.toJava()).await())
}
/**
* @see [getServerlessCache].
* @param argument Builder for [com.pulumi.awsnative.elasticache.kotlin.inputs.GetServerlessCachePlainArgs].
* @return null
*/
public suspend fun getServerlessCache(argument: suspend GetServerlessCachePlainArgsBuilder.() -> Unit): GetServerlessCacheResult {
val builder = GetServerlessCachePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServerlessCacheResultToKotlin(getServerlessCachePlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::ElastiCache::SubnetGroup
* @param argument null
* @return null
*/
public suspend fun getSubnetGroup(argument: GetSubnetGroupPlainArgs): GetSubnetGroupResult =
getSubnetGroupResultToKotlin(getSubnetGroupPlain(argument.toJava()).await())
/**
* @see [getSubnetGroup].
* @param cacheSubnetGroupName The name for the cache subnet group. This value is stored as a lowercase string.
* @return null
*/
public suspend fun getSubnetGroup(cacheSubnetGroupName: String): GetSubnetGroupResult {
val argument = GetSubnetGroupPlainArgs(
cacheSubnetGroupName = cacheSubnetGroupName,
)
return getSubnetGroupResultToKotlin(getSubnetGroupPlain(argument.toJava()).await())
}
/**
* @see [getSubnetGroup].
* @param argument Builder for [com.pulumi.awsnative.elasticache.kotlin.inputs.GetSubnetGroupPlainArgs].
* @return null
*/
public suspend fun getSubnetGroup(argument: suspend GetSubnetGroupPlainArgsBuilder.() -> Unit): GetSubnetGroupResult {
val builder = GetSubnetGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSubnetGroupResultToKotlin(getSubnetGroupPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::ElastiCache::User
* @param argument null
* @return null
*/
public suspend fun getUser(argument: GetUserPlainArgs): GetUserResult =
getUserResultToKotlin(getUserPlain(argument.toJava()).await())
/**
* @see [getUser].
* @param userId The ID of the user.
* @return null
*/
public suspend fun getUser(userId: String): GetUserResult {
val argument = GetUserPlainArgs(
userId = userId,
)
return getUserResultToKotlin(getUserPlain(argument.toJava()).await())
}
/**
* @see [getUser].
* @param argument Builder for [com.pulumi.awsnative.elasticache.kotlin.inputs.GetUserPlainArgs].
* @return null
*/
public suspend fun getUser(argument: suspend GetUserPlainArgsBuilder.() -> Unit): GetUserResult {
val builder = GetUserPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getUserResultToKotlin(getUserPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::ElastiCache::UserGroup
* @param argument null
* @return null
*/
public suspend fun getUserGroup(argument: GetUserGroupPlainArgs): GetUserGroupResult =
getUserGroupResultToKotlin(getUserGroupPlain(argument.toJava()).await())
/**
* @see [getUserGroup].
* @param userGroupId The ID of the user group.
* @return null
*/
public suspend fun getUserGroup(userGroupId: String): GetUserGroupResult {
val argument = GetUserGroupPlainArgs(
userGroupId = userGroupId,
)
return getUserGroupResultToKotlin(getUserGroupPlain(argument.toJava()).await())
}
/**
* @see [getUserGroup].
* @param argument Builder for [com.pulumi.awsnative.elasticache.kotlin.inputs.GetUserGroupPlainArgs].
* @return null
*/
public suspend fun getUserGroup(argument: suspend GetUserGroupPlainArgsBuilder.() -> Unit): GetUserGroupResult {
val builder = GetUserGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getUserGroupResultToKotlin(getUserGroupPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy