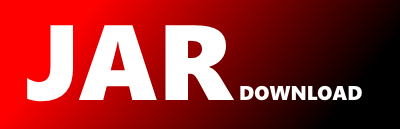
com.pulumi.awsnative.evidently.kotlin.LaunchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.evidently.kotlin
import com.pulumi.awsnative.evidently.LaunchArgs.builder
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchExecutionStatusObjectArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchExecutionStatusObjectArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchGroupObjectArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchGroupObjectArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchMetricDefinitionObjectArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchMetricDefinitionObjectArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchStepConfigArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.LaunchStepConfigArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Evidently::Launch.
* @property description An optional description for the launch.
* @property executionStatus Start or Stop Launch Launch. Default is not started.
* @property groups An array of structures that contains the feature and variations that are to be used for the launch. You can up to five launch groups in a launch.
* @property metricMonitors An array of structures that define the metrics that will be used to monitor the launch performance. You can have up to three metric monitors in the array.
* @property name The name for the launch. It can include up to 127 characters.
* @property project The name or ARN of the project that you want to create the launch in.
* @property randomizationSalt When Evidently assigns a particular user session to a launch, it must use a randomization ID to determine which variation the user session is served. This randomization ID is a combination of the entity ID and `randomizationSalt` . If you omit `randomizationSalt` , Evidently uses the launch name as the `randomizationsSalt` .
* @property scheduledSplitsConfig An array of structures that define the traffic allocation percentages among the feature variations during each step of the launch.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class LaunchArgs(
public val description: Output? = null,
public val executionStatus: Output? = null,
public val groups: Output>? = null,
public val metricMonitors: Output>? = null,
public val name: Output? = null,
public val project: Output? = null,
public val randomizationSalt: Output? = null,
public val scheduledSplitsConfig: Output>? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.evidently.LaunchArgs =
com.pulumi.awsnative.evidently.LaunchArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.executionStatus(executionStatus?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.groups(groups?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.metricMonitors(
metricMonitors?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.randomizationSalt(randomizationSalt?.applyValue({ args0 -> args0 }))
.scheduledSplitsConfig(
scheduledSplitsConfig?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [LaunchArgs].
*/
@PulumiTagMarker
public class LaunchArgsBuilder internal constructor() {
private var description: Output? = null
private var executionStatus: Output? = null
private var groups: Output>? = null
private var metricMonitors: Output>? = null
private var name: Output? = null
private var project: Output? = null
private var randomizationSalt: Output? = null
private var scheduledSplitsConfig: Output>? = null
private var tags: Output>? = null
/**
* @param value An optional description for the launch.
*/
@JvmName("undygllgbegmateu")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Start or Stop Launch Launch. Default is not started.
*/
@JvmName("lguvrbybmwodxvll")
public suspend fun executionStatus(`value`: Output) {
this.executionStatus = value
}
/**
* @param value An array of structures that contains the feature and variations that are to be used for the launch. You can up to five launch groups in a launch.
*/
@JvmName("whclstyesolqjotr")
public suspend fun groups(`value`: Output>) {
this.groups = value
}
@JvmName("axquewecwppwjkef")
public suspend fun groups(vararg values: Output) {
this.groups = Output.all(values.asList())
}
/**
* @param values An array of structures that contains the feature and variations that are to be used for the launch. You can up to five launch groups in a launch.
*/
@JvmName("ncnhknvlbldwiono")
public suspend fun groups(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy