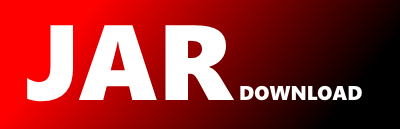
com.pulumi.awsnative.evidently.kotlin.ProjectArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.evidently.kotlin
import com.pulumi.awsnative.evidently.ProjectArgs.builder
import com.pulumi.awsnative.evidently.kotlin.inputs.ProjectAppConfigResourceObjectArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.ProjectAppConfigResourceObjectArgsBuilder
import com.pulumi.awsnative.evidently.kotlin.inputs.ProjectDataDeliveryObjectArgs
import com.pulumi.awsnative.evidently.kotlin.inputs.ProjectDataDeliveryObjectArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Evidently::Project
* @property appConfigResource Use this parameter if the project will use *client-side evaluation powered by AWS AppConfig* . Client-side evaluation allows your application to assign variations to user sessions locally instead of by calling the [EvaluateFeature](https://docs.aws.amazon.com/cloudwatchevidently/latest/APIReference/API_EvaluateFeature.html) operation. This mitigates the latency and availability risks that come with an API call. For more information, see [Use client-side evaluation - powered by AWS AppConfig .](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-Evidently-client-side-evaluation.html)
* This parameter is a structure that contains information about the AWS AppConfig application that will be used as for client-side evaluation.
* To create a project that uses client-side evaluation, you must have the `evidently:ExportProjectAsConfiguration` permission.
* @property dataDelivery A structure that contains information about where Evidently is to store evaluation events for longer term storage, if you choose to do so. If you choose not to store these events, Evidently deletes them after using them to produce metrics and other experiment results that you can view.
* You can't specify both `CloudWatchLogs` and `S3Destination` in the same operation.
* @property description An optional description of the project.
* @property name The name for the project. It can include up to 127 characters.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class ProjectArgs(
public val appConfigResource: Output? = null,
public val dataDelivery: Output? = null,
public val description: Output? = null,
public val name: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.evidently.ProjectArgs =
com.pulumi.awsnative.evidently.ProjectArgs.builder()
.appConfigResource(appConfigResource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dataDelivery(dataDelivery?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ProjectArgs].
*/
@PulumiTagMarker
public class ProjectArgsBuilder internal constructor() {
private var appConfigResource: Output? = null
private var dataDelivery: Output? = null
private var description: Output? = null
private var name: Output? = null
private var tags: Output>? = null
/**
* @param value Use this parameter if the project will use *client-side evaluation powered by AWS AppConfig* . Client-side evaluation allows your application to assign variations to user sessions locally instead of by calling the [EvaluateFeature](https://docs.aws.amazon.com/cloudwatchevidently/latest/APIReference/API_EvaluateFeature.html) operation. This mitigates the latency and availability risks that come with an API call. For more information, see [Use client-side evaluation - powered by AWS AppConfig .](https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/CloudWatch-Evidently-client-side-evaluation.html)
* This parameter is a structure that contains information about the AWS AppConfig application that will be used as for client-side evaluation.
* To create a project that uses client-side evaluation, you must have the `evidently:ExportProjectAsConfiguration` permission.
*/
@JvmName("lfntrtmpmwmtesmf")
public suspend fun appConfigResource(`value`: Output) {
this.appConfigResource = value
}
/**
* @param value A structure that contains information about where Evidently is to store evaluation events for longer term storage, if you choose to do so. If you choose not to store these events, Evidently deletes them after using them to produce metrics and other experiment results that you can view.
* You can't specify both `CloudWatchLogs` and `S3Destination` in the same operation.
*/
@JvmName("klqnqtotsbnpauvr")
public suspend fun dataDelivery(`value`: Output) {
this.dataDelivery = value
}
/**
* @param value An optional description of the project.
*/
@JvmName("kqfcvrxelgukfepm")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name for the project. It can include up to 127 characters.
*/
@JvmName("oraexhclqsqcuhtw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value An array of key-value pairs to apply to this resource.
*/
@JvmName("rysldkolbuobwgnx")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("acjsrhtpywdukyrs")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values An array of key-value pairs to apply to this resource.
*/
@JvmName("dmmogegsievlhobn")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy