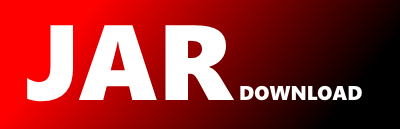
com.pulumi.awsnative.fms.kotlin.inputs.PolicyNetworkAclEntryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.fms.kotlin.inputs
import com.pulumi.awsnative.fms.inputs.PolicyNetworkAclEntryArgs.builder
import com.pulumi.awsnative.fms.kotlin.enums.PolicyNetworkAclEntryRuleAction
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Network ACL entry.
* @property cidrBlock CIDR block.
* @property egress Whether the entry is an egress entry.
* @property icmpTypeCode ICMP type and code.
* @property ipv6CidrBlock IPv6 CIDR block.
* @property portRange Port range.
* @property protocol Protocol.
* @property ruleAction Rule Action.
*/
public data class PolicyNetworkAclEntryArgs(
public val cidrBlock: Output? = null,
public val egress: Output,
public val icmpTypeCode: Output? = null,
public val ipv6CidrBlock: Output? = null,
public val portRange: Output? = null,
public val protocol: Output,
public val ruleAction: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.fms.inputs.PolicyNetworkAclEntryArgs =
com.pulumi.awsnative.fms.inputs.PolicyNetworkAclEntryArgs.builder()
.cidrBlock(cidrBlock?.applyValue({ args0 -> args0 }))
.egress(egress.applyValue({ args0 -> args0 }))
.icmpTypeCode(icmpTypeCode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ipv6CidrBlock(ipv6CidrBlock?.applyValue({ args0 -> args0 }))
.portRange(portRange?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.protocol(protocol.applyValue({ args0 -> args0 }))
.ruleAction(ruleAction.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PolicyNetworkAclEntryArgs].
*/
@PulumiTagMarker
public class PolicyNetworkAclEntryArgsBuilder internal constructor() {
private var cidrBlock: Output? = null
private var egress: Output? = null
private var icmpTypeCode: Output? = null
private var ipv6CidrBlock: Output? = null
private var portRange: Output? = null
private var protocol: Output? = null
private var ruleAction: Output? = null
/**
* @param value CIDR block.
*/
@JvmName("rxnavsfirdxusenx")
public suspend fun cidrBlock(`value`: Output) {
this.cidrBlock = value
}
/**
* @param value Whether the entry is an egress entry.
*/
@JvmName("wkwvwnwjsxohqupb")
public suspend fun egress(`value`: Output) {
this.egress = value
}
/**
* @param value ICMP type and code.
*/
@JvmName("qfeqkbkxuihthybw")
public suspend fun icmpTypeCode(`value`: Output) {
this.icmpTypeCode = value
}
/**
* @param value IPv6 CIDR block.
*/
@JvmName("urubksvyvkqjrbpg")
public suspend fun ipv6CidrBlock(`value`: Output) {
this.ipv6CidrBlock = value
}
/**
* @param value Port range.
*/
@JvmName("pvbvphfiakahavis")
public suspend fun portRange(`value`: Output) {
this.portRange = value
}
/**
* @param value Protocol.
*/
@JvmName("eukpnjqgmdigaytc")
public suspend fun protocol(`value`: Output) {
this.protocol = value
}
/**
* @param value Rule Action.
*/
@JvmName("kmihbmifcixxgerm")
public suspend fun ruleAction(`value`: Output) {
this.ruleAction = value
}
/**
* @param value CIDR block.
*/
@JvmName("lgdsthvomgypbrdu")
public suspend fun cidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cidrBlock = mapped
}
/**
* @param value Whether the entry is an egress entry.
*/
@JvmName("lingmyjpwoxkdcct")
public suspend fun egress(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.egress = mapped
}
/**
* @param value ICMP type and code.
*/
@JvmName("hymdnqgbmajktxps")
public suspend fun icmpTypeCode(`value`: PolicyNetworkAclEntryIcmpTypeCodePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.icmpTypeCode = mapped
}
/**
* @param argument ICMP type and code.
*/
@JvmName("lffefpfyvsfdrnie")
public suspend fun icmpTypeCode(argument: suspend PolicyNetworkAclEntryIcmpTypeCodePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = PolicyNetworkAclEntryIcmpTypeCodePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.icmpTypeCode = mapped
}
/**
* @param value IPv6 CIDR block.
*/
@JvmName("lidjkrqmtcvbctyt")
public suspend fun ipv6CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipv6CidrBlock = mapped
}
/**
* @param value Port range.
*/
@JvmName("vshryhqdsmvblsfx")
public suspend fun portRange(`value`: PolicyNetworkAclEntryPortRangePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.portRange = mapped
}
/**
* @param argument Port range.
*/
@JvmName("arjyqsepbbqxhlyy")
public suspend fun portRange(argument: suspend PolicyNetworkAclEntryPortRangePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = PolicyNetworkAclEntryPortRangePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.portRange = mapped
}
/**
* @param value Protocol.
*/
@JvmName("hhsfddwpuvkfnqtj")
public suspend fun protocol(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value Rule Action.
*/
@JvmName("drdtckkoccvylfql")
public suspend fun ruleAction(`value`: PolicyNetworkAclEntryRuleAction) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ruleAction = mapped
}
internal fun build(): PolicyNetworkAclEntryArgs = PolicyNetworkAclEntryArgs(
cidrBlock = cidrBlock,
egress = egress ?: throw PulumiNullFieldException("egress"),
icmpTypeCode = icmpTypeCode,
ipv6CidrBlock = ipv6CidrBlock,
portRange = portRange,
protocol = protocol ?: throw PulumiNullFieldException("protocol"),
ruleAction = ruleAction ?: throw PulumiNullFieldException("ruleAction"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy