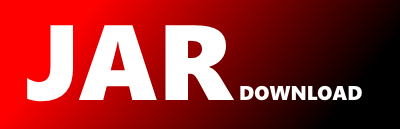
com.pulumi.awsnative.gamelift.kotlin.MatchmakingConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.gamelift.kotlin
import com.pulumi.awsnative.gamelift.MatchmakingConfigurationArgs.builder
import com.pulumi.awsnative.gamelift.kotlin.enums.MatchmakingConfigurationBackfillMode
import com.pulumi.awsnative.gamelift.kotlin.enums.MatchmakingConfigurationFlexMatchMode
import com.pulumi.awsnative.gamelift.kotlin.inputs.MatchmakingConfigurationGamePropertyArgs
import com.pulumi.awsnative.gamelift.kotlin.inputs.MatchmakingConfigurationGamePropertyArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The AWS::GameLift::MatchmakingConfiguration resource creates an Amazon GameLift (GameLift) matchmaking configuration.
* @property acceptanceRequired A flag that indicates whether a match that was created with this configuration must be accepted by the matched players
* @property acceptanceTimeoutSeconds The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required.
* @property additionalPlayerCount The number of player slots in a match to keep open for future players.
* @property backfillMode The method used to backfill game sessions created with this matchmaking configuration.
* @property creationTime A time stamp indicating when this data object was created.
* @property customEventData Information to attach to all events related to the matchmaking configuration.
* @property description A descriptive label that is associated with matchmaking configuration.
* @property flexMatchMode Indicates whether this matchmaking configuration is being used with Amazon GameLift hosting or as a standalone matchmaking solution.
* @property gameProperties A set of custom properties for a game session, formatted as key:value pairs.
* @property gameSessionData A set of custom game session properties, formatted as a single string value.
* @property gameSessionQueueArns The Amazon Resource Name (ARN) that is assigned to a Amazon GameLift game session queue resource and uniquely identifies it.
* @property name A unique identifier for the matchmaking configuration.
* @property notificationTarget An SNS topic ARN that is set up to receive matchmaking notifications.
* @property requestTimeoutSeconds The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out.
* @property ruleSetArn The Amazon Resource Name (ARN) associated with the GameLift matchmaking rule set resource that this configuration uses.
* @property ruleSetName A unique identifier for the matchmaking rule set to use with this configuration.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class MatchmakingConfigurationArgs(
public val acceptanceRequired: Output? = null,
public val acceptanceTimeoutSeconds: Output? = null,
public val additionalPlayerCount: Output? = null,
public val backfillMode: Output? = null,
public val creationTime: Output? = null,
public val customEventData: Output? = null,
public val description: Output? = null,
public val flexMatchMode: Output? = null,
public val gameProperties: Output>? = null,
public val gameSessionData: Output? = null,
public val gameSessionQueueArns: Output>? = null,
public val name: Output? = null,
public val notificationTarget: Output? = null,
public val requestTimeoutSeconds: Output? = null,
public val ruleSetArn: Output? = null,
public val ruleSetName: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.gamelift.MatchmakingConfigurationArgs =
com.pulumi.awsnative.gamelift.MatchmakingConfigurationArgs.builder()
.acceptanceRequired(acceptanceRequired?.applyValue({ args0 -> args0 }))
.acceptanceTimeoutSeconds(acceptanceTimeoutSeconds?.applyValue({ args0 -> args0 }))
.additionalPlayerCount(additionalPlayerCount?.applyValue({ args0 -> args0 }))
.backfillMode(backfillMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.creationTime(creationTime?.applyValue({ args0 -> args0 }))
.customEventData(customEventData?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.flexMatchMode(flexMatchMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.gameProperties(
gameProperties?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.gameSessionData(gameSessionData?.applyValue({ args0 -> args0 }))
.gameSessionQueueArns(gameSessionQueueArns?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name?.applyValue({ args0 -> args0 }))
.notificationTarget(notificationTarget?.applyValue({ args0 -> args0 }))
.requestTimeoutSeconds(requestTimeoutSeconds?.applyValue({ args0 -> args0 }))
.ruleSetArn(ruleSetArn?.applyValue({ args0 -> args0 }))
.ruleSetName(ruleSetName?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [MatchmakingConfigurationArgs].
*/
@PulumiTagMarker
public class MatchmakingConfigurationArgsBuilder internal constructor() {
private var acceptanceRequired: Output? = null
private var acceptanceTimeoutSeconds: Output? = null
private var additionalPlayerCount: Output? = null
private var backfillMode: Output? = null
private var creationTime: Output? = null
private var customEventData: Output? = null
private var description: Output? = null
private var flexMatchMode: Output? = null
private var gameProperties: Output>? = null
private var gameSessionData: Output? = null
private var gameSessionQueueArns: Output>? = null
private var name: Output? = null
private var notificationTarget: Output? = null
private var requestTimeoutSeconds: Output? = null
private var ruleSetArn: Output? = null
private var ruleSetName: Output? = null
private var tags: Output>? = null
/**
* @param value A flag that indicates whether a match that was created with this configuration must be accepted by the matched players
*/
@JvmName("bihdwchmoxvavgkj")
public suspend fun acceptanceRequired(`value`: Output) {
this.acceptanceRequired = value
}
/**
* @param value The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required.
*/
@JvmName("sggxticdophwwouh")
public suspend fun acceptanceTimeoutSeconds(`value`: Output) {
this.acceptanceTimeoutSeconds = value
}
/**
* @param value The number of player slots in a match to keep open for future players.
*/
@JvmName("xlqrclnaarfilspt")
public suspend fun additionalPlayerCount(`value`: Output) {
this.additionalPlayerCount = value
}
/**
* @param value The method used to backfill game sessions created with this matchmaking configuration.
*/
@JvmName("hhdgsyeyxwpltskt")
public suspend fun backfillMode(`value`: Output) {
this.backfillMode = value
}
/**
* @param value A time stamp indicating when this data object was created.
*/
@JvmName("nikepbnqaycyvxbu")
public suspend fun creationTime(`value`: Output) {
this.creationTime = value
}
/**
* @param value Information to attach to all events related to the matchmaking configuration.
*/
@JvmName("esekwnoarxcmqqaa")
public suspend fun customEventData(`value`: Output) {
this.customEventData = value
}
/**
* @param value A descriptive label that is associated with matchmaking configuration.
*/
@JvmName("dhatuiehypafroal")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Indicates whether this matchmaking configuration is being used with Amazon GameLift hosting or as a standalone matchmaking solution.
*/
@JvmName("kvwqqsswfvbsrcli")
public suspend fun flexMatchMode(`value`: Output) {
this.flexMatchMode = value
}
/**
* @param value A set of custom properties for a game session, formatted as key:value pairs.
*/
@JvmName("jcuivoattoqhfyrm")
public suspend fun gameProperties(`value`: Output>) {
this.gameProperties = value
}
@JvmName("xihhilcsbrkmnoup")
public suspend fun gameProperties(vararg values: Output) {
this.gameProperties = Output.all(values.asList())
}
/**
* @param values A set of custom properties for a game session, formatted as key:value pairs.
*/
@JvmName("ukqswbafohvotlvr")
public suspend fun gameProperties(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy