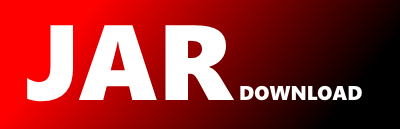
com.pulumi.awsnative.greengrassv2.kotlin.inputs.DeploymentIoTJobConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.greengrassv2.kotlin.inputs
import com.pulumi.awsnative.greengrassv2.inputs.DeploymentIoTJobConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property abortConfig The stop configuration for the job. This configuration defines when and how to stop a job rollout.
* @property jobExecutionsRolloutConfig The rollout configuration for the job. This configuration defines the rate at which the job rolls out to the fleet of target devices.
* @property timeoutConfig The timeout configuration for the job. This configuration defines the amount of time each device has to complete the job.
*/
public data class DeploymentIoTJobConfigurationArgs(
public val abortConfig: Output? = null,
public val jobExecutionsRolloutConfig: Output? =
null,
public val timeoutConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.greengrassv2.inputs.DeploymentIoTJobConfigurationArgs = com.pulumi.awsnative.greengrassv2.inputs.DeploymentIoTJobConfigurationArgs.builder()
.abortConfig(abortConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.jobExecutionsRolloutConfig(
jobExecutionsRolloutConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.timeoutConfig(timeoutConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DeploymentIoTJobConfigurationArgs].
*/
@PulumiTagMarker
public class DeploymentIoTJobConfigurationArgsBuilder internal constructor() {
private var abortConfig: Output? = null
private var jobExecutionsRolloutConfig: Output? =
null
private var timeoutConfig: Output? = null
/**
* @param value The stop configuration for the job. This configuration defines when and how to stop a job rollout.
*/
@JvmName("tlhxrvmddfuycflc")
public suspend fun abortConfig(`value`: Output) {
this.abortConfig = value
}
/**
* @param value The rollout configuration for the job. This configuration defines the rate at which the job rolls out to the fleet of target devices.
*/
@JvmName("whhgjvsqictnihew")
public suspend fun jobExecutionsRolloutConfig(`value`: Output) {
this.jobExecutionsRolloutConfig = value
}
/**
* @param value The timeout configuration for the job. This configuration defines the amount of time each device has to complete the job.
*/
@JvmName("dfumtfeacrsfthdu")
public suspend fun timeoutConfig(`value`: Output) {
this.timeoutConfig = value
}
/**
* @param value The stop configuration for the job. This configuration defines when and how to stop a job rollout.
*/
@JvmName("nugrxukxyqjvlbqj")
public suspend fun abortConfig(`value`: DeploymentIoTJobAbortConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.abortConfig = mapped
}
/**
* @param argument The stop configuration for the job. This configuration defines when and how to stop a job rollout.
*/
@JvmName("leqiuougssegihdi")
public suspend fun abortConfig(argument: suspend DeploymentIoTJobAbortConfigArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentIoTJobAbortConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.abortConfig = mapped
}
/**
* @param value The rollout configuration for the job. This configuration defines the rate at which the job rolls out to the fleet of target devices.
*/
@JvmName("opvoewaossvqdkms")
public suspend fun jobExecutionsRolloutConfig(`value`: DeploymentIoTJobExecutionsRolloutConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jobExecutionsRolloutConfig = mapped
}
/**
* @param argument The rollout configuration for the job. This configuration defines the rate at which the job rolls out to the fleet of target devices.
*/
@JvmName("gymlbowyouyvvdrj")
public suspend fun jobExecutionsRolloutConfig(argument: suspend DeploymentIoTJobExecutionsRolloutConfigArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentIoTJobExecutionsRolloutConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.jobExecutionsRolloutConfig = mapped
}
/**
* @param value The timeout configuration for the job. This configuration defines the amount of time each device has to complete the job.
*/
@JvmName("thwxtctklwhudmgd")
public suspend fun timeoutConfig(`value`: DeploymentIoTJobTimeoutConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutConfig = mapped
}
/**
* @param argument The timeout configuration for the job. This configuration defines the amount of time each device has to complete the job.
*/
@JvmName("qkwhojsdnlyommfa")
public suspend fun timeoutConfig(argument: suspend DeploymentIoTJobTimeoutConfigArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentIoTJobTimeoutConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.timeoutConfig = mapped
}
internal fun build(): DeploymentIoTJobConfigurationArgs = DeploymentIoTJobConfigurationArgs(
abortConfig = abortConfig,
jobExecutionsRolloutConfig = jobExecutionsRolloutConfig,
timeoutConfig = timeoutConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy