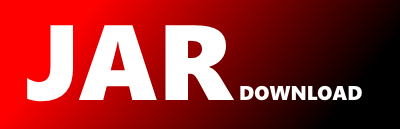
com.pulumi.awsnative.iot.kotlin.JobTemplate.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iot.kotlin
import com.pulumi.awsnative.iot.kotlin.outputs.AbortConfigProperties
import com.pulumi.awsnative.iot.kotlin.outputs.JobExecutionsRetryConfigProperties
import com.pulumi.awsnative.iot.kotlin.outputs.JobExecutionsRolloutConfigProperties
import com.pulumi.awsnative.iot.kotlin.outputs.JobTemplateMaintenanceWindow
import com.pulumi.awsnative.iot.kotlin.outputs.PresignedUrlConfigProperties
import com.pulumi.awsnative.iot.kotlin.outputs.TimeoutConfigProperties
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.iot.kotlin.outputs.AbortConfigProperties.Companion.toKotlin as abortConfigPropertiesToKotlin
import com.pulumi.awsnative.iot.kotlin.outputs.JobExecutionsRetryConfigProperties.Companion.toKotlin as jobExecutionsRetryConfigPropertiesToKotlin
import com.pulumi.awsnative.iot.kotlin.outputs.JobExecutionsRolloutConfigProperties.Companion.toKotlin as jobExecutionsRolloutConfigPropertiesToKotlin
import com.pulumi.awsnative.iot.kotlin.outputs.JobTemplateMaintenanceWindow.Companion.toKotlin as jobTemplateMaintenanceWindowToKotlin
import com.pulumi.awsnative.iot.kotlin.outputs.PresignedUrlConfigProperties.Companion.toKotlin as presignedUrlConfigPropertiesToKotlin
import com.pulumi.awsnative.iot.kotlin.outputs.TimeoutConfigProperties.Companion.toKotlin as timeoutConfigPropertiesToKotlin
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag.Companion.toKotlin as createOnlyTagToKotlin
/**
* Builder for [JobTemplate].
*/
@PulumiTagMarker
public class JobTemplateResourceBuilder internal constructor() {
public var name: String? = null
public var args: JobTemplateArgs = JobTemplateArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend JobTemplateArgsBuilder.() -> Unit) {
val builder = JobTemplateArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): JobTemplate {
val builtJavaResource = com.pulumi.awsnative.iot.JobTemplate(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return JobTemplate(builtJavaResource)
}
}
/**
* Job templates enable you to preconfigure jobs so that you can deploy them to multiple sets of target devices.
*/
public class JobTemplate internal constructor(
override val javaResource: com.pulumi.awsnative.iot.JobTemplate,
) : KotlinCustomResource(javaResource, JobTemplateMapper) {
/**
* The criteria that determine when and how a job abort takes place.
*/
public val abortConfig: Output?
get() = javaResource.abortConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
abortConfigPropertiesToKotlin(args0)
})
}).orElse(null)
})
/**
* The ARN of the job to use as the basis for the job template.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* A description of the Job Template.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* The package version Amazon Resource Names (ARNs) that are installed on the device’s reserved named shadow ( `$package` ) when the job successfully completes.
* *Note:* Up to 25 package version ARNS are allowed.
*/
public val destinationPackageVersions: Output>?
get() = javaResource.destinationPackageVersions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The job document. Required if you don't specify a value for documentSource.
*/
public val document: Output?
get() = javaResource.document().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* An S3 link to the job document to use in the template. Required if you don't specify a value for document.
*/
public val documentSource: Output?
get() = javaResource.documentSource().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Optional for copying a JobTemplate from a pre-existing Job configuration.
*/
public val jobArn: Output?
get() = javaResource.jobArn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Allows you to create the criteria to retry a job.
*/
public val jobExecutionsRetryConfig: Output?
get() = javaResource.jobExecutionsRetryConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> jobExecutionsRetryConfigPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* Allows you to create a staged rollout of a job.
*/
public val jobExecutionsRolloutConfig: Output?
get() = javaResource.jobExecutionsRolloutConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> jobExecutionsRolloutConfigPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* A unique identifier for the job template. We recommend using a UUID. Alpha-numeric characters, "-", and "_" are valid for use here.
*/
public val jobTemplateId: Output
get() = javaResource.jobTemplateId().applyValue({ args0 -> args0 })
/**
* An optional configuration within the SchedulingConfig to setup a recurring maintenance window with a predetermined start time and duration for the rollout of a job document to all devices in a target group for a job.
*/
public val maintenanceWindows: Output>?
get() = javaResource.maintenanceWindows().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
jobTemplateMaintenanceWindowToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Configuration for pre-signed S3 URLs.
*/
public val presignedUrlConfig: Output?
get() = javaResource.presignedUrlConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> presignedUrlConfigPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* Metadata that can be used to manage the JobTemplate.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> createOnlyTagToKotlin(args0) })
})
}).orElse(null)
})
/**
* Specifies the amount of time each device has to finish its execution of the job.
*/
public val timeoutConfig: Output?
get() = javaResource.timeoutConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> timeoutConfigPropertiesToKotlin(args0) })
}).orElse(null)
})
}
public object JobTemplateMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.iot.JobTemplate::class == javaResource::class
override fun map(javaResource: Resource): JobTemplate = JobTemplate(
javaResource as
com.pulumi.awsnative.iot.JobTemplate,
)
}
/**
* @see [JobTemplate].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [JobTemplate].
*/
public suspend fun jobTemplate(name: String, block: suspend JobTemplateResourceBuilder.() -> Unit): JobTemplate {
val builder = JobTemplateResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [JobTemplate].
* @param name The _unique_ name of the resulting resource.
*/
public fun jobTemplate(name: String): JobTemplate {
val builder = JobTemplateResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy