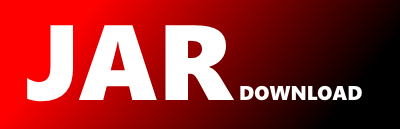
com.pulumi.awsnative.iot.kotlin.inputs.TopicRuleLocationActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iot.kotlin.inputs
import com.pulumi.awsnative.iot.inputs.TopicRuleLocationActionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property deviceId The unique ID of the device providing the location data.
* @property latitude A string that evaluates to a double value that represents the latitude of the device's location.
* @property longitude A string that evaluates to a double value that represents the longitude of the device's location.
* @property roleArn The IAM role that grants permission to write to the Amazon Location resource.
* @property timestamp The time that the location data was sampled. The default value is the time the MQTT message was processed.
* @property trackerName The name of the tracker resource in Amazon Location in which the location is updated.
*/
public data class TopicRuleLocationActionArgs(
public val deviceId: Output,
public val latitude: Output,
public val longitude: Output,
public val roleArn: Output,
public val timestamp: Output? = null,
public val trackerName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.iot.inputs.TopicRuleLocationActionArgs =
com.pulumi.awsnative.iot.inputs.TopicRuleLocationActionArgs.builder()
.deviceId(deviceId.applyValue({ args0 -> args0 }))
.latitude(latitude.applyValue({ args0 -> args0 }))
.longitude(longitude.applyValue({ args0 -> args0 }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.timestamp(timestamp?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.trackerName(trackerName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TopicRuleLocationActionArgs].
*/
@PulumiTagMarker
public class TopicRuleLocationActionArgsBuilder internal constructor() {
private var deviceId: Output? = null
private var latitude: Output? = null
private var longitude: Output? = null
private var roleArn: Output? = null
private var timestamp: Output? = null
private var trackerName: Output? = null
/**
* @param value The unique ID of the device providing the location data.
*/
@JvmName("kbhvctjcdbbnlvau")
public suspend fun deviceId(`value`: Output) {
this.deviceId = value
}
/**
* @param value A string that evaluates to a double value that represents the latitude of the device's location.
*/
@JvmName("rdthutojiwxrnwdm")
public suspend fun latitude(`value`: Output) {
this.latitude = value
}
/**
* @param value A string that evaluates to a double value that represents the longitude of the device's location.
*/
@JvmName("avvysuhubgtxkilb")
public suspend fun longitude(`value`: Output) {
this.longitude = value
}
/**
* @param value The IAM role that grants permission to write to the Amazon Location resource.
*/
@JvmName("wddddirejhfmsubb")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The time that the location data was sampled. The default value is the time the MQTT message was processed.
*/
@JvmName("xnhfraifpiibqkml")
public suspend fun timestamp(`value`: Output) {
this.timestamp = value
}
/**
* @param value The name of the tracker resource in Amazon Location in which the location is updated.
*/
@JvmName("xhjsiywabiynfdlh")
public suspend fun trackerName(`value`: Output) {
this.trackerName = value
}
/**
* @param value The unique ID of the device providing the location data.
*/
@JvmName("sovghvrbbxbajbuh")
public suspend fun deviceId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.deviceId = mapped
}
/**
* @param value A string that evaluates to a double value that represents the latitude of the device's location.
*/
@JvmName("vsrlmtftlogcraaa")
public suspend fun latitude(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.latitude = mapped
}
/**
* @param value A string that evaluates to a double value that represents the longitude of the device's location.
*/
@JvmName("tcgfgdoedmswbrry")
public suspend fun longitude(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.longitude = mapped
}
/**
* @param value The IAM role that grants permission to write to the Amazon Location resource.
*/
@JvmName("urqeowwmfocynhag")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value The time that the location data was sampled. The default value is the time the MQTT message was processed.
*/
@JvmName("iefslwfkewfynpht")
public suspend fun timestamp(`value`: TopicRuleTimestampArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timestamp = mapped
}
/**
* @param argument The time that the location data was sampled. The default value is the time the MQTT message was processed.
*/
@JvmName("lekawlclqgfiplhb")
public suspend fun timestamp(argument: suspend TopicRuleTimestampArgsBuilder.() -> Unit) {
val toBeMapped = TopicRuleTimestampArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.timestamp = mapped
}
/**
* @param value The name of the tracker resource in Amazon Location in which the location is updated.
*/
@JvmName("xbndlumbwwbxblia")
public suspend fun trackerName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.trackerName = mapped
}
internal fun build(): TopicRuleLocationActionArgs = TopicRuleLocationActionArgs(
deviceId = deviceId ?: throw PulumiNullFieldException("deviceId"),
latitude = latitude ?: throw PulumiNullFieldException("latitude"),
longitude = longitude ?: throw PulumiNullFieldException("longitude"),
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
timestamp = timestamp,
trackerName = trackerName ?: throw PulumiNullFieldException("trackerName"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy