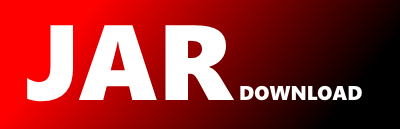
com.pulumi.awsnative.iotevents.kotlin.inputs.DetectorModelDynamoDbArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.iotevents.kotlin.inputs
import com.pulumi.awsnative.iotevents.inputs.DetectorModelDynamoDbArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that have the information about the detector model instance and the event that triggered the action. You can also customize the [payload](https://docs.aws.amazon.com/iotevents/latest/apireference/API_Payload.html). One column of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more information, see [Actions](https://docs.aws.amazon.com/iotevents/latest/developerguide/iotevents-event-actions.html) in *AWS IoT Events Developer Guide*.
* @property hashKeyField The name of the hash key (also called the partition key).
* @property hashKeyType The data type for the hash key (also called the partition key). You can specify the following values:
* * `STRING` - The hash key is a string.
* * `NUMBER` - The hash key is a number.
* If you don't specify `hashKeyType`, the default value is `STRING`.
* @property hashKeyValue The value of the hash key (also called the partition key).
* @property operation The type of operation to perform. You can specify the following values:
* * `INSERT` - Insert data as a new item into the DynamoDB table. This item uses the specified hash key as a partition key. If you specified a range key, the item uses the range key as a sort key.
* * `UPDATE` - Update an existing item of the DynamoDB table with new data. This item's partition key must match the specified hash key. If you specified a range key, the range key must match the item's sort key.
* * `DELETE` - Delete an existing item of the DynamoDB table. This item's partition key must match the specified hash key. If you specified a range key, the range key must match the item's sort key.
* If you don't specify this parameter, AWS IoT Events triggers the `INSERT` operation.
* @property payload Information needed to configure the payload.
* By default, AWS IoT Events generates a standard payload in JSON for any action. This action payload contains all attribute-value pairs that have the information about the detector model instance and the event triggered the action. To configure the action payload, you can use `contentExpression` .
* @property payloadField The name of the DynamoDB column that receives the action payload.
* If you don't specify this parameter, the name of the DynamoDB column is `payload`.
* @property rangeKeyField The name of the range key (also called the sort key).
* @property rangeKeyType The data type for the range key (also called the sort key), You can specify the following values:
* * `STRING` - The range key is a string.
* * `NUMBER` - The range key is number.
* If you don't specify `rangeKeyField`, the default value is `STRING`.
* @property rangeKeyValue The value of the range key (also called the sort key).
* @property tableName The name of the DynamoDB table.
*/
public data class DetectorModelDynamoDbArgs(
public val hashKeyField: Output,
public val hashKeyType: Output? = null,
public val hashKeyValue: Output,
public val operation: Output? = null,
public val payload: Output? = null,
public val payloadField: Output? = null,
public val rangeKeyField: Output? = null,
public val rangeKeyType: Output? = null,
public val rangeKeyValue: Output? = null,
public val tableName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.iotevents.inputs.DetectorModelDynamoDbArgs =
com.pulumi.awsnative.iotevents.inputs.DetectorModelDynamoDbArgs.builder()
.hashKeyField(hashKeyField.applyValue({ args0 -> args0 }))
.hashKeyType(hashKeyType?.applyValue({ args0 -> args0 }))
.hashKeyValue(hashKeyValue.applyValue({ args0 -> args0 }))
.operation(operation?.applyValue({ args0 -> args0 }))
.payload(payload?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.payloadField(payloadField?.applyValue({ args0 -> args0 }))
.rangeKeyField(rangeKeyField?.applyValue({ args0 -> args0 }))
.rangeKeyType(rangeKeyType?.applyValue({ args0 -> args0 }))
.rangeKeyValue(rangeKeyValue?.applyValue({ args0 -> args0 }))
.tableName(tableName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DetectorModelDynamoDbArgs].
*/
@PulumiTagMarker
public class DetectorModelDynamoDbArgsBuilder internal constructor() {
private var hashKeyField: Output? = null
private var hashKeyType: Output? = null
private var hashKeyValue: Output? = null
private var operation: Output? = null
private var payload: Output? = null
private var payloadField: Output? = null
private var rangeKeyField: Output? = null
private var rangeKeyType: Output? = null
private var rangeKeyValue: Output? = null
private var tableName: Output? = null
/**
* @param value The name of the hash key (also called the partition key).
*/
@JvmName("khgstatgssdnkiad")
public suspend fun hashKeyField(`value`: Output) {
this.hashKeyField = value
}
/**
* @param value The data type for the hash key (also called the partition key). You can specify the following values:
* * `STRING` - The hash key is a string.
* * `NUMBER` - The hash key is a number.
* If you don't specify `hashKeyType`, the default value is `STRING`.
*/
@JvmName("rjsxwwfjbahqybcv")
public suspend fun hashKeyType(`value`: Output) {
this.hashKeyType = value
}
/**
* @param value The value of the hash key (also called the partition key).
*/
@JvmName("abkpaxdjbgylvmvg")
public suspend fun hashKeyValue(`value`: Output) {
this.hashKeyValue = value
}
/**
* @param value The type of operation to perform. You can specify the following values:
* * `INSERT` - Insert data as a new item into the DynamoDB table. This item uses the specified hash key as a partition key. If you specified a range key, the item uses the range key as a sort key.
* * `UPDATE` - Update an existing item of the DynamoDB table with new data. This item's partition key must match the specified hash key. If you specified a range key, the range key must match the item's sort key.
* * `DELETE` - Delete an existing item of the DynamoDB table. This item's partition key must match the specified hash key. If you specified a range key, the range key must match the item's sort key.
* If you don't specify this parameter, AWS IoT Events triggers the `INSERT` operation.
*/
@JvmName("vlswwhccagsscwtl")
public suspend fun operation(`value`: Output) {
this.operation = value
}
/**
* @param value Information needed to configure the payload.
* By default, AWS IoT Events generates a standard payload in JSON for any action. This action payload contains all attribute-value pairs that have the information about the detector model instance and the event triggered the action. To configure the action payload, you can use `contentExpression` .
*/
@JvmName("tvowbhcosyovupmf")
public suspend fun payload(`value`: Output) {
this.payload = value
}
/**
* @param value The name of the DynamoDB column that receives the action payload.
* If you don't specify this parameter, the name of the DynamoDB column is `payload`.
*/
@JvmName("fxomjnfmgargtxow")
public suspend fun payloadField(`value`: Output) {
this.payloadField = value
}
/**
* @param value The name of the range key (also called the sort key).
*/
@JvmName("iskhkcwlbktupihc")
public suspend fun rangeKeyField(`value`: Output) {
this.rangeKeyField = value
}
/**
* @param value The data type for the range key (also called the sort key), You can specify the following values:
* * `STRING` - The range key is a string.
* * `NUMBER` - The range key is number.
* If you don't specify `rangeKeyField`, the default value is `STRING`.
*/
@JvmName("iniuutypnddsrubg")
public suspend fun rangeKeyType(`value`: Output) {
this.rangeKeyType = value
}
/**
* @param value The value of the range key (also called the sort key).
*/
@JvmName("nmlaepavjurayeyi")
public suspend fun rangeKeyValue(`value`: Output) {
this.rangeKeyValue = value
}
/**
* @param value The name of the DynamoDB table.
*/
@JvmName("foljybaawuburkcb")
public suspend fun tableName(`value`: Output) {
this.tableName = value
}
/**
* @param value The name of the hash key (also called the partition key).
*/
@JvmName("ksbnjsegpcfyrmjf")
public suspend fun hashKeyField(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.hashKeyField = mapped
}
/**
* @param value The data type for the hash key (also called the partition key). You can specify the following values:
* * `STRING` - The hash key is a string.
* * `NUMBER` - The hash key is a number.
* If you don't specify `hashKeyType`, the default value is `STRING`.
*/
@JvmName("vdjkpywrtpkyyvqx")
public suspend fun hashKeyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hashKeyType = mapped
}
/**
* @param value The value of the hash key (also called the partition key).
*/
@JvmName("ojkelcdejslxundc")
public suspend fun hashKeyValue(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.hashKeyValue = mapped
}
/**
* @param value The type of operation to perform. You can specify the following values:
* * `INSERT` - Insert data as a new item into the DynamoDB table. This item uses the specified hash key as a partition key. If you specified a range key, the item uses the range key as a sort key.
* * `UPDATE` - Update an existing item of the DynamoDB table with new data. This item's partition key must match the specified hash key. If you specified a range key, the range key must match the item's sort key.
* * `DELETE` - Delete an existing item of the DynamoDB table. This item's partition key must match the specified hash key. If you specified a range key, the range key must match the item's sort key.
* If you don't specify this parameter, AWS IoT Events triggers the `INSERT` operation.
*/
@JvmName("yboofacnblespcns")
public suspend fun operation(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.operation = mapped
}
/**
* @param value Information needed to configure the payload.
* By default, AWS IoT Events generates a standard payload in JSON for any action. This action payload contains all attribute-value pairs that have the information about the detector model instance and the event triggered the action. To configure the action payload, you can use `contentExpression` .
*/
@JvmName("hpdaoggdxiqviyiv")
public suspend fun payload(`value`: DetectorModelPayloadArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.payload = mapped
}
/**
* @param argument Information needed to configure the payload.
* By default, AWS IoT Events generates a standard payload in JSON for any action. This action payload contains all attribute-value pairs that have the information about the detector model instance and the event triggered the action. To configure the action payload, you can use `contentExpression` .
*/
@JvmName("nqbieehipksabsxi")
public suspend fun payload(argument: suspend DetectorModelPayloadArgsBuilder.() -> Unit) {
val toBeMapped = DetectorModelPayloadArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.payload = mapped
}
/**
* @param value The name of the DynamoDB column that receives the action payload.
* If you don't specify this parameter, the name of the DynamoDB column is `payload`.
*/
@JvmName("yptgbyicmhdpbhgk")
public suspend fun payloadField(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.payloadField = mapped
}
/**
* @param value The name of the range key (also called the sort key).
*/
@JvmName("ycddfarbuetkqrvs")
public suspend fun rangeKeyField(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeKeyField = mapped
}
/**
* @param value The data type for the range key (also called the sort key), You can specify the following values:
* * `STRING` - The range key is a string.
* * `NUMBER` - The range key is number.
* If you don't specify `rangeKeyField`, the default value is `STRING`.
*/
@JvmName("bnnpweggewsssyha")
public suspend fun rangeKeyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeKeyType = mapped
}
/**
* @param value The value of the range key (also called the sort key).
*/
@JvmName("kticdrpytxyjcajr")
public suspend fun rangeKeyValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeKeyValue = mapped
}
/**
* @param value The name of the DynamoDB table.
*/
@JvmName("ecdyfhacscwxwewk")
public suspend fun tableName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tableName = mapped
}
internal fun build(): DetectorModelDynamoDbArgs = DetectorModelDynamoDbArgs(
hashKeyField = hashKeyField ?: throw PulumiNullFieldException("hashKeyField"),
hashKeyType = hashKeyType,
hashKeyValue = hashKeyValue ?: throw PulumiNullFieldException("hashKeyValue"),
operation = operation,
payload = payload,
payloadField = payloadField,
rangeKeyField = rangeKeyField,
rangeKeyType = rangeKeyType,
rangeKeyValue = rangeKeyValue,
tableName = tableName ?: throw PulumiNullFieldException("tableName"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy