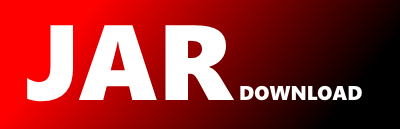
com.pulumi.awsnative.kendra.kotlin.inputs.DataSourceConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kendra.kotlin.inputs
import com.pulumi.awsnative.kendra.inputs.DataSourceConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property confluenceConfiguration Provides the configuration information to connect to Confluence as your data source.
* @property databaseConfiguration Provides the configuration information to connect to a database as your data source.
* @property googleDriveConfiguration Provides the configuration information to connect to Google Drive as your data source.
* @property oneDriveConfiguration Provides the configuration information to connect to Microsoft OneDrive as your data source.
* @property s3Configuration Provides the configuration information to connect to an Amazon S3 bucket as your data source.
* > Amazon Kendra now supports an upgraded Amazon S3 connector.
* >
* > You must now use the [TemplateConfiguration](https://docs.aws.amazon.com/kendra/latest/APIReference/API_TemplateConfiguration.html) object instead of the `S3DataSourceConfiguration` object to configure your connector.
* >
* > Connectors configured using the older console and API architecture will continue to function as configured. However, you won't be able to edit or update them. If you want to edit or update your connector configuration, you must create a new connector.
* >
* > We recommended migrating your connector workflow to the upgraded version. Support for connectors configured using the older architecture is scheduled to end by June 2024.
* @property salesforceConfiguration Provides the configuration information to connect to Salesforce as your data source.
* @property serviceNowConfiguration Provides the configuration information to connect to ServiceNow as your data source.
* @property sharePointConfiguration Provides the configuration information to connect to Microsoft SharePoint as your data source.
* @property webCrawlerConfiguration Provides the configuration information required for Amazon Kendra Web Crawler.
* @property workDocsConfiguration Provides the configuration information to connect to Amazon WorkDocs as your data source.
*/
public data class DataSourceConfigurationArgs(
public val confluenceConfiguration: Output? = null,
public val databaseConfiguration: Output? = null,
public val googleDriveConfiguration: Output? = null,
public val oneDriveConfiguration: Output? = null,
public val s3Configuration: Output? = null,
public val salesforceConfiguration: Output? = null,
public val serviceNowConfiguration: Output? = null,
public val sharePointConfiguration: Output? = null,
public val webCrawlerConfiguration: Output? = null,
public val workDocsConfiguration: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.kendra.inputs.DataSourceConfigurationArgs =
com.pulumi.awsnative.kendra.inputs.DataSourceConfigurationArgs.builder()
.confluenceConfiguration(
confluenceConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.databaseConfiguration(
databaseConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.googleDriveConfiguration(
googleDriveConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.oneDriveConfiguration(
oneDriveConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.s3Configuration(s3Configuration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.salesforceConfiguration(
salesforceConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.serviceNowConfiguration(
serviceNowConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sharePointConfiguration(
sharePointConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.webCrawlerConfiguration(
webCrawlerConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.workDocsConfiguration(
workDocsConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DataSourceConfigurationArgs].
*/
@PulumiTagMarker
public class DataSourceConfigurationArgsBuilder internal constructor() {
private var confluenceConfiguration: Output? = null
private var databaseConfiguration: Output? = null
private var googleDriveConfiguration: Output? = null
private var oneDriveConfiguration: Output? = null
private var s3Configuration: Output? = null
private var salesforceConfiguration: Output? = null
private var serviceNowConfiguration: Output? = null
private var sharePointConfiguration: Output? = null
private var webCrawlerConfiguration: Output? = null
private var workDocsConfiguration: Output? = null
/**
* @param value Provides the configuration information to connect to Confluence as your data source.
*/
@JvmName("abmssgvxxfeehcxr")
public suspend fun confluenceConfiguration(`value`: Output) {
this.confluenceConfiguration = value
}
/**
* @param value Provides the configuration information to connect to a database as your data source.
*/
@JvmName("fbbdekowhuhotbkw")
public suspend fun databaseConfiguration(`value`: Output) {
this.databaseConfiguration = value
}
/**
* @param value Provides the configuration information to connect to Google Drive as your data source.
*/
@JvmName("dtuusgqjrgsmmiff")
public suspend fun googleDriveConfiguration(`value`: Output) {
this.googleDriveConfiguration = value
}
/**
* @param value Provides the configuration information to connect to Microsoft OneDrive as your data source.
*/
@JvmName("ynfvokxttsupabsw")
public suspend fun oneDriveConfiguration(`value`: Output) {
this.oneDriveConfiguration = value
}
/**
* @param value Provides the configuration information to connect to an Amazon S3 bucket as your data source.
* > Amazon Kendra now supports an upgraded Amazon S3 connector.
* >
* > You must now use the [TemplateConfiguration](https://docs.aws.amazon.com/kendra/latest/APIReference/API_TemplateConfiguration.html) object instead of the `S3DataSourceConfiguration` object to configure your connector.
* >
* > Connectors configured using the older console and API architecture will continue to function as configured. However, you won't be able to edit or update them. If you want to edit or update your connector configuration, you must create a new connector.
* >
* > We recommended migrating your connector workflow to the upgraded version. Support for connectors configured using the older architecture is scheduled to end by June 2024.
*/
@JvmName("wirkhlcrtrbaovlv")
public suspend fun s3Configuration(`value`: Output) {
this.s3Configuration = value
}
/**
* @param value Provides the configuration information to connect to Salesforce as your data source.
*/
@JvmName("afjuigsdtbmbwwqx")
public suspend fun salesforceConfiguration(`value`: Output) {
this.salesforceConfiguration = value
}
/**
* @param value Provides the configuration information to connect to ServiceNow as your data source.
*/
@JvmName("ymwwgtithexrjasq")
public suspend fun serviceNowConfiguration(`value`: Output) {
this.serviceNowConfiguration = value
}
/**
* @param value Provides the configuration information to connect to Microsoft SharePoint as your data source.
*/
@JvmName("iocatylnukbljtor")
public suspend fun sharePointConfiguration(`value`: Output) {
this.sharePointConfiguration = value
}
/**
* @param value Provides the configuration information required for Amazon Kendra Web Crawler.
*/
@JvmName("wltqaqfqvapebsvf")
public suspend fun webCrawlerConfiguration(`value`: Output) {
this.webCrawlerConfiguration = value
}
/**
* @param value Provides the configuration information to connect to Amazon WorkDocs as your data source.
*/
@JvmName("drtircqjahtwcjxx")
public suspend fun workDocsConfiguration(`value`: Output) {
this.workDocsConfiguration = value
}
/**
* @param value Provides the configuration information to connect to Confluence as your data source.
*/
@JvmName("moyxwvnrhrklgsel")
public suspend fun confluenceConfiguration(`value`: DataSourceConfluenceConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.confluenceConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to Confluence as your data source.
*/
@JvmName("mdocxsoseophttww")
public suspend fun confluenceConfiguration(argument: suspend DataSourceConfluenceConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceConfluenceConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.confluenceConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to a database as your data source.
*/
@JvmName("ppfokdhxeaxysfcu")
public suspend fun databaseConfiguration(`value`: DataSourceDatabaseConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to a database as your data source.
*/
@JvmName("frocregwixuiuwin")
public suspend fun databaseConfiguration(argument: suspend DataSourceDatabaseConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceDatabaseConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.databaseConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to Google Drive as your data source.
*/
@JvmName("ruwmfysnpkefwhgv")
public suspend fun googleDriveConfiguration(`value`: DataSourceGoogleDriveConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.googleDriveConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to Google Drive as your data source.
*/
@JvmName("eshvrmuholdaauok")
public suspend fun googleDriveConfiguration(argument: suspend DataSourceGoogleDriveConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceGoogleDriveConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.googleDriveConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to Microsoft OneDrive as your data source.
*/
@JvmName("uyjetlpkahauglnd")
public suspend fun oneDriveConfiguration(`value`: DataSourceOneDriveConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.oneDriveConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to Microsoft OneDrive as your data source.
*/
@JvmName("pkjwuwmklfqjpegp")
public suspend fun oneDriveConfiguration(argument: suspend DataSourceOneDriveConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceOneDriveConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.oneDriveConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to an Amazon S3 bucket as your data source.
* > Amazon Kendra now supports an upgraded Amazon S3 connector.
* >
* > You must now use the [TemplateConfiguration](https://docs.aws.amazon.com/kendra/latest/APIReference/API_TemplateConfiguration.html) object instead of the `S3DataSourceConfiguration` object to configure your connector.
* >
* > Connectors configured using the older console and API architecture will continue to function as configured. However, you won't be able to edit or update them. If you want to edit or update your connector configuration, you must create a new connector.
* >
* > We recommended migrating your connector workflow to the upgraded version. Support for connectors configured using the older architecture is scheduled to end by June 2024.
*/
@JvmName("wwqotlfiothqfvkv")
public suspend fun s3Configuration(`value`: DataSourceS3DataSourceConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3Configuration = mapped
}
/**
* @param argument Provides the configuration information to connect to an Amazon S3 bucket as your data source.
* > Amazon Kendra now supports an upgraded Amazon S3 connector.
* >
* > You must now use the [TemplateConfiguration](https://docs.aws.amazon.com/kendra/latest/APIReference/API_TemplateConfiguration.html) object instead of the `S3DataSourceConfiguration` object to configure your connector.
* >
* > Connectors configured using the older console and API architecture will continue to function as configured. However, you won't be able to edit or update them. If you want to edit or update your connector configuration, you must create a new connector.
* >
* > We recommended migrating your connector workflow to the upgraded version. Support for connectors configured using the older architecture is scheduled to end by June 2024.
*/
@JvmName("wmltnsxyslbbbmbp")
public suspend fun s3Configuration(argument: suspend DataSourceS3DataSourceConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceS3DataSourceConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.s3Configuration = mapped
}
/**
* @param value Provides the configuration information to connect to Salesforce as your data source.
*/
@JvmName("kwdbvspdbnyrnphl")
public suspend fun salesforceConfiguration(`value`: DataSourceSalesforceConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.salesforceConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to Salesforce as your data source.
*/
@JvmName("hwnhoeuybgnxlall")
public suspend fun salesforceConfiguration(argument: suspend DataSourceSalesforceConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceSalesforceConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.salesforceConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to ServiceNow as your data source.
*/
@JvmName("rsefadipjdtyxtae")
public suspend fun serviceNowConfiguration(`value`: DataSourceServiceNowConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceNowConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to ServiceNow as your data source.
*/
@JvmName("umyogvxvkcxsxiat")
public suspend fun serviceNowConfiguration(argument: suspend DataSourceServiceNowConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceServiceNowConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.serviceNowConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to Microsoft SharePoint as your data source.
*/
@JvmName("syoetwwwrqrajubb")
public suspend fun sharePointConfiguration(`value`: DataSourceSharePointConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharePointConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to Microsoft SharePoint as your data source.
*/
@JvmName("stmciyillqtjalxv")
public suspend fun sharePointConfiguration(argument: suspend DataSourceSharePointConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceSharePointConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sharePointConfiguration = mapped
}
/**
* @param value Provides the configuration information required for Amazon Kendra Web Crawler.
*/
@JvmName("bifjjdkjqhxivssm")
public suspend fun webCrawlerConfiguration(`value`: DataSourceWebCrawlerConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.webCrawlerConfiguration = mapped
}
/**
* @param argument Provides the configuration information required for Amazon Kendra Web Crawler.
*/
@JvmName("spagusecqyktqlqh")
public suspend fun webCrawlerConfiguration(argument: suspend DataSourceWebCrawlerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceWebCrawlerConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.webCrawlerConfiguration = mapped
}
/**
* @param value Provides the configuration information to connect to Amazon WorkDocs as your data source.
*/
@JvmName("dwpddyvqxodpnbwq")
public suspend fun workDocsConfiguration(`value`: DataSourceWorkDocsConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workDocsConfiguration = mapped
}
/**
* @param argument Provides the configuration information to connect to Amazon WorkDocs as your data source.
*/
@JvmName("xebovwrjpgxvfkfn")
public suspend fun workDocsConfiguration(argument: suspend DataSourceWorkDocsConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceWorkDocsConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.workDocsConfiguration = mapped
}
internal fun build(): DataSourceConfigurationArgs = DataSourceConfigurationArgs(
confluenceConfiguration = confluenceConfiguration,
databaseConfiguration = databaseConfiguration,
googleDriveConfiguration = googleDriveConfiguration,
oneDriveConfiguration = oneDriveConfiguration,
s3Configuration = s3Configuration,
salesforceConfiguration = salesforceConfiguration,
serviceNowConfiguration = serviceNowConfiguration,
sharePointConfiguration = sharePointConfiguration,
webCrawlerConfiguration = webCrawlerConfiguration,
workDocsConfiguration = workDocsConfiguration,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy