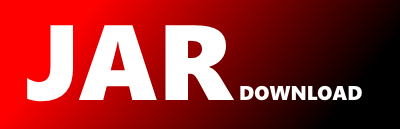
com.pulumi.awsnative.kendra.kotlin.inputs.DataSourceDatabaseConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kendra.kotlin.inputs
import com.pulumi.awsnative.kendra.inputs.DataSourceDatabaseConfigurationArgs.builder
import com.pulumi.awsnative.kendra.kotlin.enums.DataSourceDatabaseEngineType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property aclConfiguration Information about the database column that provides information for user context filtering.
* @property columnConfiguration Information about where the index should get the document information from the database.
* @property connectionConfiguration Configuration information that's required to connect to a database.
* @property databaseEngineType The type of database engine that runs the database.
* @property sqlConfiguration Provides information about how Amazon Kendra uses quote marks around SQL identifiers when querying a database data source.
* @property vpcConfiguration Provides information for connecting to an Amazon VPC.
*/
public data class DataSourceDatabaseConfigurationArgs(
public val aclConfiguration: Output? = null,
public val columnConfiguration: Output,
public val connectionConfiguration: Output,
public val databaseEngineType: Output,
public val sqlConfiguration: Output? = null,
public val vpcConfiguration: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.kendra.inputs.DataSourceDatabaseConfigurationArgs =
com.pulumi.awsnative.kendra.inputs.DataSourceDatabaseConfigurationArgs.builder()
.aclConfiguration(aclConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.columnConfiguration(
columnConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.connectionConfiguration(
connectionConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.databaseEngineType(
databaseEngineType.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sqlConfiguration(sqlConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.vpcConfiguration(
vpcConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DataSourceDatabaseConfigurationArgs].
*/
@PulumiTagMarker
public class DataSourceDatabaseConfigurationArgsBuilder internal constructor() {
private var aclConfiguration: Output? = null
private var columnConfiguration: Output? = null
private var connectionConfiguration: Output? = null
private var databaseEngineType: Output? = null
private var sqlConfiguration: Output? = null
private var vpcConfiguration: Output? = null
/**
* @param value Information about the database column that provides information for user context filtering.
*/
@JvmName("ubekdcavgdpockqn")
public suspend fun aclConfiguration(`value`: Output) {
this.aclConfiguration = value
}
/**
* @param value Information about where the index should get the document information from the database.
*/
@JvmName("bxaxqfcxrjplmdff")
public suspend fun columnConfiguration(`value`: Output) {
this.columnConfiguration = value
}
/**
* @param value Configuration information that's required to connect to a database.
*/
@JvmName("dkihbbstxrkefwsb")
public suspend fun connectionConfiguration(`value`: Output) {
this.connectionConfiguration = value
}
/**
* @param value The type of database engine that runs the database.
*/
@JvmName("weypvgrijabodwlt")
public suspend fun databaseEngineType(`value`: Output) {
this.databaseEngineType = value
}
/**
* @param value Provides information about how Amazon Kendra uses quote marks around SQL identifiers when querying a database data source.
*/
@JvmName("xstuqfyydgnckmyi")
public suspend fun sqlConfiguration(`value`: Output) {
this.sqlConfiguration = value
}
/**
* @param value Provides information for connecting to an Amazon VPC.
*/
@JvmName("wuttfbyoxvjvobkn")
public suspend fun vpcConfiguration(`value`: Output) {
this.vpcConfiguration = value
}
/**
* @param value Information about the database column that provides information for user context filtering.
*/
@JvmName("jiatyqrvatjqrcmr")
public suspend fun aclConfiguration(`value`: DataSourceAclConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.aclConfiguration = mapped
}
/**
* @param argument Information about the database column that provides information for user context filtering.
*/
@JvmName("vuudcafejxhddvwd")
public suspend fun aclConfiguration(argument: suspend DataSourceAclConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceAclConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.aclConfiguration = mapped
}
/**
* @param value Information about where the index should get the document information from the database.
*/
@JvmName("cnhuofrqwfqfswqe")
public suspend fun columnConfiguration(`value`: DataSourceColumnConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.columnConfiguration = mapped
}
/**
* @param argument Information about where the index should get the document information from the database.
*/
@JvmName("ddyuskbxeiqplppj")
public suspend fun columnConfiguration(argument: suspend DataSourceColumnConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceColumnConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.columnConfiguration = mapped
}
/**
* @param value Configuration information that's required to connect to a database.
*/
@JvmName("npjqqscmtgkfwtvf")
public suspend fun connectionConfiguration(`value`: DataSourceConnectionConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.connectionConfiguration = mapped
}
/**
* @param argument Configuration information that's required to connect to a database.
*/
@JvmName("ncoohewkdjcritcd")
public suspend fun connectionConfiguration(argument: suspend DataSourceConnectionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceConnectionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.connectionConfiguration = mapped
}
/**
* @param value The type of database engine that runs the database.
*/
@JvmName("nyqhuiaskhddklye")
public suspend fun databaseEngineType(`value`: DataSourceDatabaseEngineType) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.databaseEngineType = mapped
}
/**
* @param value Provides information about how Amazon Kendra uses quote marks around SQL identifiers when querying a database data source.
*/
@JvmName("atqokomlliueiilt")
public suspend fun sqlConfiguration(`value`: DataSourceSqlConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqlConfiguration = mapped
}
/**
* @param argument Provides information about how Amazon Kendra uses quote marks around SQL identifiers when querying a database data source.
*/
@JvmName("bhinkvdhsyhcovgt")
public suspend fun sqlConfiguration(argument: suspend DataSourceSqlConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceSqlConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sqlConfiguration = mapped
}
/**
* @param value Provides information for connecting to an Amazon VPC.
*/
@JvmName("ktrluusrwpxlyixn")
public suspend fun vpcConfiguration(`value`: DataSourceVpcConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vpcConfiguration = mapped
}
/**
* @param argument Provides information for connecting to an Amazon VPC.
*/
@JvmName("ytljceovyqndocpm")
public suspend fun vpcConfiguration(argument: suspend DataSourceVpcConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DataSourceVpcConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.vpcConfiguration = mapped
}
internal fun build(): DataSourceDatabaseConfigurationArgs = DataSourceDatabaseConfigurationArgs(
aclConfiguration = aclConfiguration,
columnConfiguration = columnConfiguration ?: throw PulumiNullFieldException("columnConfiguration"),
connectionConfiguration = connectionConfiguration ?: throw
PulumiNullFieldException("connectionConfiguration"),
databaseEngineType = databaseEngineType ?: throw PulumiNullFieldException("databaseEngineType"),
sqlConfiguration = sqlConfiguration,
vpcConfiguration = vpcConfiguration,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy