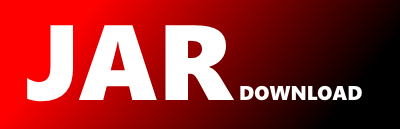
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamElasticsearchDestinationConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kinesisfirehose.kotlin.outputs
import com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamElasticsearchDestinationConfigurationIndexRotationPeriod
import com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamElasticsearchDestinationConfigurationS3BackupMode
import kotlin.String
import kotlin.Suppress
/**
*
* @property bufferingHints Configures how Kinesis Data Firehose buffers incoming data while delivering it to the Amazon ES domain.
* @property cloudWatchLoggingOptions The Amazon CloudWatch Logs logging options for the delivery stream.
* @property clusterEndpoint The endpoint to use when communicating with the cluster. Specify either this `ClusterEndpoint` or the `DomainARN` field.
* @property documentIdOptions Indicates the method for setting up document ID. The supported methods are Firehose generated document ID and OpenSearch Service generated document ID.
* @property domainArn The ARN of the Amazon ES domain. The IAM role must have permissions for `DescribeElasticsearchDomain` , `DescribeElasticsearchDomains` , and `DescribeElasticsearchDomainConfig` after assuming the role specified in *RoleARN* .
* Specify either `ClusterEndpoint` or `DomainARN` .
* @property indexName The name of the Elasticsearch index to which Kinesis Data Firehose adds data for indexing.
* @property indexRotationPeriod The frequency of Elasticsearch index rotation. If you enable index rotation, Kinesis Data Firehose appends a portion of the UTC arrival timestamp to the specified index name, and rotates the appended timestamp accordingly. For more information, see [Index Rotation for the Amazon ES Destination](https://docs.aws.amazon.com/firehose/latest/dev/basic-deliver.html#es-index-rotation) in the *Amazon Kinesis Data Firehose Developer Guide* .
* @property processingConfiguration The data processing configuration for the Kinesis Data Firehose delivery stream.
* @property retryOptions The retry behavior when Kinesis Data Firehose is unable to deliver data to Amazon ES.
* @property roleArn The Amazon Resource Name (ARN) of the IAM role to be assumed by Kinesis Data Firehose for calling the Amazon ES Configuration API and for indexing documents. For more information, see [Controlling Access with Amazon Kinesis Data Firehose](https://docs.aws.amazon.com/firehose/latest/dev/controlling-access.html) .
* @property s3BackupMode The condition under which Kinesis Data Firehose delivers data to Amazon Simple Storage Service (Amazon S3). You can send Amazon S3 all documents (all data) or only the documents that Kinesis Data Firehose could not deliver to the Amazon ES destination. For more information and valid values, see the `S3BackupMode` content for the [ElasticsearchDestinationConfiguration](https://docs.aws.amazon.com/firehose/latest/APIReference/API_ElasticsearchDestinationConfiguration.html) data type in the *Amazon Kinesis Data Firehose API Reference* .
* @property s3Configuration The S3 bucket where Kinesis Data Firehose backs up incoming data.
* @property typeName The Elasticsearch type name that Amazon ES adds to documents when indexing data.
* @property vpcConfiguration The details of the VPC of the Amazon ES destination.
*/
public data class DeliveryStreamElasticsearchDestinationConfiguration(
public val bufferingHints: DeliveryStreamElasticsearchBufferingHints? = null,
public val cloudWatchLoggingOptions: DeliveryStreamCloudWatchLoggingOptions? = null,
public val clusterEndpoint: String? = null,
public val documentIdOptions: DeliveryStreamDocumentIdOptions? = null,
public val domainArn: String? = null,
public val indexName: String,
public val indexRotationPeriod: DeliveryStreamElasticsearchDestinationConfigurationIndexRotationPeriod? = null,
public val processingConfiguration: DeliveryStreamProcessingConfiguration? = null,
public val retryOptions: DeliveryStreamElasticsearchRetryOptions? = null,
public val roleArn: String,
public val s3BackupMode: DeliveryStreamElasticsearchDestinationConfigurationS3BackupMode? = null,
public val s3Configuration: DeliveryStreamS3DestinationConfiguration,
public val typeName: String? = null,
public val vpcConfiguration: DeliveryStreamVpcConfiguration? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.kinesisfirehose.outputs.DeliveryStreamElasticsearchDestinationConfiguration): DeliveryStreamElasticsearchDestinationConfiguration =
DeliveryStreamElasticsearchDestinationConfiguration(
bufferingHints = javaType.bufferingHints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamElasticsearchBufferingHints.Companion.toKotlin(args0)
})
}).orElse(null),
cloudWatchLoggingOptions = javaType.cloudWatchLoggingOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamCloudWatchLoggingOptions.Companion.toKotlin(args0)
})
}).orElse(null),
clusterEndpoint = javaType.clusterEndpoint().map({ args0 -> args0 }).orElse(null),
documentIdOptions = javaType.documentIdOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamDocumentIdOptions.Companion.toKotlin(args0)
})
}).orElse(null),
domainArn = javaType.domainArn().map({ args0 -> args0 }).orElse(null),
indexName = javaType.indexName(),
indexRotationPeriod = javaType.indexRotationPeriod().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamElasticsearchDestinationConfigurationIndexRotationPeriod.Companion.toKotlin(args0)
})
}).orElse(null),
processingConfiguration = javaType.processingConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamProcessingConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
retryOptions = javaType.retryOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamElasticsearchRetryOptions.Companion.toKotlin(args0)
})
}).orElse(null),
roleArn = javaType.roleArn(),
s3BackupMode = javaType.s3BackupMode().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.enums.DeliveryStreamElasticsearchDestinationConfigurationS3BackupMode.Companion.toKotlin(args0)
})
}).orElse(null),
s3Configuration = javaType.s3Configuration().let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamS3DestinationConfiguration.Companion.toKotlin(args0)
}),
typeName = javaType.typeName().map({ args0 -> args0 }).orElse(null),
vpcConfiguration = javaType.vpcConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kinesisfirehose.kotlin.outputs.DeliveryStreamVpcConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy