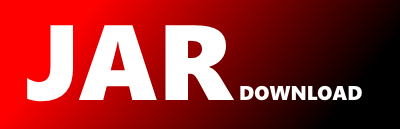
com.pulumi.awsnative.kms.kotlin.KmsFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.kms.kotlin
import com.pulumi.awsnative.kms.KmsFunctions.getAliasPlain
import com.pulumi.awsnative.kms.KmsFunctions.getKeyPlain
import com.pulumi.awsnative.kms.KmsFunctions.getReplicaKeyPlain
import com.pulumi.awsnative.kms.kotlin.inputs.GetAliasPlainArgs
import com.pulumi.awsnative.kms.kotlin.inputs.GetAliasPlainArgsBuilder
import com.pulumi.awsnative.kms.kotlin.inputs.GetKeyPlainArgs
import com.pulumi.awsnative.kms.kotlin.inputs.GetKeyPlainArgsBuilder
import com.pulumi.awsnative.kms.kotlin.inputs.GetReplicaKeyPlainArgs
import com.pulumi.awsnative.kms.kotlin.inputs.GetReplicaKeyPlainArgsBuilder
import com.pulumi.awsnative.kms.kotlin.outputs.GetAliasResult
import com.pulumi.awsnative.kms.kotlin.outputs.GetKeyResult
import com.pulumi.awsnative.kms.kotlin.outputs.GetReplicaKeyResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.kms.kotlin.outputs.GetAliasResult.Companion.toKotlin as getAliasResultToKotlin
import com.pulumi.awsnative.kms.kotlin.outputs.GetKeyResult.Companion.toKotlin as getKeyResultToKotlin
import com.pulumi.awsnative.kms.kotlin.outputs.GetReplicaKeyResult.Companion.toKotlin as getReplicaKeyResultToKotlin
public object KmsFunctions {
/**
* The ``AWS::KMS::Alias`` resource specifies a display name for a [KMS key](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#kms_keys). You can use an alias to identify a KMS key in the KMS console, in the [DescribeKey](https://docs.aws.amazon.com/kms/latest/APIReference/API_DescribeKey.html) operation, and in [cryptographic operations](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#cryptographic-operations), such as [Decrypt](https://docs.aws.amazon.com/kms/latest/APIReference/API_Decrypt.html) and [GenerateDataKey](https://docs.aws.amazon.com/kms/latest/APIReference/API_GenerateDataKey.html).
* Adding, deleting, or updating an alias can allow or deny permission to the KMS key. For details, see [ABAC for](https://docs.aws.amazon.com/kms/latest/developerguide/abac.html) in the *Developer Guide*.
* Using an alias to refer to a KMS key can help you simplify key management. For example, an alias in your code can be associated with different KMS keys in different AWS-Regions. For more information, see [Using aliases](https://docs.aws.amazon.com/kms/latest/developerguide/kms-alias.html) in the *Developer Guide*.
* When specifying an alias, observe the following rules.
* + Each alias is associated with one KMS key, but multiple aliases can be associated with the same KMS key.
* + The alias and its associated KMS key must be in the same AWS-account and Region.
* + The alias name must be unique in the AWS-account and Region. However, you can create aliases with the same name in different AWS-Regions. For example, you can have an ``alias/projectKey`` in multiple Regions, each of which is associated with a KMS key in its Region.
* + Each alias name must begin with ``alias/`` followed by a name, such as ``alias/exampleKey``. The alias name can contain only alphanumeric characters, forward slashes (/), underscores (_), and dashes (-). Alias names cannot begin with ``alias/aws/``. That alias name prefix is reserved for [](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#aws-managed-cmk).
* *Regions*
* KMS CloudFormation resources are available in all AWS-Regions in which KMS and CFN are supported.
* @param argument null
* @return null
*/
public suspend fun getAlias(argument: GetAliasPlainArgs): GetAliasResult =
getAliasResultToKotlin(getAliasPlain(argument.toJava()).await())
/**
* @see [getAlias].
* @param aliasName Specifies the alias name. This value must begin with ``alias/`` followed by a name, such as ``alias/ExampleAlias``.
* If you change the value of the ``AliasName`` property, the existing alias is deleted and a new alias is created for the specified KMS key. This change can disrupt applications that use the alias. It can also allow or deny access to a KMS key affected by attribute-based access control (ABAC).
* The alias must be string of 1-256 characters. It can contain only alphanumeric characters, forward slashes (/), underscores (_), and dashes (-). The alias name cannot begin with ``alias/aws/``. The ``alias/aws/`` prefix is reserved for [](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#aws-managed-cmk).
* @return null
*/
public suspend fun getAlias(aliasName: String): GetAliasResult {
val argument = GetAliasPlainArgs(
aliasName = aliasName,
)
return getAliasResultToKotlin(getAliasPlain(argument.toJava()).await())
}
/**
* @see [getAlias].
* @param argument Builder for [com.pulumi.awsnative.kms.kotlin.inputs.GetAliasPlainArgs].
* @return null
*/
public suspend fun getAlias(argument: suspend GetAliasPlainArgsBuilder.() -> Unit): GetAliasResult {
val builder = GetAliasPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAliasResultToKotlin(getAliasPlain(builtArgument.toJava()).await())
}
/**
* The ``AWS::KMS::Key`` resource specifies an [KMS key](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#kms_keys) in KMSlong. You can use this resource to create symmetric encryption KMS keys, asymmetric KMS keys for encryption or signing, and symmetric HMAC KMS keys. You can use ``AWS::KMS::Key`` to create [multi-Region primary keys](https://docs.aws.amazon.com/kms/latest/developerguide/multi-region-keys-overview.html#mrk-primary-key) of all supported types. To replicate a multi-Region key, use the ``AWS::KMS::ReplicaKey`` resource.
* If you change the value of the ``KeySpec``, ``KeyUsage``, ``Origin``, or ``MultiRegion`` properties of an existing KMS key, the update request fails, regardless of the value of the [UpdateReplacePolicy attribute](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-attribute-updatereplacepolicy.html). This prevents you from accidentally deleting a KMS key by changing any of its immutable property values.
* KMS replaced the term *customer master key (CMK)* with ** and *KMS key*. The concept has not changed. To prevent breaking changes, KMS is keeping some variations of this term.
* You can use symmetric encryption KMS keys to encrypt and decrypt small amounts of data, but they are more commonly used to generate data keys and data key pairs. You can also use a symmetric encryption KMS key to encrypt data stored in AWS services that are [integrated with](https://docs.aws.amazon.com//kms/features/#AWS_Service_Integration). For more information, see [Symmetric encryption KMS keys](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#symmetric-cmks) in the *Developer Guide*.
* You can use asymmetric KMS keys to encrypt and decrypt data or sign messages and verify signatures. To create an asymmetric key, you must specify an asymmetric ``KeySpec`` value and a ``KeyUsage`` value. For details, see [Asymmetric keys in](https://docs.aws.amazon.com/kms/latest/developerguide/symmetric-asymmetric.html) in the *Developer Guide*.
* You can use HMAC KMS keys (which are also symmetric keys) to generate and verify hash-based message authentication codes. To create an HMAC key, you must specify an HMAC ``KeySpec`` value and a ``KeyUsage`` value of ``GENERATE_VERIFY_MAC``. For details, see [HMAC keys in](https://docs.aws.amazon.com/kms/latest/developerguide/hmac.html) in the *Developer Guide*.
* You can also create symmetric encryption, asymmetric, and HMAC multi-Region primary keys. To create a multi-Region primary key, set the ``MultiRegion`` property to ``true``. For information about multi-Region keys, see [Multi-Region keys in](https://docs.aws.amazon.com/kms/latest/developerguide/multi-region-keys-overview.html) in the *Developer Guide*.
* You cannot use the ``AWS::KMS::Key`` resource to specify a KMS key with [imported key material](https://docs.aws.amazon.com/kms/latest/developerguide/importing-keys.html) or a KMS key in a [custom key store](https://docs.aws.amazon.com/kms/latest/developerguide/custom-key-store-overview.html).
* *Regions*
* KMS CloudFormation resources are available in all Regions in which KMS and CFN are supported. You can use the ``AWS::KMS::Key`` resource to create and manage all KMS key types that are supported in a Region.
* @param argument null
* @return null
*/
public suspend fun getKey(argument: GetKeyPlainArgs): GetKeyResult =
getKeyResultToKotlin(getKeyPlain(argument.toJava()).await())
/**
* @see [getKey].
* @param keyId The key ID of the KMS key, such as `1234abcd-12ab-34cd-56ef-1234567890ab` .
* For information about the key ID of a KMS key, see [Key ID](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#key-id-key-id) in the *AWS Key Management Service Developer Guide* .
* @return null
*/
public suspend fun getKey(keyId: String): GetKeyResult {
val argument = GetKeyPlainArgs(
keyId = keyId,
)
return getKeyResultToKotlin(getKeyPlain(argument.toJava()).await())
}
/**
* @see [getKey].
* @param argument Builder for [com.pulumi.awsnative.kms.kotlin.inputs.GetKeyPlainArgs].
* @return null
*/
public suspend fun getKey(argument: suspend GetKeyPlainArgsBuilder.() -> Unit): GetKeyResult {
val builder = GetKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getKeyResultToKotlin(getKeyPlain(builtArgument.toJava()).await())
}
/**
* The AWS::KMS::ReplicaKey resource specifies a multi-region replica AWS KMS key in AWS Key Management Service (AWS KMS).
* @param argument null
* @return null
*/
public suspend fun getReplicaKey(argument: GetReplicaKeyPlainArgs): GetReplicaKeyResult =
getReplicaKeyResultToKotlin(getReplicaKeyPlain(argument.toJava()).await())
/**
* @see [getReplicaKey].
* @param keyId The key ID of the replica key, such as `mrk-1234abcd12ab34cd56ef1234567890ab` .
* Related multi-Region keys have the same key ID. For information about the key IDs of multi-Region keys, see [How multi-Region keys work](https://docs.aws.amazon.com/kms/latest/developerguide/multi-region-keys-overview.html#mrk-how-it-works) in the *AWS Key Management Service Developer Guide* .
* @return null
*/
public suspend fun getReplicaKey(keyId: String): GetReplicaKeyResult {
val argument = GetReplicaKeyPlainArgs(
keyId = keyId,
)
return getReplicaKeyResultToKotlin(getReplicaKeyPlain(argument.toJava()).await())
}
/**
* @see [getReplicaKey].
* @param argument Builder for [com.pulumi.awsnative.kms.kotlin.inputs.GetReplicaKeyPlainArgs].
* @return null
*/
public suspend fun getReplicaKey(argument: suspend GetReplicaKeyPlainArgsBuilder.() -> Unit): GetReplicaKeyResult {
val builder = GetReplicaKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getReplicaKeyResultToKotlin(getReplicaKeyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy