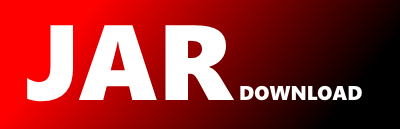
com.pulumi.awsnative.lex.kotlin.inputs.BotLocaleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lex.kotlin.inputs
import com.pulumi.awsnative.lex.inputs.BotLocaleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A locale in the bot, which contains the intents and slot types that the bot uses in conversations with users in the specified language and locale.
* @property customVocabulary Specifies a custom vocabulary to use with a specific locale.
* @property description A description of the bot locale. Use this to help identify the bot locale in lists.
* @property intents List of intents
* @property localeId The identifier of the language and locale that the bot will be used in. The string must match one of the supported locales.
* @property nluConfidenceThreshold Determines the threshold where Amazon Lex will insert the `AMAZON.FallbackIntent` , `AMAZON.KendraSearchIntent` , or both when returning alternative intents. You must configure an `AMAZON.FallbackIntent` . `AMAZON.KendraSearchIntent` is only inserted if it is configured for the bot.
* @property slotTypes List of SlotTypes
* @property voiceSettings Defines settings for using an Amazon Polly voice to communicate with a user.
*/
public data class BotLocaleArgs(
public val customVocabulary: Output? = null,
public val description: Output? = null,
public val intents: Output>? = null,
public val localeId: Output,
public val nluConfidenceThreshold: Output,
public val slotTypes: Output>? = null,
public val voiceSettings: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lex.inputs.BotLocaleArgs =
com.pulumi.awsnative.lex.inputs.BotLocaleArgs.builder()
.customVocabulary(customVocabulary?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.intents(
intents?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.localeId(localeId.applyValue({ args0 -> args0 }))
.nluConfidenceThreshold(nluConfidenceThreshold.applyValue({ args0 -> args0 }))
.slotTypes(
slotTypes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.voiceSettings(voiceSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [BotLocaleArgs].
*/
@PulumiTagMarker
public class BotLocaleArgsBuilder internal constructor() {
private var customVocabulary: Output? = null
private var description: Output? = null
private var intents: Output>? = null
private var localeId: Output? = null
private var nluConfidenceThreshold: Output? = null
private var slotTypes: Output>? = null
private var voiceSettings: Output? = null
/**
* @param value Specifies a custom vocabulary to use with a specific locale.
*/
@JvmName("drqwwqhntxkrwjvr")
public suspend fun customVocabulary(`value`: Output) {
this.customVocabulary = value
}
/**
* @param value A description of the bot locale. Use this to help identify the bot locale in lists.
*/
@JvmName("aiowcbxllwjfdusl")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value List of intents
*/
@JvmName("khawtawyefybxlnn")
public suspend fun intents(`value`: Output>) {
this.intents = value
}
@JvmName("kqlrgmsqumcduvlu")
public suspend fun intents(vararg values: Output) {
this.intents = Output.all(values.asList())
}
/**
* @param values List of intents
*/
@JvmName("eyfeasvyypkipgcs")
public suspend fun intents(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy