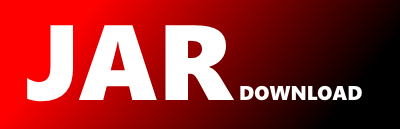
com.pulumi.awsnative.lightsail.kotlin.inputs.DatabaseRelationalDatabaseParameterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.lightsail.kotlin.inputs
import com.pulumi.awsnative.lightsail.inputs.DatabaseRelationalDatabaseParameterArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Describes the parameters of the database.
* @property allowedValues Specifies the valid range of values for the parameter.
* @property applyMethod Indicates when parameter updates are applied. Can be immediate or pending-reboot.
* @property applyType Specifies the engine-specific parameter type.
* @property dataType Specifies the valid data type for the parameter.
* @property description Provides a description of the parameter.
* @property isModifiable A Boolean value indicating whether the parameter can be modified.
* @property parameterName Specifies the name of the parameter.
* @property parameterValue Specifies the value of the parameter.
*/
public data class DatabaseRelationalDatabaseParameterArgs(
public val allowedValues: Output? = null,
public val applyMethod: Output? = null,
public val applyType: Output? = null,
public val dataType: Output? = null,
public val description: Output? = null,
public val isModifiable: Output? = null,
public val parameterName: Output? = null,
public val parameterValue: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.lightsail.inputs.DatabaseRelationalDatabaseParameterArgs =
com.pulumi.awsnative.lightsail.inputs.DatabaseRelationalDatabaseParameterArgs.builder()
.allowedValues(allowedValues?.applyValue({ args0 -> args0 }))
.applyMethod(applyMethod?.applyValue({ args0 -> args0 }))
.applyType(applyType?.applyValue({ args0 -> args0 }))
.dataType(dataType?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.isModifiable(isModifiable?.applyValue({ args0 -> args0 }))
.parameterName(parameterName?.applyValue({ args0 -> args0 }))
.parameterValue(parameterValue?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabaseRelationalDatabaseParameterArgs].
*/
@PulumiTagMarker
public class DatabaseRelationalDatabaseParameterArgsBuilder internal constructor() {
private var allowedValues: Output? = null
private var applyMethod: Output? = null
private var applyType: Output? = null
private var dataType: Output? = null
private var description: Output? = null
private var isModifiable: Output? = null
private var parameterName: Output? = null
private var parameterValue: Output? = null
/**
* @param value Specifies the valid range of values for the parameter.
*/
@JvmName("ipbrobefvofbduwf")
public suspend fun allowedValues(`value`: Output) {
this.allowedValues = value
}
/**
* @param value Indicates when parameter updates are applied. Can be immediate or pending-reboot.
*/
@JvmName("nksxthxlprnxioax")
public suspend fun applyMethod(`value`: Output) {
this.applyMethod = value
}
/**
* @param value Specifies the engine-specific parameter type.
*/
@JvmName("gyvvlstjeygmfxiw")
public suspend fun applyType(`value`: Output) {
this.applyType = value
}
/**
* @param value Specifies the valid data type for the parameter.
*/
@JvmName("adydbnlexbokeaqt")
public suspend fun dataType(`value`: Output) {
this.dataType = value
}
/**
* @param value Provides a description of the parameter.
*/
@JvmName("ddemxlkuskfwqqlh")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A Boolean value indicating whether the parameter can be modified.
*/
@JvmName("eaygnhubnylxrhxd")
public suspend fun isModifiable(`value`: Output) {
this.isModifiable = value
}
/**
* @param value Specifies the name of the parameter.
*/
@JvmName("lvnabtcwojiqlkyc")
public suspend fun parameterName(`value`: Output) {
this.parameterName = value
}
/**
* @param value Specifies the value of the parameter.
*/
@JvmName("ibeovjeufwfwjwle")
public suspend fun parameterValue(`value`: Output) {
this.parameterValue = value
}
/**
* @param value Specifies the valid range of values for the parameter.
*/
@JvmName("bkvrwomgpphvptqc")
public suspend fun allowedValues(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowedValues = mapped
}
/**
* @param value Indicates when parameter updates are applied. Can be immediate or pending-reboot.
*/
@JvmName("kseepnkeciymuwon")
public suspend fun applyMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applyMethod = mapped
}
/**
* @param value Specifies the engine-specific parameter type.
*/
@JvmName("btapupjjpshdwqfh")
public suspend fun applyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applyType = mapped
}
/**
* @param value Specifies the valid data type for the parameter.
*/
@JvmName("tbjwttprbxoxiqgm")
public suspend fun dataType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataType = mapped
}
/**
* @param value Provides a description of the parameter.
*/
@JvmName("jerjbrpxbiomogrd")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value A Boolean value indicating whether the parameter can be modified.
*/
@JvmName("lcnilaegdhaqchfl")
public suspend fun isModifiable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isModifiable = mapped
}
/**
* @param value Specifies the name of the parameter.
*/
@JvmName("xqpgreeqjelefeqw")
public suspend fun parameterName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parameterName = mapped
}
/**
* @param value Specifies the value of the parameter.
*/
@JvmName("vrfrtehwsmjmbhfd")
public suspend fun parameterValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parameterValue = mapped
}
internal fun build(): DatabaseRelationalDatabaseParameterArgs =
DatabaseRelationalDatabaseParameterArgs(
allowedValues = allowedValues,
applyMethod = applyMethod,
applyType = applyType,
dataType = dataType,
description = description,
isModifiable = isModifiable,
parameterName = parameterName,
parameterValue = parameterValue,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy