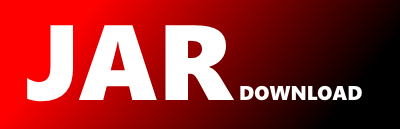
com.pulumi.awsnative.mediaconnect.kotlin.inputs.FlowFailoverConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.mediaconnect.kotlin.inputs
import com.pulumi.awsnative.mediaconnect.inputs.FlowFailoverConfigArgs.builder
import com.pulumi.awsnative.mediaconnect.kotlin.enums.FlowFailoverConfigFailoverMode
import com.pulumi.awsnative.mediaconnect.kotlin.enums.FlowFailoverConfigState
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The settings for source failover
* @property failoverMode The type of failover you choose for this flow. MERGE combines the source streams into a single stream, allowing graceful recovery from any single-source loss. FAILOVER allows switching between different streams.
* @property recoveryWindow Search window time to look for dash-7 packets
* @property sourcePriority The priority you want to assign to a source. You can have a primary stream and a backup stream or two equally prioritized streams.
* @property state The state of source failover on the flow. If the state is inactive, the flow can have only one source. If the state is active, the flow can have one or two sources.
*/
public data class FlowFailoverConfigArgs(
public val failoverMode: Output? = null,
public val recoveryWindow: Output? = null,
public val sourcePriority: Output? = null,
public val state: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.mediaconnect.inputs.FlowFailoverConfigArgs =
com.pulumi.awsnative.mediaconnect.inputs.FlowFailoverConfigArgs.builder()
.failoverMode(failoverMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.recoveryWindow(recoveryWindow?.applyValue({ args0 -> args0 }))
.sourcePriority(sourcePriority?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.state(state?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FlowFailoverConfigArgs].
*/
@PulumiTagMarker
public class FlowFailoverConfigArgsBuilder internal constructor() {
private var failoverMode: Output? = null
private var recoveryWindow: Output? = null
private var sourcePriority: Output? = null
private var state: Output? = null
/**
* @param value The type of failover you choose for this flow. MERGE combines the source streams into a single stream, allowing graceful recovery from any single-source loss. FAILOVER allows switching between different streams.
*/
@JvmName("mrqoydqxnoqwcpaj")
public suspend fun failoverMode(`value`: Output) {
this.failoverMode = value
}
/**
* @param value Search window time to look for dash-7 packets
*/
@JvmName("vfmfcpkqkrptbwfd")
public suspend fun recoveryWindow(`value`: Output) {
this.recoveryWindow = value
}
/**
* @param value The priority you want to assign to a source. You can have a primary stream and a backup stream or two equally prioritized streams.
*/
@JvmName("wgsyqtfynegvywup")
public suspend fun sourcePriority(`value`: Output) {
this.sourcePriority = value
}
/**
* @param value The state of source failover on the flow. If the state is inactive, the flow can have only one source. If the state is active, the flow can have one or two sources.
*/
@JvmName("mdypdfbmudabvvgk")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value The type of failover you choose for this flow. MERGE combines the source streams into a single stream, allowing graceful recovery from any single-source loss. FAILOVER allows switching between different streams.
*/
@JvmName("uwtmyviagjleaavt")
public suspend fun failoverMode(`value`: FlowFailoverConfigFailoverMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failoverMode = mapped
}
/**
* @param value Search window time to look for dash-7 packets
*/
@JvmName("pgfpjgkadmqtcrin")
public suspend fun recoveryWindow(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoveryWindow = mapped
}
/**
* @param value The priority you want to assign to a source. You can have a primary stream and a backup stream or two equally prioritized streams.
*/
@JvmName("aaucykiculfhtsix")
public suspend fun sourcePriority(`value`: FlowFailoverConfigSourcePriorityPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourcePriority = mapped
}
/**
* @param argument The priority you want to assign to a source. You can have a primary stream and a backup stream or two equally prioritized streams.
*/
@JvmName("bsifcncubwhqnwan")
public suspend fun sourcePriority(argument: suspend FlowFailoverConfigSourcePriorityPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = FlowFailoverConfigSourcePriorityPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sourcePriority = mapped
}
/**
* @param value The state of source failover on the flow. If the state is inactive, the flow can have only one source. If the state is active, the flow can have one or two sources.
*/
@JvmName("nlfsnvbpnkwelber")
public suspend fun state(`value`: FlowFailoverConfigState?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): FlowFailoverConfigArgs = FlowFailoverConfigArgs(
failoverMode = failoverMode,
recoveryWindow = recoveryWindow,
sourcePriority = sourcePriority,
state = state,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy