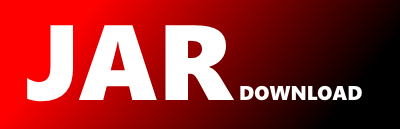
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointDashPackage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.mediapackage.kotlin.outputs
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointAdsOnDeliveryRestrictions
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageAdTriggersItem
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageManifestLayout
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackagePeriodTriggersItem
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageProfile
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageSegmentTemplateFormat
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageUtcTiming
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A Dynamic Adaptive Streaming over HTTP (DASH) packaging configuration.
* @property adTriggers A list of SCTE-35 message types that are treated as ad markers in the output. If empty, no ad markers are output. Specify multiple items to create ad markers for all of the included message types.
* @property adsOnDeliveryRestrictions The flags on SCTE-35 segmentation descriptors that have to be present for AWS Elemental MediaPackage to insert ad markers in the output manifest. For information about SCTE-35 in AWS Elemental MediaPackage , see [SCTE-35 Message Options in AWS Elemental MediaPackage](https://docs.aws.amazon.com/mediapackage/latest/ug/scte.html) .
* @property encryption Parameters for encrypting content.
* @property includeIframeOnlyStream When enabled, an I-Frame only stream will be included in the output.
* @property manifestLayout Determines the position of some tags in the Media Presentation Description (MPD). When set to FULL, elements like SegmentTemplate and ContentProtection are included in each Representation. When set to COMPACT, duplicate elements are combined and presented at the AdaptationSet level.
* @property manifestWindowSeconds Time window (in seconds) contained in each manifest.
* @property minBufferTimeSeconds Minimum duration (in seconds) that a player will buffer media before starting the presentation.
* @property minUpdatePeriodSeconds Minimum duration (in seconds) between potential changes to the Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD).
* @property periodTriggers A list of triggers that controls when the outgoing Dynamic Adaptive Streaming over HTTP (DASH) Media Presentation Description (MPD) will be partitioned into multiple periods. If empty, the content will not be partitioned into more than one period. If the list contains "ADS", new periods will be created where the Channel source contains SCTE-35 ad markers.
* @property profile The Dynamic Adaptive Streaming over HTTP (DASH) profile type. When set to "HBBTV_1_5", HbbTV 1.5 compliant output is enabled.
* @property segmentDurationSeconds Duration (in seconds) of each segment. Actual segments will be rounded to the nearest multiple of the source segment duration.
* @property segmentTemplateFormat Determines the type of SegmentTemplate included in the Media Presentation Description (MPD). When set to NUMBER_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Number$ media URLs. When set to TIME_WITH_TIMELINE, a full timeline is presented in each SegmentTemplate, with $Time$ media URLs. When set to NUMBER_WITH_DURATION, only a duration is included in each SegmentTemplate, with $Number$ media URLs.
* @property streamSelection Limitations for outputs from the endpoint, based on the video bitrate.
* @property suggestedPresentationDelaySeconds Duration (in seconds) to delay live content before presentation.
* @property utcTiming Determines the type of UTCTiming included in the Media Presentation Description (MPD)
* @property utcTimingUri Specifies the value attribute of the UTCTiming field when utcTiming is set to HTTP-ISO, HTTP-HEAD or HTTP-XSDATE
*/
public data class OriginEndpointDashPackage(
public val adTriggers: List? = null,
public val adsOnDeliveryRestrictions: OriginEndpointAdsOnDeliveryRestrictions? = null,
public val encryption: OriginEndpointDashEncryption? = null,
public val includeIframeOnlyStream: Boolean? = null,
public val manifestLayout: OriginEndpointDashPackageManifestLayout? = null,
public val manifestWindowSeconds: Int? = null,
public val minBufferTimeSeconds: Int? = null,
public val minUpdatePeriodSeconds: Int? = null,
public val periodTriggers: List? = null,
public val profile: OriginEndpointDashPackageProfile? = null,
public val segmentDurationSeconds: Int? = null,
public val segmentTemplateFormat: OriginEndpointDashPackageSegmentTemplateFormat? = null,
public val streamSelection: OriginEndpointStreamSelection? = null,
public val suggestedPresentationDelaySeconds: Int? = null,
public val utcTiming: OriginEndpointDashPackageUtcTiming? = null,
public val utcTimingUri: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.mediapackage.outputs.OriginEndpointDashPackage): OriginEndpointDashPackage = OriginEndpointDashPackage(
adTriggers = javaType.adTriggers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageAdTriggersItem.Companion.toKotlin(args0)
})
}),
adsOnDeliveryRestrictions = javaType.adsOnDeliveryRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointAdsOnDeliveryRestrictions.Companion.toKotlin(args0)
})
}).orElse(null),
encryption = javaType.encryption().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointDashEncryption.Companion.toKotlin(args0)
})
}).orElse(null),
includeIframeOnlyStream = javaType.includeIframeOnlyStream().map({ args0 -> args0 }).orElse(null),
manifestLayout = javaType.manifestLayout().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageManifestLayout.Companion.toKotlin(args0)
})
}).orElse(null),
manifestWindowSeconds = javaType.manifestWindowSeconds().map({ args0 -> args0 }).orElse(null),
minBufferTimeSeconds = javaType.minBufferTimeSeconds().map({ args0 -> args0 }).orElse(null),
minUpdatePeriodSeconds = javaType.minUpdatePeriodSeconds().map({ args0 -> args0 }).orElse(null),
periodTriggers = javaType.periodTriggers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackagePeriodTriggersItem.Companion.toKotlin(args0)
})
}),
profile = javaType.profile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageProfile.Companion.toKotlin(args0)
})
}).orElse(null),
segmentDurationSeconds = javaType.segmentDurationSeconds().map({ args0 -> args0 }).orElse(null),
segmentTemplateFormat = javaType.segmentTemplateFormat().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageSegmentTemplateFormat.Companion.toKotlin(args0)
})
}).orElse(null),
streamSelection = javaType.streamSelection().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointStreamSelection.Companion.toKotlin(args0)
})
}).orElse(null),
suggestedPresentationDelaySeconds = javaType.suggestedPresentationDelaySeconds().map({ args0 ->
args0
}).orElse(null),
utcTiming = javaType.utcTiming().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointDashPackageUtcTiming.Companion.toKotlin(args0)
})
}).orElse(null),
utcTimingUri = javaType.utcTimingUri().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy