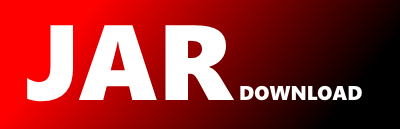
com.pulumi.awsnative.neptune.kotlin.DbCluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.neptune.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.neptune.kotlin.outputs.DbClusterDbClusterRole
import com.pulumi.awsnative.neptune.kotlin.outputs.DbClusterServerlessScalingConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.neptune.kotlin.outputs.DbClusterDbClusterRole.Companion.toKotlin as dbClusterDbClusterRoleToKotlin
import com.pulumi.awsnative.neptune.kotlin.outputs.DbClusterServerlessScalingConfiguration.Companion.toKotlin as dbClusterServerlessScalingConfigurationToKotlin
/**
* Builder for [DbCluster].
*/
@PulumiTagMarker
public class DbClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: DbClusterArgs = DbClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DbClusterArgsBuilder.() -> Unit) {
val builder = DbClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): DbCluster {
val builtJavaResource = com.pulumi.awsnative.neptune.DbCluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return DbCluster(builtJavaResource)
}
}
/**
* The AWS::Neptune::DBCluster resource creates an Amazon Neptune DB cluster.
*/
public class DbCluster internal constructor(
override val javaResource: com.pulumi.awsnative.neptune.DbCluster,
) : KotlinCustomResource(javaResource, DbClusterMapper) {
/**
* Provides a list of the AWS Identity and Access Management (IAM) roles that are associated with the DB cluster. IAM roles that are associated with a DB cluster grant permission for the DB cluster to access other AWS services on your behalf.
*/
public val associatedRoles: Output>?
get() = javaResource.associatedRoles().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
dbClusterDbClusterRoleToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Provides the list of EC2 Availability Zones that instances in the DB cluster can be created in.
*/
public val availabilityZones: Output>?
get() = javaResource.availabilityZones().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Specifies the number of days for which automatic DB snapshots are retained.
*/
public val backupRetentionPeriod: Output?
get() = javaResource.backupRetentionPeriod().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The resource id for the DB cluster. For example: `cluster-ABCD1234EFGH5678IJKL90MNOP`. The cluster ID uniquely identifies the cluster and is used in things like IAM authentication policies.
*/
public val clusterResourceId: Output
get() = javaResource.clusterResourceId().applyValue({ args0 -> args0 })
/**
* A value that indicates whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default behaviour is not to copy them.
*/
public val copyTagsToSnapshot: Output?
get() = javaResource.copyTagsToSnapshot().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The DB cluster identifier. Contains a user-supplied DB cluster identifier. This identifier is the unique key that identifies a DB cluster stored as a lowercase string.
*/
public val dbClusterIdentifier: Output?
get() = javaResource.dbClusterIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Provides the name of the DB cluster parameter group.
*/
public val dbClusterParameterGroupName: Output?
get() = javaResource.dbClusterParameterGroupName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the DB parameter group to apply to all instances of the DB cluster. Used only in case of a major EngineVersion upgrade request.
*/
public val dbInstanceParameterGroupName: Output?
get() = javaResource.dbInstanceParameterGroupName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The port number on which the DB instances in the DB cluster accept connections.
* If not specified, the default port used is `8182`.
* Note: `Port` property will soon be deprecated from this resource. Please update existing templates to rename it with new property `DBPort` having same functionalities.
*/
public val dbPort: Output?
get() = javaResource.dbPort().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies information on the subnet group associated with the DB cluster, including the name, description, and subnets in the subnet group.
*/
public val dbSubnetGroupName: Output?
get() = javaResource.dbSubnetGroupName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Indicates whether or not the DB cluster has deletion protection enabled. The database can't be deleted when deletion protection is enabled.
*/
public val deletionProtection: Output?
get() = javaResource.deletionProtection().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies a list of log types that are enabled for export to CloudWatch Logs.
*/
public val enableCloudwatchLogsExports: Output>?
get() = javaResource.enableCloudwatchLogsExports().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The connection endpoint for the DB cluster. For example: `mystack-mydbcluster-1apw1j4phylrk.cg034hpkmmjt.us-east-2.rds.amazonaws.com`
*/
public val endpoint: Output
get() = javaResource.endpoint().applyValue({ args0 -> args0 })
/**
* Indicates the database engine version.
*/
public val engineVersion: Output?
get() = javaResource.engineVersion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* True if mapping of Amazon Identity and Access Management (IAM) accounts to database accounts is enabled, and otherwise false.
*/
public val iamAuthEnabled: Output?
get() = javaResource.iamAuthEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If `StorageEncrypted` is true, the Amazon KMS key identifier for the encrypted DB cluster.
*/
public val kmsKeyId: Output?
get() = javaResource.kmsKeyId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The port number on which the DB cluster accepts connections. For example: `8182`.
*/
public val port: Output
get() = javaResource.port().applyValue({ args0 -> args0 })
/**
* Specifies the daily time range during which automated backups are created if automated backups are enabled, as determined by the BackupRetentionPeriod.
*/
public val preferredBackupWindow: Output?
get() = javaResource.preferredBackupWindow().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*/
public val preferredMaintenanceWindow: Output?
get() = javaResource.preferredMaintenanceWindow().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The reader endpoint for the DB cluster. For example: `mystack-mydbcluster-ro-1apw1j4phylrk.cg034hpkmmjt.us-east-2.rds.amazonaws.com`
*/
public val readEndpoint: Output
get() = javaResource.readEndpoint().applyValue({ args0 -> args0 })
/**
* Creates a new DB cluster from a DB snapshot or DB cluster snapshot.
* If a DB snapshot is specified, the target DB cluster is created from the source DB snapshot with a default configuration and default security group.
* If a DB cluster snapshot is specified, the target DB cluster is created from the source DB cluster restore point with the same configuration as the original source DB cluster, except that the new DB cluster is created with the default security group.
*/
public val restoreToTime: Output?
get() = javaResource.restoreToTime().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Creates a new DB cluster from a DB snapshot or DB cluster snapshot.
* If a DB snapshot is specified, the target DB cluster is created from the source DB snapshot with a default configuration and default security group.
* If a DB cluster snapshot is specified, the target DB cluster is created from the source DB cluster restore point with the same configuration as the original source DB cluster, except that the new DB cluster is created with the default security group.
*/
public val restoreType: Output?
get() = javaResource.restoreType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Contains the scaling configuration used by the Neptune Serverless Instances within this DB cluster.
*/
public val serverlessScalingConfiguration: Output?
get() = javaResource.serverlessScalingConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
dbClusterServerlessScalingConfigurationToKotlin(args0)
})
}).orElse(null)
})
/**
* Specifies the identifier for a DB cluster snapshot. Must match the identifier of an existing snapshot.
* After you restore a DB cluster using a SnapshotIdentifier, you must specify the same SnapshotIdentifier for any future updates to the DB cluster. When you specify this property for an update, the DB cluster is not restored from the snapshot again, and the data in the database is not changed.
* However, if you don't specify the SnapshotIdentifier, an empty DB cluster is created, and the original DB cluster is deleted. If you specify a property that is different from the previous snapshot restore property, the DB cluster is restored from the snapshot specified by the SnapshotIdentifier, and the original DB cluster is deleted.
*/
public val snapshotIdentifier: Output?
get() = javaResource.snapshotIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Creates a new DB cluster from a DB snapshot or DB cluster snapshot.
* If a DB snapshot is specified, the target DB cluster is created from the source DB snapshot with a default configuration and default security group.
* If a DB cluster snapshot is specified, the target DB cluster is created from the source DB cluster restore point with the same configuration as the original source DB cluster, except that the new DB cluster is created with the default security group.
*/
public val sourceDbClusterIdentifier: Output?
get() = javaResource.sourceDbClusterIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Indicates whether the DB cluster is encrypted.
* If you specify the `DBClusterIdentifier`, `DBSnapshotIdentifier`, or `SourceDBInstanceIdentifier` property, don't specify this property. The value is inherited from the cluster, snapshot, or source DB instance. If you specify the KmsKeyId property, you must enable encryption.
* If you specify the KmsKeyId, you must enable encryption by setting StorageEncrypted to true.
*/
public val storageEncrypted: Output?
get() = javaResource.storageEncrypted().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The tags assigned to this cluster.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* Creates a new DB cluster from a DB snapshot or DB cluster snapshot.
* If a DB snapshot is specified, the target DB cluster is created from the source DB snapshot with a default configuration and default security group.
* If a DB cluster snapshot is specified, the target DB cluster is created from the source DB cluster restore point with the same configuration as the original source DB cluster, except that the new DB cluster is created with the default security group.
*/
public val useLatestRestorableTime: Output?
get() = javaResource.useLatestRestorableTime().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Provides a list of VPC security groups that the DB cluster belongs to.
*/
public val vpcSecurityGroupIds: Output>?
get() = javaResource.vpcSecurityGroupIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
}
public object DbClusterMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.neptune.DbCluster::class == javaResource::class
override fun map(javaResource: Resource): DbCluster = DbCluster(
javaResource as
com.pulumi.awsnative.neptune.DbCluster,
)
}
/**
* @see [DbCluster].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [DbCluster].
*/
public suspend fun dbCluster(name: String, block: suspend DbClusterResourceBuilder.() -> Unit): DbCluster {
val builder = DbClusterResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [DbCluster].
* @param name The _unique_ name of the resulting resource.
*/
public fun dbCluster(name: String): DbCluster {
val builder = DbClusterResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy