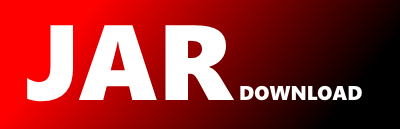
com.pulumi.awsnative.networkfirewall.kotlin.inputs.RuleGroupStatefulRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.networkfirewall.kotlin.inputs
import com.pulumi.awsnative.networkfirewall.inputs.RuleGroupStatefulRuleArgs.builder
import com.pulumi.awsnative.networkfirewall.kotlin.enums.RuleGroupStatefulRuleAction
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property action Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful inspection of the traffic flow.
* The actions for a stateful rule are defined as follows:
* - *PASS* - Permits the packets to go to the intended destination.
* - *DROP* - Blocks the packets from going to the intended destination and sends an alert log message, if alert logging is configured in the `Firewall` `LoggingConfiguration` .
* - *REJECT* - Drops traffic that matches the conditions of the stateful rule and sends a TCP reset packet back to sender of the packet. A TCP reset packet is a packet with no payload and a `RST` bit contained in the TCP header flags. `REJECT` is available only for TCP traffic.
* - *ALERT* - Permits the packets to go to the intended destination and sends an alert log message, if alert logging is configured in the `Firewall` `LoggingConfiguration` .
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with `ALERT` action, verify in the logs that the rule is filtering as you want, then change the action to `DROP` .
* - *REJECT* - Drops TCP traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back to sender of the packet. A TCP reset packet is a packet with no payload and a `RST` bit contained in the TCP header flags. Also sends an alert log mesage if alert logging is configured in the `Firewall` `LoggingConfiguration` .
* `REJECT` isn't currently available for use with IMAP and FTP protocols.
* @property header The stateful inspection criteria for this rule, used to inspect traffic flows.
* @property ruleOptions Additional settings for a stateful rule, provided as keywords and settings.
*/
public data class RuleGroupStatefulRuleArgs(
public val action: Output,
public val `header`: Output,
public val ruleOptions: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.networkfirewall.inputs.RuleGroupStatefulRuleArgs =
com.pulumi.awsnative.networkfirewall.inputs.RuleGroupStatefulRuleArgs.builder()
.action(action.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`header`(`header`.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ruleOptions(
ruleOptions.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RuleGroupStatefulRuleArgs].
*/
@PulumiTagMarker
public class RuleGroupStatefulRuleArgsBuilder internal constructor() {
private var action: Output? = null
private var `header`: Output? = null
private var ruleOptions: Output>? = null
/**
* @param value Defines what Network Firewall should do with the packets in a traffic flow when the flow matches the stateful rule criteria. For all actions, Network Firewall performs the specified action and discontinues stateful inspection of the traffic flow.
* The actions for a stateful rule are defined as follows:
* - *PASS* - Permits the packets to go to the intended destination.
* - *DROP* - Blocks the packets from going to the intended destination and sends an alert log message, if alert logging is configured in the `Firewall` `LoggingConfiguration` .
* - *REJECT* - Drops traffic that matches the conditions of the stateful rule and sends a TCP reset packet back to sender of the packet. A TCP reset packet is a packet with no payload and a `RST` bit contained in the TCP header flags. `REJECT` is available only for TCP traffic.
* - *ALERT* - Permits the packets to go to the intended destination and sends an alert log message, if alert logging is configured in the `Firewall` `LoggingConfiguration` .
* You can use this action to test a rule that you intend to use to drop traffic. You can enable the rule with `ALERT` action, verify in the logs that the rule is filtering as you want, then change the action to `DROP` .
* - *REJECT* - Drops TCP traffic that matches the conditions of the stateful rule, and sends a TCP reset packet back to sender of the packet. A TCP reset packet is a packet with no payload and a `RST` bit contained in the TCP header flags. Also sends an alert log mesage if alert logging is configured in the `Firewall` `LoggingConfiguration` .
* `REJECT` isn't currently available for use with IMAP and FTP protocols.
*/
@JvmName("vkfynbytunqxoidx")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value The stateful inspection criteria for this rule, used to inspect traffic flows.
*/
@JvmName("ouevdllnlteadrjx")
public suspend fun `header`(`value`: Output) {
this.`header` = value
}
/**
* @param value Additional settings for a stateful rule, provided as keywords and settings.
*/
@JvmName("yglgyccpyssgtajm")
public suspend fun ruleOptions(`value`: Output>) {
this.ruleOptions = value
}
@JvmName("faikbgkwatvoumrc")
public suspend fun ruleOptions(vararg values: Output) {
this.ruleOptions = Output.all(values.asList())
}
/**
* @param values Additional settings for a stateful rule, provided as keywords and settings.
*/
@JvmName("cntwixhncwjiqpwf")
public suspend fun ruleOptions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy