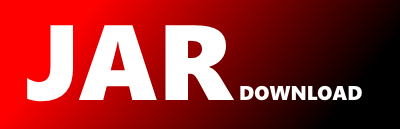
com.pulumi.awsnative.opensearchservice.kotlin.inputs.DomainEbsOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.opensearchservice.kotlin.inputs
import com.pulumi.awsnative.opensearchservice.inputs.DomainEbsOptionsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property ebsEnabled Specifies whether Amazon EBS volumes are attached to data nodes in the OpenSearch Service domain.
* @property iops The number of I/O operations per second (IOPS) that the volume supports. This property applies only to the `gp3` and provisioned IOPS EBS volume types.
* @property throughput The throughput (in MiB/s) of the EBS volumes attached to data nodes. Applies only to the `gp3` volume type.
* @property volumeSize The size (in GiB) of the EBS volume for each data node. The minimum and maximum size of an EBS volume depends on the EBS volume type and the instance type to which it is attached. For more information, see [EBS volume size limits](https://docs.aws.amazon.com/opensearch-service/latest/developerguide/limits.html#ebsresource) in the *Amazon OpenSearch Service Developer Guide* .
* @property volumeType The EBS volume type to use with the OpenSearch Service domain. If you choose `gp3` , you must also specify values for `Iops` and `Throughput` . For more information about each type, see [Amazon EBS volume types](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSVolumeTypes.html) in the *Amazon EC2 User Guide for Linux Instances* .
*/
public data class DomainEbsOptionsArgs(
public val ebsEnabled: Output? = null,
public val iops: Output? = null,
public val throughput: Output? = null,
public val volumeSize: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.opensearchservice.inputs.DomainEbsOptionsArgs =
com.pulumi.awsnative.opensearchservice.inputs.DomainEbsOptionsArgs.builder()
.ebsEnabled(ebsEnabled?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.throughput(throughput?.applyValue({ args0 -> args0 }))
.volumeSize(volumeSize?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DomainEbsOptionsArgs].
*/
@PulumiTagMarker
public class DomainEbsOptionsArgsBuilder internal constructor() {
private var ebsEnabled: Output? = null
private var iops: Output? = null
private var throughput: Output? = null
private var volumeSize: Output? = null
private var volumeType: Output? = null
/**
* @param value Specifies whether Amazon EBS volumes are attached to data nodes in the OpenSearch Service domain.
*/
@JvmName("baaadntxiyeuqlqt")
public suspend fun ebsEnabled(`value`: Output) {
this.ebsEnabled = value
}
/**
* @param value The number of I/O operations per second (IOPS) that the volume supports. This property applies only to the `gp3` and provisioned IOPS EBS volume types.
*/
@JvmName("sxlygudnhnclqcjf")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value The throughput (in MiB/s) of the EBS volumes attached to data nodes. Applies only to the `gp3` volume type.
*/
@JvmName("dyvrvieirgfsojsq")
public suspend fun throughput(`value`: Output) {
this.throughput = value
}
/**
* @param value The size (in GiB) of the EBS volume for each data node. The minimum and maximum size of an EBS volume depends on the EBS volume type and the instance type to which it is attached. For more information, see [EBS volume size limits](https://docs.aws.amazon.com/opensearch-service/latest/developerguide/limits.html#ebsresource) in the *Amazon OpenSearch Service Developer Guide* .
*/
@JvmName("ablxfrvxfjlydgro")
public suspend fun volumeSize(`value`: Output) {
this.volumeSize = value
}
/**
* @param value The EBS volume type to use with the OpenSearch Service domain. If you choose `gp3` , you must also specify values for `Iops` and `Throughput` . For more information about each type, see [Amazon EBS volume types](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSVolumeTypes.html) in the *Amazon EC2 User Guide for Linux Instances* .
*/
@JvmName("ekjbsuykewskbxwh")
public suspend fun volumeType(`value`: Output) {
this.volumeType = value
}
/**
* @param value Specifies whether Amazon EBS volumes are attached to data nodes in the OpenSearch Service domain.
*/
@JvmName("vqoocgmjngejvfsn")
public suspend fun ebsEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ebsEnabled = mapped
}
/**
* @param value The number of I/O operations per second (IOPS) that the volume supports. This property applies only to the `gp3` and provisioned IOPS EBS volume types.
*/
@JvmName("bpewpvycvwlrebkg")
public suspend fun iops(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iops = mapped
}
/**
* @param value The throughput (in MiB/s) of the EBS volumes attached to data nodes. Applies only to the `gp3` volume type.
*/
@JvmName("sfddipxeyfdcqkjj")
public suspend fun throughput(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throughput = mapped
}
/**
* @param value The size (in GiB) of the EBS volume for each data node. The minimum and maximum size of an EBS volume depends on the EBS volume type and the instance type to which it is attached. For more information, see [EBS volume size limits](https://docs.aws.amazon.com/opensearch-service/latest/developerguide/limits.html#ebsresource) in the *Amazon OpenSearch Service Developer Guide* .
*/
@JvmName("omreetwdnhnglnao")
public suspend fun volumeSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeSize = mapped
}
/**
* @param value The EBS volume type to use with the OpenSearch Service domain. If you choose `gp3` , you must also specify values for `Iops` and `Throughput` . For more information about each type, see [Amazon EBS volume types](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EBSVolumeTypes.html) in the *Amazon EC2 User Guide for Linux Instances* .
*/
@JvmName("ndcgssoyvknldtbs")
public suspend fun volumeType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeType = mapped
}
internal fun build(): DomainEbsOptionsArgs = DomainEbsOptionsArgs(
ebsEnabled = ebsEnabled,
iops = iops,
throughput = throughput,
volumeSize = volumeSize,
volumeType = volumeType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy