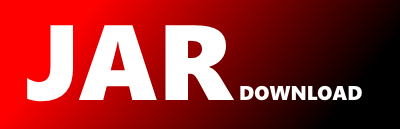
com.pulumi.awsnative.paymentcryptography.kotlin.inputs.KeyModesOfUseArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.paymentcryptography.kotlin.inputs
import com.pulumi.awsnative.paymentcryptography.inputs.KeyModesOfUseArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property decrypt Specifies whether an AWS Payment Cryptography key can be used to decrypt data.
* @property deriveKey Specifies whether an AWS Payment Cryptography key can be used to derive new keys.
* @property encrypt Specifies whether an AWS Payment Cryptography key can be used to encrypt data.
* @property generate Specifies whether an AWS Payment Cryptography key can be used to generate and verify other card and PIN verification keys.
* @property noRestrictions Specifies whether an AWS Payment Cryptography key has no special restrictions other than the restrictions implied by `KeyUsage` .
* @property sign Specifies whether an AWS Payment Cryptography key can be used for signing.
* @property unwrap
* @property verify Specifies whether an AWS Payment Cryptography key can be used to verify signatures.
* @property wrap Specifies whether an AWS Payment Cryptography key can be used to wrap other keys.
*/
public data class KeyModesOfUseArgs(
public val decrypt: Output? = null,
public val deriveKey: Output? = null,
public val encrypt: Output? = null,
public val generate: Output? = null,
public val noRestrictions: Output? = null,
public val sign: Output? = null,
public val unwrap: Output? = null,
public val verify: Output? = null,
public val wrap: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.paymentcryptography.inputs.KeyModesOfUseArgs =
com.pulumi.awsnative.paymentcryptography.inputs.KeyModesOfUseArgs.builder()
.decrypt(decrypt?.applyValue({ args0 -> args0 }))
.deriveKey(deriveKey?.applyValue({ args0 -> args0 }))
.encrypt(encrypt?.applyValue({ args0 -> args0 }))
.generate(generate?.applyValue({ args0 -> args0 }))
.noRestrictions(noRestrictions?.applyValue({ args0 -> args0 }))
.sign(sign?.applyValue({ args0 -> args0 }))
.unwrap(unwrap?.applyValue({ args0 -> args0 }))
.verify(verify?.applyValue({ args0 -> args0 }))
.wrap(wrap?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KeyModesOfUseArgs].
*/
@PulumiTagMarker
public class KeyModesOfUseArgsBuilder internal constructor() {
private var decrypt: Output? = null
private var deriveKey: Output? = null
private var encrypt: Output? = null
private var generate: Output? = null
private var noRestrictions: Output? = null
private var sign: Output? = null
private var unwrap: Output? = null
private var verify: Output? = null
private var wrap: Output? = null
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to decrypt data.
*/
@JvmName("fsvdrqblrhrkhooa")
public suspend fun decrypt(`value`: Output) {
this.decrypt = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to derive new keys.
*/
@JvmName("exevbuhkxsustnkh")
public suspend fun deriveKey(`value`: Output) {
this.deriveKey = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to encrypt data.
*/
@JvmName("gyaxaiximcbuertl")
public suspend fun encrypt(`value`: Output) {
this.encrypt = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to generate and verify other card and PIN verification keys.
*/
@JvmName("tvmmuswevynpxwkq")
public suspend fun generate(`value`: Output) {
this.generate = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key has no special restrictions other than the restrictions implied by `KeyUsage` .
*/
@JvmName("trrgvsxothlymlue")
public suspend fun noRestrictions(`value`: Output) {
this.noRestrictions = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used for signing.
*/
@JvmName("rpfppighrdewwlcx")
public suspend fun sign(`value`: Output) {
this.sign = value
}
/**
* @param value
*/
@JvmName("uuirgvndqhgufhmu")
public suspend fun unwrap(`value`: Output) {
this.unwrap = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to verify signatures.
*/
@JvmName("dtbpfpuoktikvopm")
public suspend fun verify(`value`: Output) {
this.verify = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to wrap other keys.
*/
@JvmName("hhnusuxvapcnbfbd")
public suspend fun wrap(`value`: Output) {
this.wrap = value
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to decrypt data.
*/
@JvmName("xhbstuoodwabayxd")
public suspend fun decrypt(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.decrypt = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to derive new keys.
*/
@JvmName("ilyvohllrwslqxxb")
public suspend fun deriveKey(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deriveKey = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to encrypt data.
*/
@JvmName("xwblhhdaxukarthe")
public suspend fun encrypt(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encrypt = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to generate and verify other card and PIN verification keys.
*/
@JvmName("firlchwaeywqcnbp")
public suspend fun generate(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.generate = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key has no special restrictions other than the restrictions implied by `KeyUsage` .
*/
@JvmName("sqqxfjoqecyxcint")
public suspend fun noRestrictions(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.noRestrictions = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used for signing.
*/
@JvmName("jtktcsdvsqufvlus")
public suspend fun sign(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sign = mapped
}
/**
* @param value
*/
@JvmName("xjsdkeuqfykrmlxs")
public suspend fun unwrap(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.unwrap = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to verify signatures.
*/
@JvmName("gkatjmgtsqftqawq")
public suspend fun verify(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verify = mapped
}
/**
* @param value Specifies whether an AWS Payment Cryptography key can be used to wrap other keys.
*/
@JvmName("tmhsvpyptgvktwsi")
public suspend fun wrap(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wrap = mapped
}
internal fun build(): KeyModesOfUseArgs = KeyModesOfUseArgs(
decrypt = decrypt,
deriveKey = deriveKey,
encrypt = encrypt,
generate = generate,
noRestrictions = noRestrictions,
sign = sign,
unwrap = unwrap,
verify = verify,
wrap = wrap,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy