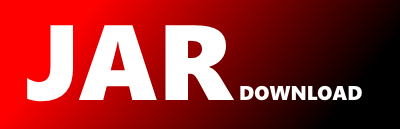
com.pulumi.awsnative.pipes.kotlin.inputs.PipeEcsTaskOverrideArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.pipes.kotlin.inputs
import com.pulumi.awsnative.pipes.inputs.PipeEcsTaskOverrideArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property containerOverrides One or more container overrides that are sent to a task.
* @property cpu The cpu override for the task.
* @property ephemeralStorage The ephemeral storage setting override for the task.
* > This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
* >
* > - Linux platform version `1.4.0` or later.
* > - Windows platform version `1.0.0` or later.
* @property executionRoleArn The Amazon Resource Name (ARN) of the task execution IAM role override for the task. For more information, see [Amazon ECS task execution IAM role](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/task_execution_IAM_role.html) in the *Amazon Elastic Container Service Developer Guide* .
* @property inferenceAcceleratorOverrides The Elastic Inference accelerator override for the task.
* @property memory The memory override for the task.
* @property taskRoleArn The Amazon Resource Name (ARN) of the IAM role that containers in this task can assume. All containers in this task are granted the permissions that are specified in this role. For more information, see [IAM Role for Tasks](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/task-iam-roles.html) in the *Amazon Elastic Container Service Developer Guide* .
*/
public data class PipeEcsTaskOverrideArgs(
public val containerOverrides: Output>? = null,
public val cpu: Output? = null,
public val ephemeralStorage: Output? = null,
public val executionRoleArn: Output? = null,
public val inferenceAcceleratorOverrides: Output>? =
null,
public val memory: Output? = null,
public val taskRoleArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.pipes.inputs.PipeEcsTaskOverrideArgs =
com.pulumi.awsnative.pipes.inputs.PipeEcsTaskOverrideArgs.builder()
.containerOverrides(
containerOverrides?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.cpu(cpu?.applyValue({ args0 -> args0 }))
.ephemeralStorage(ephemeralStorage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.executionRoleArn(executionRoleArn?.applyValue({ args0 -> args0 }))
.inferenceAcceleratorOverrides(
inferenceAcceleratorOverrides?.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.memory(memory?.applyValue({ args0 -> args0 }))
.taskRoleArn(taskRoleArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PipeEcsTaskOverrideArgs].
*/
@PulumiTagMarker
public class PipeEcsTaskOverrideArgsBuilder internal constructor() {
private var containerOverrides: Output>? = null
private var cpu: Output? = null
private var ephemeralStorage: Output? = null
private var executionRoleArn: Output? = null
private var inferenceAcceleratorOverrides: Output>? =
null
private var memory: Output? = null
private var taskRoleArn: Output? = null
/**
* @param value One or more container overrides that are sent to a task.
*/
@JvmName("tafmulnjyjychdgt")
public suspend fun containerOverrides(`value`: Output>) {
this.containerOverrides = value
}
@JvmName("qsdatgvxkuoixcvf")
public suspend fun containerOverrides(vararg values: Output) {
this.containerOverrides = Output.all(values.asList())
}
/**
* @param values One or more container overrides that are sent to a task.
*/
@JvmName("birgrsxrqrivcmcp")
public suspend fun containerOverrides(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy