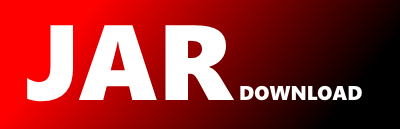
com.pulumi.awsnative.pipes.kotlin.inputs.PipeTargetParametersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.pipes.kotlin.inputs
import com.pulumi.awsnative.pipes.inputs.PipeTargetParametersArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property batchJobParameters The parameters for using an AWS Batch job as a target.
* @property cloudWatchLogsParameters The parameters for using an CloudWatch Logs log stream as a target.
* @property ecsTaskParameters The parameters for using an Amazon ECS task as a target.
* @property eventBridgeEventBusParameters The parameters for using an EventBridge event bus as a target.
* @property httpParameters These are custom parameter to be used when the target is an API Gateway REST APIs or EventBridge ApiDestinations.
* @property inputTemplate Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For more information, see [The JavaScript Object Notation (JSON) Data Interchange Format](https://docs.aws.amazon.com/http://www.rfc-editor.org/rfc/rfc7159.txt) .
* To remove an input template, specify an empty string.
* @property kinesisStreamParameters The parameters for using a Kinesis stream as a target.
* @property lambdaFunctionParameters The parameters for using a Lambda function as a target.
* @property redshiftDataParameters These are custom parameters to be used when the target is a Amazon Redshift cluster to invoke the Amazon Redshift Data API BatchExecuteStatement.
* @property sageMakerPipelineParameters The parameters for using a SageMaker pipeline as a target.
* @property sqsQueueParameters The parameters for using a Amazon SQS stream as a target.
* @property stepFunctionStateMachineParameters The parameters for using a Step Functions state machine as a target.
* @property timestreamParameters The parameters for using a Timestream for LiveAnalytics table as a target.
*/
public data class PipeTargetParametersArgs(
public val batchJobParameters: Output? = null,
public val cloudWatchLogsParameters: Output? = null,
public val ecsTaskParameters: Output? = null,
public val eventBridgeEventBusParameters: Output? =
null,
public val httpParameters: Output? = null,
public val inputTemplate: Output? = null,
public val kinesisStreamParameters: Output? = null,
public val lambdaFunctionParameters: Output? = null,
public val redshiftDataParameters: Output? = null,
public val sageMakerPipelineParameters: Output? = null,
public val sqsQueueParameters: Output? = null,
public val stepFunctionStateMachineParameters: Output? =
null,
public val timestreamParameters: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.pipes.inputs.PipeTargetParametersArgs =
com.pulumi.awsnative.pipes.inputs.PipeTargetParametersArgs.builder()
.batchJobParameters(
batchJobParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.cloudWatchLogsParameters(
cloudWatchLogsParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.ecsTaskParameters(ecsTaskParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.eventBridgeEventBusParameters(
eventBridgeEventBusParameters?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.httpParameters(httpParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.inputTemplate(inputTemplate?.applyValue({ args0 -> args0 }))
.kinesisStreamParameters(
kinesisStreamParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.lambdaFunctionParameters(
lambdaFunctionParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.redshiftDataParameters(
redshiftDataParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sageMakerPipelineParameters(
sageMakerPipelineParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sqsQueueParameters(
sqsQueueParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.stepFunctionStateMachineParameters(
stepFunctionStateMachineParameters?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.timestreamParameters(
timestreamParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [PipeTargetParametersArgs].
*/
@PulumiTagMarker
public class PipeTargetParametersArgsBuilder internal constructor() {
private var batchJobParameters: Output? = null
private var cloudWatchLogsParameters: Output? = null
private var ecsTaskParameters: Output? = null
private var eventBridgeEventBusParameters: Output? =
null
private var httpParameters: Output? = null
private var inputTemplate: Output? = null
private var kinesisStreamParameters: Output? = null
private var lambdaFunctionParameters: Output? = null
private var redshiftDataParameters: Output? = null
private var sageMakerPipelineParameters: Output? = null
private var sqsQueueParameters: Output? = null
private var stepFunctionStateMachineParameters: Output? =
null
private var timestreamParameters: Output? = null
/**
* @param value The parameters for using an AWS Batch job as a target.
*/
@JvmName("hgsldossvuxfnxlx")
public suspend fun batchJobParameters(`value`: Output) {
this.batchJobParameters = value
}
/**
* @param value The parameters for using an CloudWatch Logs log stream as a target.
*/
@JvmName("wprcybluhplkmhbp")
public suspend fun cloudWatchLogsParameters(`value`: Output) {
this.cloudWatchLogsParameters = value
}
/**
* @param value The parameters for using an Amazon ECS task as a target.
*/
@JvmName("sxpsboynvbmceuko")
public suspend fun ecsTaskParameters(`value`: Output) {
this.ecsTaskParameters = value
}
/**
* @param value The parameters for using an EventBridge event bus as a target.
*/
@JvmName("uaqsymjpikoiblfw")
public suspend fun eventBridgeEventBusParameters(`value`: Output) {
this.eventBridgeEventBusParameters = value
}
/**
* @param value These are custom parameter to be used when the target is an API Gateway REST APIs or EventBridge ApiDestinations.
*/
@JvmName("hqhqdqwsjkhitidu")
public suspend fun httpParameters(`value`: Output) {
this.httpParameters = value
}
/**
* @param value Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For more information, see [The JavaScript Object Notation (JSON) Data Interchange Format](https://docs.aws.amazon.com/http://www.rfc-editor.org/rfc/rfc7159.txt) .
* To remove an input template, specify an empty string.
*/
@JvmName("bodoawnnhdghvldh")
public suspend fun inputTemplate(`value`: Output) {
this.inputTemplate = value
}
/**
* @param value The parameters for using a Kinesis stream as a target.
*/
@JvmName("ucawlemxvsqdeksg")
public suspend fun kinesisStreamParameters(`value`: Output) {
this.kinesisStreamParameters = value
}
/**
* @param value The parameters for using a Lambda function as a target.
*/
@JvmName("njjdkcpmswyttrjt")
public suspend fun lambdaFunctionParameters(`value`: Output) {
this.lambdaFunctionParameters = value
}
/**
* @param value These are custom parameters to be used when the target is a Amazon Redshift cluster to invoke the Amazon Redshift Data API BatchExecuteStatement.
*/
@JvmName("kofjnkfxyldoasyh")
public suspend fun redshiftDataParameters(`value`: Output) {
this.redshiftDataParameters = value
}
/**
* @param value The parameters for using a SageMaker pipeline as a target.
*/
@JvmName("nguexsvuorggpyak")
public suspend fun sageMakerPipelineParameters(`value`: Output) {
this.sageMakerPipelineParameters = value
}
/**
* @param value The parameters for using a Amazon SQS stream as a target.
*/
@JvmName("oexkpsurakdqkowx")
public suspend fun sqsQueueParameters(`value`: Output) {
this.sqsQueueParameters = value
}
/**
* @param value The parameters for using a Step Functions state machine as a target.
*/
@JvmName("jxksaugbevqxnkch")
public suspend fun stepFunctionStateMachineParameters(`value`: Output) {
this.stepFunctionStateMachineParameters = value
}
/**
* @param value The parameters for using a Timestream for LiveAnalytics table as a target.
*/
@JvmName("cyacblvbdefmxpid")
public suspend fun timestreamParameters(`value`: Output) {
this.timestreamParameters = value
}
/**
* @param value The parameters for using an AWS Batch job as a target.
*/
@JvmName("amhxyyesaovpwkwt")
public suspend fun batchJobParameters(`value`: PipeTargetBatchJobParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.batchJobParameters = mapped
}
/**
* @param argument The parameters for using an AWS Batch job as a target.
*/
@JvmName("oxpxdttrqbtngxbi")
public suspend fun batchJobParameters(argument: suspend PipeTargetBatchJobParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetBatchJobParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.batchJobParameters = mapped
}
/**
* @param value The parameters for using an CloudWatch Logs log stream as a target.
*/
@JvmName("ckrrecijlucuymjc")
public suspend fun cloudWatchLogsParameters(`value`: PipeTargetCloudWatchLogsParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudWatchLogsParameters = mapped
}
/**
* @param argument The parameters for using an CloudWatch Logs log stream as a target.
*/
@JvmName("mghjifydbyqdlrgl")
public suspend fun cloudWatchLogsParameters(argument: suspend PipeTargetCloudWatchLogsParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetCloudWatchLogsParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudWatchLogsParameters = mapped
}
/**
* @param value The parameters for using an Amazon ECS task as a target.
*/
@JvmName("mleifmiqmffvbtqu")
public suspend fun ecsTaskParameters(`value`: PipeTargetEcsTaskParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ecsTaskParameters = mapped
}
/**
* @param argument The parameters for using an Amazon ECS task as a target.
*/
@JvmName("eflqogjhfqitrtvs")
public suspend fun ecsTaskParameters(argument: suspend PipeTargetEcsTaskParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetEcsTaskParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ecsTaskParameters = mapped
}
/**
* @param value The parameters for using an EventBridge event bus as a target.
*/
@JvmName("mjyfqggrjebbmqun")
public suspend fun eventBridgeEventBusParameters(`value`: PipeTargetEventBridgeEventBusParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventBridgeEventBusParameters = mapped
}
/**
* @param argument The parameters for using an EventBridge event bus as a target.
*/
@JvmName("qbbgasheikevkelv")
public suspend fun eventBridgeEventBusParameters(argument: suspend PipeTargetEventBridgeEventBusParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetEventBridgeEventBusParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.eventBridgeEventBusParameters = mapped
}
/**
* @param value These are custom parameter to be used when the target is an API Gateway REST APIs or EventBridge ApiDestinations.
*/
@JvmName("patidlmteidqrtbi")
public suspend fun httpParameters(`value`: PipeTargetHttpParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpParameters = mapped
}
/**
* @param argument These are custom parameter to be used when the target is an API Gateway REST APIs or EventBridge ApiDestinations.
*/
@JvmName("cewhfsyfhsbhgirm")
public suspend fun httpParameters(argument: suspend PipeTargetHttpParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetHttpParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.httpParameters = mapped
}
/**
* @param value Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For more information, see [The JavaScript Object Notation (JSON) Data Interchange Format](https://docs.aws.amazon.com/http://www.rfc-editor.org/rfc/rfc7159.txt) .
* To remove an input template, specify an empty string.
*/
@JvmName("qxkfqffgjxsknxeq")
public suspend fun inputTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inputTemplate = mapped
}
/**
* @param value The parameters for using a Kinesis stream as a target.
*/
@JvmName("gyfwjtgcqwtfcmdm")
public suspend fun kinesisStreamParameters(`value`: PipeTargetKinesisStreamParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisStreamParameters = mapped
}
/**
* @param argument The parameters for using a Kinesis stream as a target.
*/
@JvmName("mvqnjmsearspmpnl")
public suspend fun kinesisStreamParameters(argument: suspend PipeTargetKinesisStreamParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetKinesisStreamParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kinesisStreamParameters = mapped
}
/**
* @param value The parameters for using a Lambda function as a target.
*/
@JvmName("blxwaevopptfpwup")
public suspend fun lambdaFunctionParameters(`value`: PipeTargetLambdaFunctionParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lambdaFunctionParameters = mapped
}
/**
* @param argument The parameters for using a Lambda function as a target.
*/
@JvmName("ijenvamsvorjtmno")
public suspend fun lambdaFunctionParameters(argument: suspend PipeTargetLambdaFunctionParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetLambdaFunctionParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.lambdaFunctionParameters = mapped
}
/**
* @param value These are custom parameters to be used when the target is a Amazon Redshift cluster to invoke the Amazon Redshift Data API BatchExecuteStatement.
*/
@JvmName("rchgqodfkwaeeowk")
public suspend fun redshiftDataParameters(`value`: PipeTargetRedshiftDataParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redshiftDataParameters = mapped
}
/**
* @param argument These are custom parameters to be used when the target is a Amazon Redshift cluster to invoke the Amazon Redshift Data API BatchExecuteStatement.
*/
@JvmName("plysrbpjbqowhqtb")
public suspend fun redshiftDataParameters(argument: suspend PipeTargetRedshiftDataParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetRedshiftDataParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.redshiftDataParameters = mapped
}
/**
* @param value The parameters for using a SageMaker pipeline as a target.
*/
@JvmName("iggilmrlshiktrsf")
public suspend fun sageMakerPipelineParameters(`value`: PipeTargetSageMakerPipelineParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sageMakerPipelineParameters = mapped
}
/**
* @param argument The parameters for using a SageMaker pipeline as a target.
*/
@JvmName("svjehpqlegycoons")
public suspend fun sageMakerPipelineParameters(argument: suspend PipeTargetSageMakerPipelineParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetSageMakerPipelineParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sageMakerPipelineParameters = mapped
}
/**
* @param value The parameters for using a Amazon SQS stream as a target.
*/
@JvmName("asgcoiumgfqgvruv")
public suspend fun sqsQueueParameters(`value`: PipeTargetSqsQueueParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqsQueueParameters = mapped
}
/**
* @param argument The parameters for using a Amazon SQS stream as a target.
*/
@JvmName("fhupcxicawkilrno")
public suspend fun sqsQueueParameters(argument: suspend PipeTargetSqsQueueParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetSqsQueueParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sqsQueueParameters = mapped
}
/**
* @param value The parameters for using a Step Functions state machine as a target.
*/
@JvmName("lpqjnfxmmmenukhp")
public suspend fun stepFunctionStateMachineParameters(`value`: PipeTargetStateMachineParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stepFunctionStateMachineParameters = mapped
}
/**
* @param argument The parameters for using a Step Functions state machine as a target.
*/
@JvmName("luteetckqwgixwax")
public suspend fun stepFunctionStateMachineParameters(argument: suspend PipeTargetStateMachineParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetStateMachineParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.stepFunctionStateMachineParameters = mapped
}
/**
* @param value The parameters for using a Timestream for LiveAnalytics table as a target.
*/
@JvmName("dlmjnbsmrfveweis")
public suspend fun timestreamParameters(`value`: PipeTargetTimestreamParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timestreamParameters = mapped
}
/**
* @param argument The parameters for using a Timestream for LiveAnalytics table as a target.
*/
@JvmName("oherwnrqvbrtcyur")
public suspend fun timestreamParameters(argument: suspend PipeTargetTimestreamParametersArgsBuilder.() -> Unit) {
val toBeMapped = PipeTargetTimestreamParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.timestreamParameters = mapped
}
internal fun build(): PipeTargetParametersArgs = PipeTargetParametersArgs(
batchJobParameters = batchJobParameters,
cloudWatchLogsParameters = cloudWatchLogsParameters,
ecsTaskParameters = ecsTaskParameters,
eventBridgeEventBusParameters = eventBridgeEventBusParameters,
httpParameters = httpParameters,
inputTemplate = inputTemplate,
kinesisStreamParameters = kinesisStreamParameters,
lambdaFunctionParameters = lambdaFunctionParameters,
redshiftDataParameters = redshiftDataParameters,
sageMakerPipelineParameters = sageMakerPipelineParameters,
sqsQueueParameters = sqsQueueParameters,
stepFunctionStateMachineParameters = stepFunctionStateMachineParameters,
timestreamParameters = timestreamParameters,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy