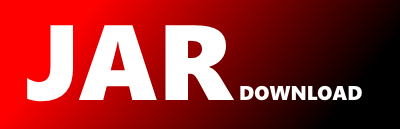
com.pulumi.awsnative.qbusiness.kotlin.Application.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.qbusiness.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.qbusiness.kotlin.enums.ApplicationStatus
import com.pulumi.awsnative.qbusiness.kotlin.outputs.ApplicationAttachmentsConfiguration
import com.pulumi.awsnative.qbusiness.kotlin.outputs.ApplicationEncryptionConfiguration
import com.pulumi.awsnative.qbusiness.kotlin.outputs.ApplicationQAppsConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.enums.ApplicationStatus.Companion.toKotlin as applicationStatusToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.outputs.ApplicationAttachmentsConfiguration.Companion.toKotlin as applicationAttachmentsConfigurationToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.outputs.ApplicationEncryptionConfiguration.Companion.toKotlin as applicationEncryptionConfigurationToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.outputs.ApplicationQAppsConfiguration.Companion.toKotlin as applicationQAppsConfigurationToKotlin
/**
* Builder for [Application].
*/
@PulumiTagMarker
public class ApplicationResourceBuilder internal constructor() {
public var name: String? = null
public var args: ApplicationArgs = ApplicationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ApplicationArgsBuilder.() -> Unit) {
val builder = ApplicationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Application {
val builtJavaResource = com.pulumi.awsnative.qbusiness.Application(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Application(builtJavaResource)
}
}
/**
* Definition of AWS::QBusiness::Application Resource Type
*/
public class Application internal constructor(
override val javaResource: com.pulumi.awsnative.qbusiness.Application,
) : KotlinCustomResource(javaResource, ApplicationMapper) {
/**
* The Amazon Resource Name (ARN) of the Amazon Q Business application.
*/
public val applicationArn: Output
get() = javaResource.applicationArn().applyValue({ args0 -> args0 })
/**
* The identifier for the Amazon Q Business application.
*/
public val applicationId: Output
get() = javaResource.applicationId().applyValue({ args0 -> args0 })
/**
* Configuration information for the file upload during chat feature.
*/
public val attachmentsConfiguration: Output?
get() = javaResource.attachmentsConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> applicationAttachmentsConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* The Unix timestamp when the Amazon Q Business application was created.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* A description for the Amazon Q Business application.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the Amazon Q Business application.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Provides the identifier of the AWS KMS key used to encrypt data indexed by Amazon Q Business. Amazon Q Business doesn't support asymmetric keys.
*/
public val encryptionConfiguration: Output?
get() = javaResource.encryptionConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> applicationEncryptionConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) of the AWS IAM Identity Center instance attached to your Amazon Q Business application.
*/
public val identityCenterApplicationArn: Output
get() = javaResource.identityCenterApplicationArn().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of the IAM Identity Center instance you are either creating for—or connecting to—your Amazon Q Business application.
* *Required* : `Yes`
*/
public val identityCenterInstanceArn: Output?
get() = javaResource.identityCenterInstanceArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configuration information about Amazon Q Apps. (preview feature)
*/
public val qAppsConfiguration: Output?
get() = javaResource.qAppsConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> applicationQAppsConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) of an IAM role with permissions to access your Amazon CloudWatch logs and metrics.
*/
public val roleArn: Output?
get() = javaResource.roleArn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The status of the Amazon Q Business application. The application is ready to use when the status is `ACTIVE` .
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 ->
args0.let({ args0 ->
applicationStatusToKotlin(args0)
})
})
/**
* A list of key-value pairs that identify or categorize your Amazon Q Business application. You can also use tags to help control access to the application. Tag keys and values can consist of Unicode letters, digits, white space, and any of the following symbols: _ . : / = + - @.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The Unix timestamp when the Amazon Q Business application was last updated.
*/
public val updatedAt: Output
get() = javaResource.updatedAt().applyValue({ args0 -> args0 })
}
public object ApplicationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.qbusiness.Application::class == javaResource::class
override fun map(javaResource: Resource): Application = Application(
javaResource as
com.pulumi.awsnative.qbusiness.Application,
)
}
/**
* @see [Application].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Application].
*/
public suspend fun application(name: String, block: suspend ApplicationResourceBuilder.() -> Unit): Application {
val builder = ApplicationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Application].
* @param name The _unique_ name of the resulting resource.
*/
public fun application(name: String): Application {
val builder = ApplicationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy