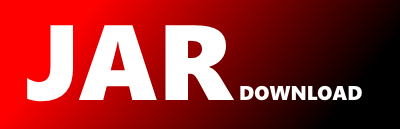
com.pulumi.awsnative.qbusiness.kotlin.outputs.GetDataSourceResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.qbusiness.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.qbusiness.kotlin.enums.DataSourceStatus
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property configuration Configuration information to connect your data source repository to Amazon Q Business. Use this parameter to provide a JSON schema with configuration information specific to your data source connector.
* Each data source has a JSON schema provided by Amazon Q Business that you must use. For example, the Amazon S3 and Web Crawler connectors require the following JSON schemas:
* - [Amazon S3 JSON schema](https://docs.aws.amazon.com/amazonq/latest/qbusiness-ug/s3-api.html)
* - [Web Crawler JSON schema](https://docs.aws.amazon.com/amazonq/latest/qbusiness-ug/web-crawler-api.html)
* You can find configuration templates for your specific data source using the following steps:
* - Navigate to the [Supported connectors](https://docs.aws.amazon.com/amazonq/latest/business-use-dg/connectors-list.html) page in the Amazon Q Business User Guide, and select the data source of your choice.
* - Then, from your specific data source connector page, select *Using the API* . You will find the JSON schema for your data source, including parameter descriptions, in this section.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::QBusiness::DataSource` for more information about the expected schema for this property.
* @property createdAt The Unix timestamp when the Amazon Q Business data source was created.
* @property dataSourceArn The Amazon Resource Name (ARN) of a data source in an Amazon Q Business application.
* @property dataSourceId The identifier of the Amazon Q Business data source.
* @property description A description for the data source connector.
* @property displayName The name of the Amazon Q Business data source.
* @property documentEnrichmentConfiguration Provides the configuration information for altering document metadata and content during the document ingestion process.
* For more information, see [Custom document enrichment](https://docs.aws.amazon.com/amazonq/latest/business-use-dg/custom-document-enrichment.html) .
* @property roleArn The Amazon Resource Name (ARN) of an IAM role with permission to access the data source and required resources.
* @property status The status of the Amazon Q Business data source.
* @property syncSchedule Sets the frequency for Amazon Q Business to check the documents in your data source repository and update your index. If you don't set a schedule, Amazon Q Business won't periodically update the index.
* Specify a `cron-` format schedule string or an empty string to indicate that the index is updated on demand. You can't specify the `Schedule` parameter when the `Type` parameter is set to `CUSTOM` . If you do, you receive a `ValidationException` exception.
* @property tags A list of key-value pairs that identify or categorize the data source connector. You can also use tags to help control access to the data source connector. Tag keys and values can consist of Unicode letters, digits, white space, and any of the following symbols: _ . : / = + - @.
* @property type The type of the Amazon Q Business data source.
* @property updatedAt The Unix timestamp when the Amazon Q Business data source was last updated.
* @property vpcConfiguration Configuration information for an Amazon VPC (Virtual Private Cloud) to connect to your data source. For more information, see [Using Amazon VPC with Amazon Q Business connectors](https://docs.aws.amazon.com/amazonq/latest/business-use-dg/connector-vpc.html) .
*/
public data class GetDataSourceResult(
public val configuration: Any? = null,
public val createdAt: String? = null,
public val dataSourceArn: String? = null,
public val dataSourceId: String? = null,
public val description: String? = null,
public val displayName: String? = null,
public val documentEnrichmentConfiguration: DataSourceDocumentEnrichmentConfiguration? = null,
public val roleArn: String? = null,
public val status: DataSourceStatus? = null,
public val syncSchedule: String? = null,
public val tags: List? = null,
public val type: String? = null,
public val updatedAt: String? = null,
public val vpcConfiguration: DataSourceVpcConfiguration? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.qbusiness.outputs.GetDataSourceResult): GetDataSourceResult = GetDataSourceResult(
configuration = javaType.configuration().map({ args0 -> args0 }).orElse(null),
createdAt = javaType.createdAt().map({ args0 -> args0 }).orElse(null),
dataSourceArn = javaType.dataSourceArn().map({ args0 -> args0 }).orElse(null),
dataSourceId = javaType.dataSourceId().map({ args0 -> args0 }).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
displayName = javaType.displayName().map({ args0 -> args0 }).orElse(null),
documentEnrichmentConfiguration = javaType.documentEnrichmentConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.qbusiness.kotlin.outputs.DataSourceDocumentEnrichmentConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
roleArn = javaType.roleArn().map({ args0 -> args0 }).orElse(null),
status = javaType.status().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.qbusiness.kotlin.enums.DataSourceStatus.Companion.toKotlin(args0)
})
}).orElse(null),
syncSchedule = javaType.syncSchedule().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
type = javaType.type().map({ args0 -> args0 }).orElse(null),
updatedAt = javaType.updatedAt().map({ args0 -> args0 }).orElse(null),
vpcConfiguration = javaType.vpcConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.qbusiness.kotlin.outputs.DataSourceVpcConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy