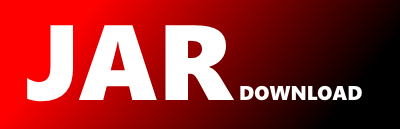
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisWordCloudOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisWordCloudOptionsArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisWordCloudCloudLayout
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisWordCloudWordCasing
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisWordCloudWordOrientation
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisWordCloudWordPadding
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisWordCloudWordScaling
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property cloudLayout The cloud layout options (fluid, normal) of a word cloud.
* @property maximumStringLength The length limit of each word from 1-100.
* @property wordCasing The word casing options (lower_case, existing_case) for the words in a word cloud.
* @property wordOrientation The word orientation options (horizontal, horizontal_and_vertical) for the words in a word cloud.
* @property wordPadding The word padding options (none, small, medium, large) for the words in a word cloud.
* @property wordScaling The word scaling options (emphasize, normal) for the words in a word cloud.
*/
public data class AnalysisWordCloudOptionsArgs(
public val cloudLayout: Output? = null,
public val maximumStringLength: Output? = null,
public val wordCasing: Output? = null,
public val wordOrientation: Output? = null,
public val wordPadding: Output? = null,
public val wordScaling: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisWordCloudOptionsArgs =
com.pulumi.awsnative.quicksight.inputs.AnalysisWordCloudOptionsArgs.builder()
.cloudLayout(cloudLayout?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maximumStringLength(maximumStringLength?.applyValue({ args0 -> args0 }))
.wordCasing(wordCasing?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.wordOrientation(wordOrientation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.wordPadding(wordPadding?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.wordScaling(wordScaling?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AnalysisWordCloudOptionsArgs].
*/
@PulumiTagMarker
public class AnalysisWordCloudOptionsArgsBuilder internal constructor() {
private var cloudLayout: Output? = null
private var maximumStringLength: Output? = null
private var wordCasing: Output? = null
private var wordOrientation: Output? = null
private var wordPadding: Output? = null
private var wordScaling: Output? = null
/**
* @param value The cloud layout options (fluid, normal) of a word cloud.
*/
@JvmName("djnaefbxgderwmnt")
public suspend fun cloudLayout(`value`: Output) {
this.cloudLayout = value
}
/**
* @param value The length limit of each word from 1-100.
*/
@JvmName("usvioajxvybrscpp")
public suspend fun maximumStringLength(`value`: Output) {
this.maximumStringLength = value
}
/**
* @param value The word casing options (lower_case, existing_case) for the words in a word cloud.
*/
@JvmName("jhhyyihwoehtjclc")
public suspend fun wordCasing(`value`: Output) {
this.wordCasing = value
}
/**
* @param value The word orientation options (horizontal, horizontal_and_vertical) for the words in a word cloud.
*/
@JvmName("qirwbtxeurxwyqfl")
public suspend fun wordOrientation(`value`: Output) {
this.wordOrientation = value
}
/**
* @param value The word padding options (none, small, medium, large) for the words in a word cloud.
*/
@JvmName("ipowokqddahhkgkq")
public suspend fun wordPadding(`value`: Output) {
this.wordPadding = value
}
/**
* @param value The word scaling options (emphasize, normal) for the words in a word cloud.
*/
@JvmName("utdxrkpoilqnkkod")
public suspend fun wordScaling(`value`: Output) {
this.wordScaling = value
}
/**
* @param value The cloud layout options (fluid, normal) of a word cloud.
*/
@JvmName("cbrfhlrpbxxyfosu")
public suspend fun cloudLayout(`value`: AnalysisWordCloudCloudLayout?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudLayout = mapped
}
/**
* @param value The length limit of each word from 1-100.
*/
@JvmName("pjnlyntncadorufc")
public suspend fun maximumStringLength(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumStringLength = mapped
}
/**
* @param value The word casing options (lower_case, existing_case) for the words in a word cloud.
*/
@JvmName("foshdfqhnsuadwpv")
public suspend fun wordCasing(`value`: AnalysisWordCloudWordCasing?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wordCasing = mapped
}
/**
* @param value The word orientation options (horizontal, horizontal_and_vertical) for the words in a word cloud.
*/
@JvmName("qxddhlqovomrumdr")
public suspend fun wordOrientation(`value`: AnalysisWordCloudWordOrientation?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wordOrientation = mapped
}
/**
* @param value The word padding options (none, small, medium, large) for the words in a word cloud.
*/
@JvmName("psciduqdpbjmejay")
public suspend fun wordPadding(`value`: AnalysisWordCloudWordPadding?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wordPadding = mapped
}
/**
* @param value The word scaling options (emphasize, normal) for the words in a word cloud.
*/
@JvmName("vfuckubcewqbmuqr")
public suspend fun wordScaling(`value`: AnalysisWordCloudWordScaling?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wordScaling = mapped
}
internal fun build(): AnalysisWordCloudOptionsArgs = AnalysisWordCloudOptionsArgs(
cloudLayout = cloudLayout,
maximumStringLength = maximumStringLength,
wordCasing = wordCasing,
wordOrientation = wordOrientation,
wordPadding = wordPadding,
wordScaling = wordScaling,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy