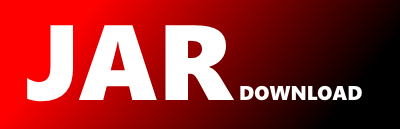
com.pulumi.awsnative.quicksight.kotlin.inputs.DashboardGeospatialMapConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.DashboardGeospatialMapConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property fieldWells The field wells of the visual.
* @property legend The legend display setup of the visual.
* @property mapStyleOptions The map style options of the geospatial map.
* @property pointStyleOptions The point style options of the geospatial map.
* @property tooltip The tooltip display setup of the visual.
* @property visualPalette
* @property windowOptions The window options of the geospatial map.
*/
public data class DashboardGeospatialMapConfigurationArgs(
public val fieldWells: Output? = null,
public val legend: Output? = null,
public val mapStyleOptions: Output? = null,
public val pointStyleOptions: Output? = null,
public val tooltip: Output? = null,
public val visualPalette: Output? = null,
public val windowOptions: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.DashboardGeospatialMapConfigurationArgs =
com.pulumi.awsnative.quicksight.inputs.DashboardGeospatialMapConfigurationArgs.builder()
.fieldWells(fieldWells?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.legend(legend?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.mapStyleOptions(mapStyleOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.pointStyleOptions(pointStyleOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tooltip(tooltip?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.visualPalette(visualPalette?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.windowOptions(windowOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DashboardGeospatialMapConfigurationArgs].
*/
@PulumiTagMarker
public class DashboardGeospatialMapConfigurationArgsBuilder internal constructor() {
private var fieldWells: Output? = null
private var legend: Output? = null
private var mapStyleOptions: Output? = null
private var pointStyleOptions: Output? = null
private var tooltip: Output? = null
private var visualPalette: Output? = null
private var windowOptions: Output? = null
/**
* @param value The field wells of the visual.
*/
@JvmName("xvuhplmpyygdvrif")
public suspend fun fieldWells(`value`: Output) {
this.fieldWells = value
}
/**
* @param value The legend display setup of the visual.
*/
@JvmName("tfrvrxjmadedpuec")
public suspend fun legend(`value`: Output) {
this.legend = value
}
/**
* @param value The map style options of the geospatial map.
*/
@JvmName("pmihtbkjjlmjhjrq")
public suspend fun mapStyleOptions(`value`: Output) {
this.mapStyleOptions = value
}
/**
* @param value The point style options of the geospatial map.
*/
@JvmName("bupppoqdexxljdph")
public suspend fun pointStyleOptions(`value`: Output) {
this.pointStyleOptions = value
}
/**
* @param value The tooltip display setup of the visual.
*/
@JvmName("yrvwguusfwgkodvn")
public suspend fun tooltip(`value`: Output) {
this.tooltip = value
}
/**
* @param value
*/
@JvmName("hgelmpsyirnwsikv")
public suspend fun visualPalette(`value`: Output) {
this.visualPalette = value
}
/**
* @param value The window options of the geospatial map.
*/
@JvmName("hclbayrobleqoqsu")
public suspend fun windowOptions(`value`: Output) {
this.windowOptions = value
}
/**
* @param value The field wells of the visual.
*/
@JvmName("dviugqcrrngdjfmo")
public suspend fun fieldWells(`value`: DashboardGeospatialMapFieldWellsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldWells = mapped
}
/**
* @param argument The field wells of the visual.
*/
@JvmName("olwjwylceautroji")
public suspend fun fieldWells(argument: suspend DashboardGeospatialMapFieldWellsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardGeospatialMapFieldWellsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.fieldWells = mapped
}
/**
* @param value The legend display setup of the visual.
*/
@JvmName("omdmdjtmdcdvbviv")
public suspend fun legend(`value`: DashboardLegendOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.legend = mapped
}
/**
* @param argument The legend display setup of the visual.
*/
@JvmName("qhmfsdduvrwsxdnw")
public suspend fun legend(argument: suspend DashboardLegendOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardLegendOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.legend = mapped
}
/**
* @param value The map style options of the geospatial map.
*/
@JvmName("echxgftfokijbhjm")
public suspend fun mapStyleOptions(`value`: DashboardGeospatialMapStyleOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mapStyleOptions = mapped
}
/**
* @param argument The map style options of the geospatial map.
*/
@JvmName("iqjduedexurbemlj")
public suspend fun mapStyleOptions(argument: suspend DashboardGeospatialMapStyleOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardGeospatialMapStyleOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.mapStyleOptions = mapped
}
/**
* @param value The point style options of the geospatial map.
*/
@JvmName("vumenfkelugniiat")
public suspend fun pointStyleOptions(`value`: DashboardGeospatialPointStyleOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pointStyleOptions = mapped
}
/**
* @param argument The point style options of the geospatial map.
*/
@JvmName("mgriblelxtpfeeuu")
public suspend fun pointStyleOptions(argument: suspend DashboardGeospatialPointStyleOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardGeospatialPointStyleOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pointStyleOptions = mapped
}
/**
* @param value The tooltip display setup of the visual.
*/
@JvmName("qajsyhnynagsobsj")
public suspend fun tooltip(`value`: DashboardTooltipOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tooltip = mapped
}
/**
* @param argument The tooltip display setup of the visual.
*/
@JvmName("sxfdhohugivmaule")
public suspend fun tooltip(argument: suspend DashboardTooltipOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardTooltipOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.tooltip = mapped
}
/**
* @param value
*/
@JvmName("ifcmskceetmafkop")
public suspend fun visualPalette(`value`: DashboardVisualPaletteArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visualPalette = mapped
}
/**
* @param argument
*/
@JvmName("bblwyfujgkcjokln")
public suspend fun visualPalette(argument: suspend DashboardVisualPaletteArgsBuilder.() -> Unit) {
val toBeMapped = DashboardVisualPaletteArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.visualPalette = mapped
}
/**
* @param value The window options of the geospatial map.
*/
@JvmName("xantsmyxqcbugotg")
public suspend fun windowOptions(`value`: DashboardGeospatialWindowOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.windowOptions = mapped
}
/**
* @param argument The window options of the geospatial map.
*/
@JvmName("cxaqueojpkyhevob")
public suspend fun windowOptions(argument: suspend DashboardGeospatialWindowOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardGeospatialWindowOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.windowOptions = mapped
}
internal fun build(): DashboardGeospatialMapConfigurationArgs =
DashboardGeospatialMapConfigurationArgs(
fieldWells = fieldWells,
legend = legend,
mapStyleOptions = mapStyleOptions,
pointStyleOptions = pointStyleOptions,
tooltip = tooltip,
visualPalette = visualPalette,
windowOptions = windowOptions,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy