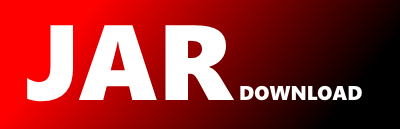
com.pulumi.awsnative.quicksight.kotlin.inputs.DashboardParametersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.DashboardParametersArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A list of Amazon QuickSight parameters and the list's override values.
* @property dateTimeParameters The parameters that have a data type of date-time.
* @property decimalParameters The parameters that have a data type of decimal.
* @property integerParameters The parameters that have a data type of integer.
* @property stringParameters The parameters that have a data type of string.
*/
public data class DashboardParametersArgs(
public val dateTimeParameters: Output>? = null,
public val decimalParameters: Output>? = null,
public val integerParameters: Output>? = null,
public val stringParameters: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.DashboardParametersArgs =
com.pulumi.awsnative.quicksight.inputs.DashboardParametersArgs.builder()
.dateTimeParameters(
dateTimeParameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.decimalParameters(
decimalParameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.integerParameters(
integerParameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.stringParameters(
stringParameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [DashboardParametersArgs].
*/
@PulumiTagMarker
public class DashboardParametersArgsBuilder internal constructor() {
private var dateTimeParameters: Output>? = null
private var decimalParameters: Output>? = null
private var integerParameters: Output>? = null
private var stringParameters: Output>? = null
/**
* @param value The parameters that have a data type of date-time.
*/
@JvmName("obnktoahprjlamdb")
public suspend fun dateTimeParameters(`value`: Output>) {
this.dateTimeParameters = value
}
@JvmName("jsyifixwombygqdo")
public suspend fun dateTimeParameters(vararg values: Output) {
this.dateTimeParameters = Output.all(values.asList())
}
/**
* @param values The parameters that have a data type of date-time.
*/
@JvmName("kcwdkkvfbrpvhjlw")
public suspend fun dateTimeParameters(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy