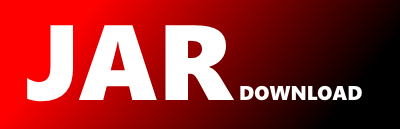
com.pulumi.awsnative.quicksight.kotlin.inputs.DashboardPivotTableOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.DashboardPivotTableOptionsArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.DashboardPivotTableMetricPlacement
import com.pulumi.awsnative.quicksight.kotlin.enums.DashboardPivotTableRowsLayout
import com.pulumi.awsnative.quicksight.kotlin.enums.DashboardVisibility
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cellStyle The table cell style of cells.
* @property collapsedRowDimensionsVisibility The visibility setting of a pivot table's collapsed row dimension fields. If the value of this structure is `HIDDEN` , all collapsed columns in a pivot table are automatically hidden. The default value is `VISIBLE` .
* @property columnHeaderStyle The table cell style of the column header.
* @property columnNamesVisibility The visibility of the column names.
* @property defaultCellWidth String based length that is composed of value and unit in px
* @property metricPlacement The metric placement (row, column) options.
* @property rowAlternateColorOptions The row alternate color options (widget status, row alternate colors).
* @property rowFieldNamesStyle The table cell style of row field names.
* @property rowHeaderStyle The table cell style of the row headers.
* @property rowsLabelOptions The options for the label that is located above the row headers. This option is only applicable when `RowsLayout` is set to `HIERARCHY` .
* @property rowsLayout The layout for the row dimension headers of a pivot table. Choose one of the following options.
* - `TABULAR` : (Default) Each row field is displayed in a separate column.
* - `HIERARCHY` : All row fields are displayed in a single column. Indentation is used to differentiate row headers of different fields.
* @property singleMetricVisibility The visibility of the single metric options.
* @property toggleButtonsVisibility Determines the visibility of the pivot table.
*/
public data class DashboardPivotTableOptionsArgs(
public val cellStyle: Output? = null,
public val collapsedRowDimensionsVisibility: Output? = null,
public val columnHeaderStyle: Output? = null,
public val columnNamesVisibility: Output? = null,
public val defaultCellWidth: Output? = null,
public val metricPlacement: Output? = null,
public val rowAlternateColorOptions: Output? = null,
public val rowFieldNamesStyle: Output? = null,
public val rowHeaderStyle: Output? = null,
public val rowsLabelOptions: Output? = null,
public val rowsLayout: Output? = null,
public val singleMetricVisibility: Output? = null,
public val toggleButtonsVisibility: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.DashboardPivotTableOptionsArgs =
com.pulumi.awsnative.quicksight.inputs.DashboardPivotTableOptionsArgs.builder()
.cellStyle(cellStyle?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.collapsedRowDimensionsVisibility(
collapsedRowDimensionsVisibility?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.columnHeaderStyle(columnHeaderStyle?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.columnNamesVisibility(
columnNamesVisibility?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.defaultCellWidth(defaultCellWidth?.applyValue({ args0 -> args0 }))
.metricPlacement(metricPlacement?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rowAlternateColorOptions(
rowAlternateColorOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rowFieldNamesStyle(
rowFieldNamesStyle?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rowHeaderStyle(rowHeaderStyle?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rowsLabelOptions(rowsLabelOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rowsLayout(rowsLayout?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.singleMetricVisibility(
singleMetricVisibility?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.toggleButtonsVisibility(
toggleButtonsVisibility?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DashboardPivotTableOptionsArgs].
*/
@PulumiTagMarker
public class DashboardPivotTableOptionsArgsBuilder internal constructor() {
private var cellStyle: Output? = null
private var collapsedRowDimensionsVisibility: Output? = null
private var columnHeaderStyle: Output? = null
private var columnNamesVisibility: Output? = null
private var defaultCellWidth: Output? = null
private var metricPlacement: Output? = null
private var rowAlternateColorOptions: Output? = null
private var rowFieldNamesStyle: Output? = null
private var rowHeaderStyle: Output? = null
private var rowsLabelOptions: Output? = null
private var rowsLayout: Output? = null
private var singleMetricVisibility: Output? = null
private var toggleButtonsVisibility: Output? = null
/**
* @param value The table cell style of cells.
*/
@JvmName("iefqnycfkdrgiooq")
public suspend fun cellStyle(`value`: Output) {
this.cellStyle = value
}
/**
* @param value The visibility setting of a pivot table's collapsed row dimension fields. If the value of this structure is `HIDDEN` , all collapsed columns in a pivot table are automatically hidden. The default value is `VISIBLE` .
*/
@JvmName("dhjqfrlnaajgpvvl")
public suspend fun collapsedRowDimensionsVisibility(`value`: Output) {
this.collapsedRowDimensionsVisibility = value
}
/**
* @param value The table cell style of the column header.
*/
@JvmName("bqlgdtclvnvndutw")
public suspend fun columnHeaderStyle(`value`: Output) {
this.columnHeaderStyle = value
}
/**
* @param value The visibility of the column names.
*/
@JvmName("gljevfhtpvbxkvrk")
public suspend fun columnNamesVisibility(`value`: Output) {
this.columnNamesVisibility = value
}
/**
* @param value String based length that is composed of value and unit in px
*/
@JvmName("uxbtndalpuvknaxd")
public suspend fun defaultCellWidth(`value`: Output) {
this.defaultCellWidth = value
}
/**
* @param value The metric placement (row, column) options.
*/
@JvmName("oafxkffokelakice")
public suspend fun metricPlacement(`value`: Output) {
this.metricPlacement = value
}
/**
* @param value The row alternate color options (widget status, row alternate colors).
*/
@JvmName("dxkcopcdtdipmjvv")
public suspend fun rowAlternateColorOptions(`value`: Output) {
this.rowAlternateColorOptions = value
}
/**
* @param value The table cell style of row field names.
*/
@JvmName("ldfuksktcpcjhloy")
public suspend fun rowFieldNamesStyle(`value`: Output) {
this.rowFieldNamesStyle = value
}
/**
* @param value The table cell style of the row headers.
*/
@JvmName("lgjnnreunstweiyv")
public suspend fun rowHeaderStyle(`value`: Output) {
this.rowHeaderStyle = value
}
/**
* @param value The options for the label that is located above the row headers. This option is only applicable when `RowsLayout` is set to `HIERARCHY` .
*/
@JvmName("xygaqcbowhvrapmm")
public suspend fun rowsLabelOptions(`value`: Output) {
this.rowsLabelOptions = value
}
/**
* @param value The layout for the row dimension headers of a pivot table. Choose one of the following options.
* - `TABULAR` : (Default) Each row field is displayed in a separate column.
* - `HIERARCHY` : All row fields are displayed in a single column. Indentation is used to differentiate row headers of different fields.
*/
@JvmName("jxotlpkfyyuoasyf")
public suspend fun rowsLayout(`value`: Output) {
this.rowsLayout = value
}
/**
* @param value The visibility of the single metric options.
*/
@JvmName("qoxcdualibnrarcu")
public suspend fun singleMetricVisibility(`value`: Output) {
this.singleMetricVisibility = value
}
/**
* @param value Determines the visibility of the pivot table.
*/
@JvmName("sscelytepthwvimw")
public suspend fun toggleButtonsVisibility(`value`: Output) {
this.toggleButtonsVisibility = value
}
/**
* @param value The table cell style of cells.
*/
@JvmName("wwydsonrawchppwn")
public suspend fun cellStyle(`value`: DashboardTableCellStyleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cellStyle = mapped
}
/**
* @param argument The table cell style of cells.
*/
@JvmName("tdknbbtjatkamkeq")
public suspend fun cellStyle(argument: suspend DashboardTableCellStyleArgsBuilder.() -> Unit) {
val toBeMapped = DashboardTableCellStyleArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.cellStyle = mapped
}
/**
* @param value The visibility setting of a pivot table's collapsed row dimension fields. If the value of this structure is `HIDDEN` , all collapsed columns in a pivot table are automatically hidden. The default value is `VISIBLE` .
*/
@JvmName("quvcbclufxyjrcgn")
public suspend fun collapsedRowDimensionsVisibility(`value`: DashboardVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.collapsedRowDimensionsVisibility = mapped
}
/**
* @param value The table cell style of the column header.
*/
@JvmName("cjlybarvjkkxkxxc")
public suspend fun columnHeaderStyle(`value`: DashboardTableCellStyleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.columnHeaderStyle = mapped
}
/**
* @param argument The table cell style of the column header.
*/
@JvmName("gwhvptbbrmgqhsth")
public suspend fun columnHeaderStyle(argument: suspend DashboardTableCellStyleArgsBuilder.() -> Unit) {
val toBeMapped = DashboardTableCellStyleArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.columnHeaderStyle = mapped
}
/**
* @param value The visibility of the column names.
*/
@JvmName("fnrsthetihhbisys")
public suspend fun columnNamesVisibility(`value`: DashboardVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.columnNamesVisibility = mapped
}
/**
* @param value String based length that is composed of value and unit in px
*/
@JvmName("lphtxgfuxxxfpslr")
public suspend fun defaultCellWidth(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultCellWidth = mapped
}
/**
* @param value The metric placement (row, column) options.
*/
@JvmName("fimdwwhbphknnanq")
public suspend fun metricPlacement(`value`: DashboardPivotTableMetricPlacement?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricPlacement = mapped
}
/**
* @param value The row alternate color options (widget status, row alternate colors).
*/
@JvmName("sijghovyqmjiejbc")
public suspend fun rowAlternateColorOptions(`value`: DashboardRowAlternateColorOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowAlternateColorOptions = mapped
}
/**
* @param argument The row alternate color options (widget status, row alternate colors).
*/
@JvmName("vwnhdfegumvsshnf")
public suspend fun rowAlternateColorOptions(argument: suspend DashboardRowAlternateColorOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardRowAlternateColorOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rowAlternateColorOptions = mapped
}
/**
* @param value The table cell style of row field names.
*/
@JvmName("noqecxyjgevwklxc")
public suspend fun rowFieldNamesStyle(`value`: DashboardTableCellStyleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowFieldNamesStyle = mapped
}
/**
* @param argument The table cell style of row field names.
*/
@JvmName("qobojwfqylnaeiye")
public suspend fun rowFieldNamesStyle(argument: suspend DashboardTableCellStyleArgsBuilder.() -> Unit) {
val toBeMapped = DashboardTableCellStyleArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.rowFieldNamesStyle = mapped
}
/**
* @param value The table cell style of the row headers.
*/
@JvmName("lbinknytxyimhmpn")
public suspend fun rowHeaderStyle(`value`: DashboardTableCellStyleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowHeaderStyle = mapped
}
/**
* @param argument The table cell style of the row headers.
*/
@JvmName("gkvmcuafbydmafbk")
public suspend fun rowHeaderStyle(argument: suspend DashboardTableCellStyleArgsBuilder.() -> Unit) {
val toBeMapped = DashboardTableCellStyleArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.rowHeaderStyle = mapped
}
/**
* @param value The options for the label that is located above the row headers. This option is only applicable when `RowsLayout` is set to `HIERARCHY` .
*/
@JvmName("emhmaubpwqaqlwir")
public suspend fun rowsLabelOptions(`value`: DashboardPivotTableRowsLabelOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowsLabelOptions = mapped
}
/**
* @param argument The options for the label that is located above the row headers. This option is only applicable when `RowsLayout` is set to `HIERARCHY` .
*/
@JvmName("fxjspnnoobhqyoxg")
public suspend fun rowsLabelOptions(argument: suspend DashboardPivotTableRowsLabelOptionsArgsBuilder.() -> Unit) {
val toBeMapped = DashboardPivotTableRowsLabelOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rowsLabelOptions = mapped
}
/**
* @param value The layout for the row dimension headers of a pivot table. Choose one of the following options.
* - `TABULAR` : (Default) Each row field is displayed in a separate column.
* - `HIERARCHY` : All row fields are displayed in a single column. Indentation is used to differentiate row headers of different fields.
*/
@JvmName("xqquhwgxyuqegudo")
public suspend fun rowsLayout(`value`: DashboardPivotTableRowsLayout?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowsLayout = mapped
}
/**
* @param value The visibility of the single metric options.
*/
@JvmName("uokeoieqpjvtxnjq")
public suspend fun singleMetricVisibility(`value`: DashboardVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.singleMetricVisibility = mapped
}
/**
* @param value Determines the visibility of the pivot table.
*/
@JvmName("msywpcjmdxcwubmq")
public suspend fun toggleButtonsVisibility(`value`: DashboardVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.toggleButtonsVisibility = mapped
}
internal fun build(): DashboardPivotTableOptionsArgs = DashboardPivotTableOptionsArgs(
cellStyle = cellStyle,
collapsedRowDimensionsVisibility = collapsedRowDimensionsVisibility,
columnHeaderStyle = columnHeaderStyle,
columnNamesVisibility = columnNamesVisibility,
defaultCellWidth = defaultCellWidth,
metricPlacement = metricPlacement,
rowAlternateColorOptions = rowAlternateColorOptions,
rowFieldNamesStyle = rowFieldNamesStyle,
rowHeaderStyle = rowHeaderStyle,
rowsLabelOptions = rowsLabelOptions,
rowsLayout = rowsLayout,
singleMetricVisibility = singleMetricVisibility,
toggleButtonsVisibility = toggleButtonsVisibility,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy