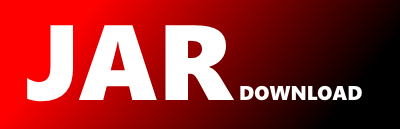
com.pulumi.awsnative.quicksight.kotlin.inputs.RefreshScheduleMapArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.RefreshScheduleMapArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.RefreshScheduleMapRefreshType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property refreshType The type of refresh that a dataset undergoes. Valid values are as follows:
* - `FULL_REFRESH` : A complete refresh of a dataset.
* - `INCREMENTAL_REFRESH` : A partial refresh of some rows of a dataset, based on the time window specified.
* For more information on full and incremental refreshes, see [Refreshing SPICE data](https://docs.aws.amazon.com/quicksight/latest/user/refreshing-imported-data.html) in the *Amazon QuickSight User Guide* .
* @property scheduleFrequency Information about the schedule frequency.
* @property scheduleId An unique identifier for the refresh schedule.
* @property startAfterDateTime The date time after which refresh is to be scheduled
*/
public data class RefreshScheduleMapArgs(
public val refreshType: Output? = null,
public val scheduleFrequency: Output? = null,
public val scheduleId: Output? = null,
public val startAfterDateTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.RefreshScheduleMapArgs =
com.pulumi.awsnative.quicksight.inputs.RefreshScheduleMapArgs.builder()
.refreshType(refreshType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scheduleFrequency(scheduleFrequency?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scheduleId(scheduleId?.applyValue({ args0 -> args0 }))
.startAfterDateTime(startAfterDateTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RefreshScheduleMapArgs].
*/
@PulumiTagMarker
public class RefreshScheduleMapArgsBuilder internal constructor() {
private var refreshType: Output? = null
private var scheduleFrequency: Output? = null
private var scheduleId: Output? = null
private var startAfterDateTime: Output? = null
/**
* @param value The type of refresh that a dataset undergoes. Valid values are as follows:
* - `FULL_REFRESH` : A complete refresh of a dataset.
* - `INCREMENTAL_REFRESH` : A partial refresh of some rows of a dataset, based on the time window specified.
* For more information on full and incremental refreshes, see [Refreshing SPICE data](https://docs.aws.amazon.com/quicksight/latest/user/refreshing-imported-data.html) in the *Amazon QuickSight User Guide* .
*/
@JvmName("pjbhwqjdrubtbofe")
public suspend fun refreshType(`value`: Output) {
this.refreshType = value
}
/**
* @param value Information about the schedule frequency.
*/
@JvmName("waymjlwwdekoejuc")
public suspend fun scheduleFrequency(`value`: Output) {
this.scheduleFrequency = value
}
/**
* @param value An unique identifier for the refresh schedule.
*/
@JvmName("dncsdwwicptmittb")
public suspend fun scheduleId(`value`: Output) {
this.scheduleId = value
}
/**
* @param value The date time after which refresh is to be scheduled
*/
@JvmName("ofwmupvqqjbcrdwl")
public suspend fun startAfterDateTime(`value`: Output) {
this.startAfterDateTime = value
}
/**
* @param value The type of refresh that a dataset undergoes. Valid values are as follows:
* - `FULL_REFRESH` : A complete refresh of a dataset.
* - `INCREMENTAL_REFRESH` : A partial refresh of some rows of a dataset, based on the time window specified.
* For more information on full and incremental refreshes, see [Refreshing SPICE data](https://docs.aws.amazon.com/quicksight/latest/user/refreshing-imported-data.html) in the *Amazon QuickSight User Guide* .
*/
@JvmName("olyoktksaygofglq")
public suspend fun refreshType(`value`: RefreshScheduleMapRefreshType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.refreshType = mapped
}
/**
* @param value Information about the schedule frequency.
*/
@JvmName("xsudbnlsrnibficm")
public suspend fun scheduleFrequency(`value`: RefreshScheduleMapScheduleFrequencyPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduleFrequency = mapped
}
/**
* @param argument Information about the schedule frequency.
*/
@JvmName("wqjwtplvytxojomk")
public suspend fun scheduleFrequency(argument: suspend RefreshScheduleMapScheduleFrequencyPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = RefreshScheduleMapScheduleFrequencyPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.scheduleFrequency = mapped
}
/**
* @param value An unique identifier for the refresh schedule.
*/
@JvmName("erbewuqmilgclswi")
public suspend fun scheduleId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduleId = mapped
}
/**
* @param value The date time after which refresh is to be scheduled
*/
@JvmName("idjbkcnsuutxknhm")
public suspend fun startAfterDateTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startAfterDateTime = mapped
}
internal fun build(): RefreshScheduleMapArgs = RefreshScheduleMapArgs(
refreshType = refreshType,
scheduleFrequency = scheduleFrequency,
scheduleId = scheduleId,
startAfterDateTime = startAfterDateTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy