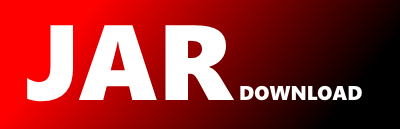
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateTimeBasedForecastPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateTimeBasedForecastPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property lowerBoundary The lower boundary setup of a forecast computation.
* @property periodsBackward The periods backward setup of a forecast computation.
* @property periodsForward The periods forward setup of a forecast computation.
* @property predictionInterval The prediction interval setup of a forecast computation.
* @property seasonality The seasonality setup of a forecast computation. Choose one of the following options:
* - `NULL` : The input is set to `NULL` .
* - `NON_NULL` : The input is set to a custom value.
* @property upperBoundary The upper boundary setup of a forecast computation.
*/
public data class TemplateTimeBasedForecastPropertiesArgs(
public val lowerBoundary: Output? = null,
public val periodsBackward: Output? = null,
public val periodsForward: Output? = null,
public val predictionInterval: Output? = null,
public val seasonality: Output? = null,
public val upperBoundary: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateTimeBasedForecastPropertiesArgs =
com.pulumi.awsnative.quicksight.inputs.TemplateTimeBasedForecastPropertiesArgs.builder()
.lowerBoundary(lowerBoundary?.applyValue({ args0 -> args0 }))
.periodsBackward(periodsBackward?.applyValue({ args0 -> args0 }))
.periodsForward(periodsForward?.applyValue({ args0 -> args0 }))
.predictionInterval(predictionInterval?.applyValue({ args0 -> args0 }))
.seasonality(seasonality?.applyValue({ args0 -> args0 }))
.upperBoundary(upperBoundary?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TemplateTimeBasedForecastPropertiesArgs].
*/
@PulumiTagMarker
public class TemplateTimeBasedForecastPropertiesArgsBuilder internal constructor() {
private var lowerBoundary: Output? = null
private var periodsBackward: Output? = null
private var periodsForward: Output? = null
private var predictionInterval: Output? = null
private var seasonality: Output? = null
private var upperBoundary: Output? = null
/**
* @param value The lower boundary setup of a forecast computation.
*/
@JvmName("hnqeqssqorpykdjk")
public suspend fun lowerBoundary(`value`: Output) {
this.lowerBoundary = value
}
/**
* @param value The periods backward setup of a forecast computation.
*/
@JvmName("krdbkmadevalfcvd")
public suspend fun periodsBackward(`value`: Output) {
this.periodsBackward = value
}
/**
* @param value The periods forward setup of a forecast computation.
*/
@JvmName("dlvehllyduukyyno")
public suspend fun periodsForward(`value`: Output) {
this.periodsForward = value
}
/**
* @param value The prediction interval setup of a forecast computation.
*/
@JvmName("xfyiepfjsmodplaq")
public suspend fun predictionInterval(`value`: Output) {
this.predictionInterval = value
}
/**
* @param value The seasonality setup of a forecast computation. Choose one of the following options:
* - `NULL` : The input is set to `NULL` .
* - `NON_NULL` : The input is set to a custom value.
*/
@JvmName("xdrbdwueycbryrdw")
public suspend fun seasonality(`value`: Output) {
this.seasonality = value
}
/**
* @param value The upper boundary setup of a forecast computation.
*/
@JvmName("tonkspjpasycrweg")
public suspend fun upperBoundary(`value`: Output) {
this.upperBoundary = value
}
/**
* @param value The lower boundary setup of a forecast computation.
*/
@JvmName("ycpfnqejsthlvfkf")
public suspend fun lowerBoundary(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lowerBoundary = mapped
}
/**
* @param value The periods backward setup of a forecast computation.
*/
@JvmName("tosuseakmtaouail")
public suspend fun periodsBackward(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.periodsBackward = mapped
}
/**
* @param value The periods forward setup of a forecast computation.
*/
@JvmName("jojeeioximantnpn")
public suspend fun periodsForward(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.periodsForward = mapped
}
/**
* @param value The prediction interval setup of a forecast computation.
*/
@JvmName("cwofmmwolmapakrr")
public suspend fun predictionInterval(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.predictionInterval = mapped
}
/**
* @param value The seasonality setup of a forecast computation. Choose one of the following options:
* - `NULL` : The input is set to `NULL` .
* - `NON_NULL` : The input is set to a custom value.
*/
@JvmName("rwwxwswignukketf")
public suspend fun seasonality(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.seasonality = mapped
}
/**
* @param value The upper boundary setup of a forecast computation.
*/
@JvmName("slivxixerdsnowkt")
public suspend fun upperBoundary(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.upperBoundary = mapped
}
internal fun build(): TemplateTimeBasedForecastPropertiesArgs =
TemplateTimeBasedForecastPropertiesArgs(
lowerBoundary = lowerBoundary,
periodsBackward = periodsBackward,
periodsForward = periodsForward,
predictionInterval = predictionInterval,
seasonality = seasonality,
upperBoundary = upperBoundary,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy