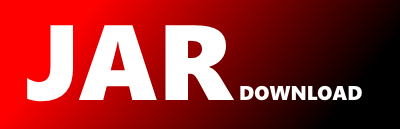
com.pulumi.awsnative.rds.kotlin.CustomDbEngineVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.rds.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.rds.CustomDbEngineVersionArgs.builder
import com.pulumi.awsnative.rds.kotlin.enums.CustomDbEngineVersionStatus
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The AWS::RDS::CustomDBEngineVersion resource creates an Amazon RDS custom DB engine version.
* @property databaseInstallationFilesS3BucketName The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a valid bucket name is `my-custom-installation-files`.
* @property databaseInstallationFilesS3Prefix The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid bucket name is `123456789012/cev1`. If this setting isn't specified, no prefix is assumed.
* @property description An optional description of your CEV.
* @property engine The database engine to use for your custom engine version (CEV). The only supported value is `custom-oracle-ee`.
* @property engineVersion The name of your CEV. The name format is 19.customized_string . For example, a valid name is 19.my_cev1. This setting is required for RDS Custom for Oracle, but optional for Amazon RDS. The combination of Engine and EngineVersion is unique per customer per Region.
* @property imageId The identifier of Amazon Machine Image (AMI) used for CEV.
* @property kmsKeyId The AWS KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS Custom, but optional for Amazon RDS.
* @property manifest The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3. Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in which they are listed.
* @property sourceCustomDbEngineVersionIdentifier The identifier of the source custom engine version.
* @property status The availability status to be assigned to the CEV.
* @property tags An array of key-value pairs to apply to this resource.
* @property useAwsProvidedLatestImage A value that indicates whether AWS provided latest image is applied automatically to the Custom Engine Version. By default, AWS provided latest image is applied automatically. This value is only applied on create.
*/
public data class CustomDbEngineVersionArgs(
public val databaseInstallationFilesS3BucketName: Output? = null,
public val databaseInstallationFilesS3Prefix: Output? = null,
public val description: Output? = null,
public val engine: Output? = null,
public val engineVersion: Output? = null,
public val imageId: Output? = null,
public val kmsKeyId: Output? = null,
public val manifest: Output? = null,
public val sourceCustomDbEngineVersionIdentifier: Output? = null,
public val status: Output? = null,
public val tags: Output>? = null,
public val useAwsProvidedLatestImage: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.rds.CustomDbEngineVersionArgs =
com.pulumi.awsnative.rds.CustomDbEngineVersionArgs.builder()
.databaseInstallationFilesS3BucketName(
databaseInstallationFilesS3BucketName?.applyValue({ args0 ->
args0
}),
)
.databaseInstallationFilesS3Prefix(
databaseInstallationFilesS3Prefix?.applyValue({ args0 ->
args0
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.engine(engine?.applyValue({ args0 -> args0 }))
.engineVersion(engineVersion?.applyValue({ args0 -> args0 }))
.imageId(imageId?.applyValue({ args0 -> args0 }))
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.manifest(manifest?.applyValue({ args0 -> args0 }))
.sourceCustomDbEngineVersionIdentifier(
sourceCustomDbEngineVersionIdentifier?.applyValue({ args0 ->
args0
}),
)
.status(status?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.useAwsProvidedLatestImage(useAwsProvidedLatestImage?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CustomDbEngineVersionArgs].
*/
@PulumiTagMarker
public class CustomDbEngineVersionArgsBuilder internal constructor() {
private var databaseInstallationFilesS3BucketName: Output? = null
private var databaseInstallationFilesS3Prefix: Output? = null
private var description: Output? = null
private var engine: Output? = null
private var engineVersion: Output? = null
private var imageId: Output? = null
private var kmsKeyId: Output? = null
private var manifest: Output? = null
private var sourceCustomDbEngineVersionIdentifier: Output? = null
private var status: Output? = null
private var tags: Output>? = null
private var useAwsProvidedLatestImage: Output? = null
/**
* @param value The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a valid bucket name is `my-custom-installation-files`.
*/
@JvmName("jasqlxstbobmttnk")
public suspend fun databaseInstallationFilesS3BucketName(`value`: Output) {
this.databaseInstallationFilesS3BucketName = value
}
/**
* @param value The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid bucket name is `123456789012/cev1`. If this setting isn't specified, no prefix is assumed.
*/
@JvmName("pwwatxxrdpjrwaeo")
public suspend fun databaseInstallationFilesS3Prefix(`value`: Output) {
this.databaseInstallationFilesS3Prefix = value
}
/**
* @param value An optional description of your CEV.
*/
@JvmName("mtfmefxpxtfptcgp")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The database engine to use for your custom engine version (CEV). The only supported value is `custom-oracle-ee`.
*/
@JvmName("exhhghykuuhotruf")
public suspend fun engine(`value`: Output) {
this.engine = value
}
/**
* @param value The name of your CEV. The name format is 19.customized_string . For example, a valid name is 19.my_cev1. This setting is required for RDS Custom for Oracle, but optional for Amazon RDS. The combination of Engine and EngineVersion is unique per customer per Region.
*/
@JvmName("srlckejjjrgebjvs")
public suspend fun engineVersion(`value`: Output) {
this.engineVersion = value
}
/**
* @param value The identifier of Amazon Machine Image (AMI) used for CEV.
*/
@JvmName("qfguvtmgqcurbvgb")
public suspend fun imageId(`value`: Output) {
this.imageId = value
}
/**
* @param value The AWS KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS Custom, but optional for Amazon RDS.
*/
@JvmName("ppayhyttrbwnolkv")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3. Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in which they are listed.
*/
@JvmName("nsbhjkmfsvfriflr")
public suspend fun manifest(`value`: Output) {
this.manifest = value
}
/**
* @param value The identifier of the source custom engine version.
*/
@JvmName("fajwxsnetojuewnw")
public suspend fun sourceCustomDbEngineVersionIdentifier(`value`: Output) {
this.sourceCustomDbEngineVersionIdentifier = value
}
/**
* @param value The availability status to be assigned to the CEV.
*/
@JvmName("oewhmeqfqssharrl")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value An array of key-value pairs to apply to this resource.
*/
@JvmName("tfojmgrpglettnrq")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("cnmkswkftvvvyuxi")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values An array of key-value pairs to apply to this resource.
*/
@JvmName("fnhdjaqwloagwfrp")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy