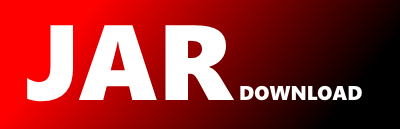
com.pulumi.awsnative.redshift.kotlin.RedshiftFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.redshift.kotlin
import com.pulumi.awsnative.redshift.RedshiftFunctions.getClusterParameterGroupPlain
import com.pulumi.awsnative.redshift.RedshiftFunctions.getClusterPlain
import com.pulumi.awsnative.redshift.RedshiftFunctions.getClusterSubnetGroupPlain
import com.pulumi.awsnative.redshift.RedshiftFunctions.getEndpointAccessPlain
import com.pulumi.awsnative.redshift.RedshiftFunctions.getEndpointAuthorizationPlain
import com.pulumi.awsnative.redshift.RedshiftFunctions.getEventSubscriptionPlain
import com.pulumi.awsnative.redshift.RedshiftFunctions.getScheduledActionPlain
import com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterParameterGroupPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterParameterGroupPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterSubnetGroupPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterSubnetGroupPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.inputs.GetEndpointAccessPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetEndpointAccessPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.inputs.GetEndpointAuthorizationPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetEndpointAuthorizationPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.inputs.GetEventSubscriptionPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetEventSubscriptionPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.inputs.GetScheduledActionPlainArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.GetScheduledActionPlainArgsBuilder
import com.pulumi.awsnative.redshift.kotlin.outputs.GetClusterParameterGroupResult
import com.pulumi.awsnative.redshift.kotlin.outputs.GetClusterResult
import com.pulumi.awsnative.redshift.kotlin.outputs.GetClusterSubnetGroupResult
import com.pulumi.awsnative.redshift.kotlin.outputs.GetEndpointAccessResult
import com.pulumi.awsnative.redshift.kotlin.outputs.GetEndpointAuthorizationResult
import com.pulumi.awsnative.redshift.kotlin.outputs.GetEventSubscriptionResult
import com.pulumi.awsnative.redshift.kotlin.outputs.GetScheduledActionResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.redshift.kotlin.outputs.GetClusterParameterGroupResult.Companion.toKotlin as getClusterParameterGroupResultToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.GetClusterResult.Companion.toKotlin as getClusterResultToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.GetClusterSubnetGroupResult.Companion.toKotlin as getClusterSubnetGroupResultToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.GetEndpointAccessResult.Companion.toKotlin as getEndpointAccessResultToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.GetEndpointAuthorizationResult.Companion.toKotlin as getEndpointAuthorizationResultToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.GetEventSubscriptionResult.Companion.toKotlin as getEventSubscriptionResultToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.GetScheduledActionResult.Companion.toKotlin as getScheduledActionResultToKotlin
public object RedshiftFunctions {
/**
* An example resource schema demonstrating some basic constructs and validation rules.
* @param argument null
* @return null
*/
public suspend fun getCluster(argument: GetClusterPlainArgs): GetClusterResult =
getClusterResultToKotlin(getClusterPlain(argument.toJava()).await())
/**
* @see [getCluster].
* @param clusterIdentifier A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent cluster operations such as deleting or modifying. All alphabetical characters must be lower case, no hypens at the end, no two consecutive hyphens. Cluster name should be unique for all clusters within an AWS account
* @return null
*/
public suspend fun getCluster(clusterIdentifier: String): GetClusterResult {
val argument = GetClusterPlainArgs(
clusterIdentifier = clusterIdentifier,
)
return getClusterResultToKotlin(getClusterPlain(argument.toJava()).await())
}
/**
* @see [getCluster].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterPlainArgs].
* @return null
*/
public suspend fun getCluster(argument: suspend GetClusterPlainArgsBuilder.() -> Unit): GetClusterResult {
val builder = GetClusterPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getClusterResultToKotlin(getClusterPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Redshift::ClusterParameterGroup
* @param argument null
* @return null
*/
public suspend fun getClusterParameterGroup(argument: GetClusterParameterGroupPlainArgs): GetClusterParameterGroupResult =
getClusterParameterGroupResultToKotlin(getClusterParameterGroupPlain(argument.toJava()).await())
/**
* @see [getClusterParameterGroup].
* @param parameterGroupName The name of the cluster parameter group.
* @return null
*/
public suspend fun getClusterParameterGroup(parameterGroupName: String): GetClusterParameterGroupResult {
val argument = GetClusterParameterGroupPlainArgs(
parameterGroupName = parameterGroupName,
)
return getClusterParameterGroupResultToKotlin(getClusterParameterGroupPlain(argument.toJava()).await())
}
/**
* @see [getClusterParameterGroup].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterParameterGroupPlainArgs].
* @return null
*/
public suspend fun getClusterParameterGroup(argument: suspend GetClusterParameterGroupPlainArgsBuilder.() -> Unit): GetClusterParameterGroupResult {
val builder = GetClusterParameterGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getClusterParameterGroupResultToKotlin(getClusterParameterGroupPlain(builtArgument.toJava()).await())
}
/**
* Specifies an Amazon Redshift subnet group.
* @param argument null
* @return null
*/
public suspend fun getClusterSubnetGroup(argument: GetClusterSubnetGroupPlainArgs): GetClusterSubnetGroupResult =
getClusterSubnetGroupResultToKotlin(getClusterSubnetGroupPlain(argument.toJava()).await())
/**
* @see [getClusterSubnetGroup].
* @param clusterSubnetGroupName This name must be unique for all subnet groups that are created by your AWS account. If costumer do not provide it, cloudformation will generate it. Must not be "Default".
* @return null
*/
public suspend fun getClusterSubnetGroup(clusterSubnetGroupName: String): GetClusterSubnetGroupResult {
val argument = GetClusterSubnetGroupPlainArgs(
clusterSubnetGroupName = clusterSubnetGroupName,
)
return getClusterSubnetGroupResultToKotlin(getClusterSubnetGroupPlain(argument.toJava()).await())
}
/**
* @see [getClusterSubnetGroup].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetClusterSubnetGroupPlainArgs].
* @return null
*/
public suspend fun getClusterSubnetGroup(argument: suspend GetClusterSubnetGroupPlainArgsBuilder.() -> Unit): GetClusterSubnetGroupResult {
val builder = GetClusterSubnetGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getClusterSubnetGroupResultToKotlin(getClusterSubnetGroupPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for a Redshift-managed VPC endpoint.
* @param argument null
* @return null
*/
public suspend fun getEndpointAccess(argument: GetEndpointAccessPlainArgs): GetEndpointAccessResult =
getEndpointAccessResultToKotlin(getEndpointAccessPlain(argument.toJava()).await())
/**
* @see [getEndpointAccess].
* @param endpointName The name of the endpoint.
* @return null
*/
public suspend fun getEndpointAccess(endpointName: String): GetEndpointAccessResult {
val argument = GetEndpointAccessPlainArgs(
endpointName = endpointName,
)
return getEndpointAccessResultToKotlin(getEndpointAccessPlain(argument.toJava()).await())
}
/**
* @see [getEndpointAccess].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetEndpointAccessPlainArgs].
* @return null
*/
public suspend fun getEndpointAccess(argument: suspend GetEndpointAccessPlainArgsBuilder.() -> Unit): GetEndpointAccessResult {
val builder = GetEndpointAccessPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEndpointAccessResultToKotlin(getEndpointAccessPlain(builtArgument.toJava()).await())
}
/**
* Describes an endpoint authorization for authorizing Redshift-managed VPC endpoint access to a cluster across AWS accounts.
* @param argument null
* @return null
*/
public suspend fun getEndpointAuthorization(argument: GetEndpointAuthorizationPlainArgs): GetEndpointAuthorizationResult =
getEndpointAuthorizationResultToKotlin(getEndpointAuthorizationPlain(argument.toJava()).await())
/**
* @see [getEndpointAuthorization].
* @param account The target AWS account ID to grant or revoke access for.
* @param clusterIdentifier The cluster identifier.
* @return null
*/
public suspend fun getEndpointAuthorization(account: String, clusterIdentifier: String): GetEndpointAuthorizationResult {
val argument = GetEndpointAuthorizationPlainArgs(
account = account,
clusterIdentifier = clusterIdentifier,
)
return getEndpointAuthorizationResultToKotlin(getEndpointAuthorizationPlain(argument.toJava()).await())
}
/**
* @see [getEndpointAuthorization].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetEndpointAuthorizationPlainArgs].
* @return null
*/
public suspend fun getEndpointAuthorization(argument: suspend GetEndpointAuthorizationPlainArgsBuilder.() -> Unit): GetEndpointAuthorizationResult {
val builder = GetEndpointAuthorizationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEndpointAuthorizationResultToKotlin(getEndpointAuthorizationPlain(builtArgument.toJava()).await())
}
/**
* The `AWS::Redshift::EventSubscription` resource creates an Amazon Redshift Event Subscription.
* @param argument null
* @return null
*/
public suspend fun getEventSubscription(argument: GetEventSubscriptionPlainArgs): GetEventSubscriptionResult =
getEventSubscriptionResultToKotlin(getEventSubscriptionPlain(argument.toJava()).await())
/**
* @see [getEventSubscription].
* @param subscriptionName The name of the Amazon Redshift event notification subscription
* @return null
*/
public suspend fun getEventSubscription(subscriptionName: String): GetEventSubscriptionResult {
val argument = GetEventSubscriptionPlainArgs(
subscriptionName = subscriptionName,
)
return getEventSubscriptionResultToKotlin(getEventSubscriptionPlain(argument.toJava()).await())
}
/**
* @see [getEventSubscription].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetEventSubscriptionPlainArgs].
* @return null
*/
public suspend fun getEventSubscription(argument: suspend GetEventSubscriptionPlainArgsBuilder.() -> Unit): GetEventSubscriptionResult {
val builder = GetEventSubscriptionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEventSubscriptionResultToKotlin(getEventSubscriptionPlain(builtArgument.toJava()).await())
}
/**
* The `AWS::Redshift::ScheduledAction` resource creates an Amazon Redshift Scheduled Action.
* @param argument null
* @return null
*/
public suspend fun getScheduledAction(argument: GetScheduledActionPlainArgs): GetScheduledActionResult =
getScheduledActionResultToKotlin(getScheduledActionPlain(argument.toJava()).await())
/**
* @see [getScheduledAction].
* @param scheduledActionName The name of the scheduled action. The name must be unique within an account.
* @return null
*/
public suspend fun getScheduledAction(scheduledActionName: String): GetScheduledActionResult {
val argument = GetScheduledActionPlainArgs(
scheduledActionName = scheduledActionName,
)
return getScheduledActionResultToKotlin(getScheduledActionPlain(argument.toJava()).await())
}
/**
* @see [getScheduledAction].
* @param argument Builder for [com.pulumi.awsnative.redshift.kotlin.inputs.GetScheduledActionPlainArgs].
* @return null
*/
public suspend fun getScheduledAction(argument: suspend GetScheduledActionPlainArgsBuilder.() -> Unit): GetScheduledActionResult {
val builder = GetScheduledActionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getScheduledActionResultToKotlin(getScheduledActionPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy