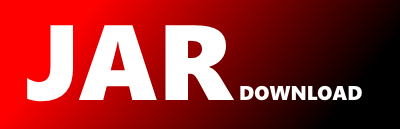
com.pulumi.awsnative.resiliencehub.kotlin.inputs.AppPhysicalResourceIdArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.resiliencehub.kotlin.inputs
import com.pulumi.awsnative.resiliencehub.inputs.AppPhysicalResourceIdArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property awsAccountId The AWS account that owns the physical resource.
* @property awsRegion The AWS Region that the physical resource is located in.
* @property identifier Identifier of the physical resource.
* @property type Specifies the type of physical resource identifier.
* - **Arn** - The resource identifier is an Amazon Resource Name (ARN) and it can identify the following list of resources:
* - `AWS::ECS::Service`
* - `AWS::EFS::FileSystem`
* - `AWS::ElasticLoadBalancingV2::LoadBalancer`
* - `AWS::Lambda::Function`
* - `AWS::SNS::Topic`
* - **Native** - The resource identifier is an AWS Resilience Hub -native identifier and it can identify the following list of resources:
* - `AWS::ApiGateway::RestApi`
* - `AWS::ApiGatewayV2::Api`
* - `AWS::AutoScaling::AutoScalingGroup`
* - `AWS::DocDB::DBCluster`
* - `AWS::DocDB::DBGlobalCluster`
* - `AWS::DocDB::DBInstance`
* - `AWS::DynamoDB::GlobalTable`
* - `AWS::DynamoDB::Table`
* - `AWS::EC2::EC2Fleet`
* - `AWS::EC2::Instance`
* - `AWS::EC2::NatGateway`
* - `AWS::EC2::Volume`
* - `AWS::ElasticLoadBalancing::LoadBalancer`
* - `AWS::RDS::DBCluster`
* - `AWS::RDS::DBInstance`
* - `AWS::RDS::GlobalCluster`
* - `AWS::Route53::RecordSet`
* - `AWS::S3::Bucket`
* - `AWS::SQS::Queue`
*/
public data class AppPhysicalResourceIdArgs(
public val awsAccountId: Output? = null,
public val awsRegion: Output? = null,
public val identifier: Output,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.resiliencehub.inputs.AppPhysicalResourceIdArgs =
com.pulumi.awsnative.resiliencehub.inputs.AppPhysicalResourceIdArgs.builder()
.awsAccountId(awsAccountId?.applyValue({ args0 -> args0 }))
.awsRegion(awsRegion?.applyValue({ args0 -> args0 }))
.identifier(identifier.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AppPhysicalResourceIdArgs].
*/
@PulumiTagMarker
public class AppPhysicalResourceIdArgsBuilder internal constructor() {
private var awsAccountId: Output? = null
private var awsRegion: Output? = null
private var identifier: Output? = null
private var type: Output? = null
/**
* @param value The AWS account that owns the physical resource.
*/
@JvmName("ryqphqvrwxsijhrn")
public suspend fun awsAccountId(`value`: Output) {
this.awsAccountId = value
}
/**
* @param value The AWS Region that the physical resource is located in.
*/
@JvmName("fhsoubbyjnafqduj")
public suspend fun awsRegion(`value`: Output) {
this.awsRegion = value
}
/**
* @param value Identifier of the physical resource.
*/
@JvmName("pyaycpdebprthtnu")
public suspend fun identifier(`value`: Output) {
this.identifier = value
}
/**
* @param value Specifies the type of physical resource identifier.
* - **Arn** - The resource identifier is an Amazon Resource Name (ARN) and it can identify the following list of resources:
* - `AWS::ECS::Service`
* - `AWS::EFS::FileSystem`
* - `AWS::ElasticLoadBalancingV2::LoadBalancer`
* - `AWS::Lambda::Function`
* - `AWS::SNS::Topic`
* - **Native** - The resource identifier is an AWS Resilience Hub -native identifier and it can identify the following list of resources:
* - `AWS::ApiGateway::RestApi`
* - `AWS::ApiGatewayV2::Api`
* - `AWS::AutoScaling::AutoScalingGroup`
* - `AWS::DocDB::DBCluster`
* - `AWS::DocDB::DBGlobalCluster`
* - `AWS::DocDB::DBInstance`
* - `AWS::DynamoDB::GlobalTable`
* - `AWS::DynamoDB::Table`
* - `AWS::EC2::EC2Fleet`
* - `AWS::EC2::Instance`
* - `AWS::EC2::NatGateway`
* - `AWS::EC2::Volume`
* - `AWS::ElasticLoadBalancing::LoadBalancer`
* - `AWS::RDS::DBCluster`
* - `AWS::RDS::DBInstance`
* - `AWS::RDS::GlobalCluster`
* - `AWS::Route53::RecordSet`
* - `AWS::S3::Bucket`
* - `AWS::SQS::Queue`
*/
@JvmName("dklfbxaxkkivwjdq")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The AWS account that owns the physical resource.
*/
@JvmName("vlpqlprndjkpeths")
public suspend fun awsAccountId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.awsAccountId = mapped
}
/**
* @param value The AWS Region that the physical resource is located in.
*/
@JvmName("mnnpljkksgtikaan")
public suspend fun awsRegion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.awsRegion = mapped
}
/**
* @param value Identifier of the physical resource.
*/
@JvmName("aclnmmgxrmvvhtdx")
public suspend fun identifier(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.identifier = mapped
}
/**
* @param value Specifies the type of physical resource identifier.
* - **Arn** - The resource identifier is an Amazon Resource Name (ARN) and it can identify the following list of resources:
* - `AWS::ECS::Service`
* - `AWS::EFS::FileSystem`
* - `AWS::ElasticLoadBalancingV2::LoadBalancer`
* - `AWS::Lambda::Function`
* - `AWS::SNS::Topic`
* - **Native** - The resource identifier is an AWS Resilience Hub -native identifier and it can identify the following list of resources:
* - `AWS::ApiGateway::RestApi`
* - `AWS::ApiGatewayV2::Api`
* - `AWS::AutoScaling::AutoScalingGroup`
* - `AWS::DocDB::DBCluster`
* - `AWS::DocDB::DBGlobalCluster`
* - `AWS::DocDB::DBInstance`
* - `AWS::DynamoDB::GlobalTable`
* - `AWS::DynamoDB::Table`
* - `AWS::EC2::EC2Fleet`
* - `AWS::EC2::Instance`
* - `AWS::EC2::NatGateway`
* - `AWS::EC2::Volume`
* - `AWS::ElasticLoadBalancing::LoadBalancer`
* - `AWS::RDS::DBCluster`
* - `AWS::RDS::DBInstance`
* - `AWS::RDS::GlobalCluster`
* - `AWS::Route53::RecordSet`
* - `AWS::S3::Bucket`
* - `AWS::SQS::Queue`
*/
@JvmName("hdxllshjnocljtbx")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): AppPhysicalResourceIdArgs = AppPhysicalResourceIdArgs(
awsAccountId = awsAccountId,
awsRegion = awsRegion,
identifier = identifier ?: throw PulumiNullFieldException("identifier"),
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy