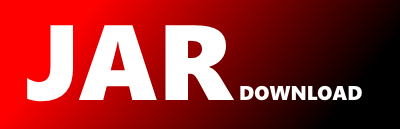
com.pulumi.awsnative.s3.kotlin.inputs.StorageLensAccountLevelArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin.inputs
import com.pulumi.awsnative.s3.inputs.StorageLensAccountLevelArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Account-level metrics configurations.
* @property activityMetrics This property contains the details of account-level activity metrics for S3 Storage Lens.
* @property advancedCostOptimizationMetrics This property contains the details of account-level advanced cost optimization metrics for S3 Storage Lens.
* @property advancedDataProtectionMetrics This property contains the details of account-level advanced data protection metrics for S3 Storage Lens.
* @property bucketLevel This property contains the details of the account-level bucket-level configurations for Amazon S3 Storage Lens.
* @property detailedStatusCodesMetrics This property contains the details of account-level detailed status code metrics for S3 Storage Lens.
* @property storageLensGroupLevel This property determines the scope of Storage Lens group data that is displayed in the Storage Lens dashboard.
*/
public data class StorageLensAccountLevelArgs(
public val activityMetrics: Output? = null,
public val advancedCostOptimizationMetrics: Output? = null,
public val advancedDataProtectionMetrics: Output? =
null,
public val bucketLevel: Output,
public val detailedStatusCodesMetrics: Output? = null,
public val storageLensGroupLevel: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.s3.inputs.StorageLensAccountLevelArgs =
com.pulumi.awsnative.s3.inputs.StorageLensAccountLevelArgs.builder()
.activityMetrics(activityMetrics?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.advancedCostOptimizationMetrics(
advancedCostOptimizationMetrics?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.advancedDataProtectionMetrics(
advancedDataProtectionMetrics?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.bucketLevel(bucketLevel.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.detailedStatusCodesMetrics(
detailedStatusCodesMetrics?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.storageLensGroupLevel(
storageLensGroupLevel?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [StorageLensAccountLevelArgs].
*/
@PulumiTagMarker
public class StorageLensAccountLevelArgsBuilder internal constructor() {
private var activityMetrics: Output? = null
private var advancedCostOptimizationMetrics:
Output? = null
private var advancedDataProtectionMetrics: Output? =
null
private var bucketLevel: Output? = null
private var detailedStatusCodesMetrics: Output? = null
private var storageLensGroupLevel: Output? = null
/**
* @param value This property contains the details of account-level activity metrics for S3 Storage Lens.
*/
@JvmName("edkvlkaveskclqff")
public suspend fun activityMetrics(`value`: Output) {
this.activityMetrics = value
}
/**
* @param value This property contains the details of account-level advanced cost optimization metrics for S3 Storage Lens.
*/
@JvmName("miqavypkviemgeoi")
public suspend fun advancedCostOptimizationMetrics(`value`: Output) {
this.advancedCostOptimizationMetrics = value
}
/**
* @param value This property contains the details of account-level advanced data protection metrics for S3 Storage Lens.
*/
@JvmName("ajjjcplshugkumki")
public suspend fun advancedDataProtectionMetrics(`value`: Output) {
this.advancedDataProtectionMetrics = value
}
/**
* @param value This property contains the details of the account-level bucket-level configurations for Amazon S3 Storage Lens.
*/
@JvmName("sfhjrdkaxfmvvatw")
public suspend fun bucketLevel(`value`: Output) {
this.bucketLevel = value
}
/**
* @param value This property contains the details of account-level detailed status code metrics for S3 Storage Lens.
*/
@JvmName("hrquuwrwnxuwrqkp")
public suspend fun detailedStatusCodesMetrics(`value`: Output) {
this.detailedStatusCodesMetrics = value
}
/**
* @param value This property determines the scope of Storage Lens group data that is displayed in the Storage Lens dashboard.
*/
@JvmName("sprwblxulwtilmcy")
public suspend fun storageLensGroupLevel(`value`: Output) {
this.storageLensGroupLevel = value
}
/**
* @param value This property contains the details of account-level activity metrics for S3 Storage Lens.
*/
@JvmName("tcftdfwqtyufejce")
public suspend fun activityMetrics(`value`: StorageLensActivityMetricsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.activityMetrics = mapped
}
/**
* @param argument This property contains the details of account-level activity metrics for S3 Storage Lens.
*/
@JvmName("gpihqdayepvpadvs")
public suspend fun activityMetrics(argument: suspend StorageLensActivityMetricsArgsBuilder.() -> Unit) {
val toBeMapped = StorageLensActivityMetricsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.activityMetrics = mapped
}
/**
* @param value This property contains the details of account-level advanced cost optimization metrics for S3 Storage Lens.
*/
@JvmName("fqytdwhjgutrdpsu")
public suspend fun advancedCostOptimizationMetrics(`value`: StorageLensAdvancedCostOptimizationMetricsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.advancedCostOptimizationMetrics = mapped
}
/**
* @param argument This property contains the details of account-level advanced cost optimization metrics for S3 Storage Lens.
*/
@JvmName("cqoggljkrmkecdtu")
public suspend fun advancedCostOptimizationMetrics(argument: suspend StorageLensAdvancedCostOptimizationMetricsArgsBuilder.() -> Unit) {
val toBeMapped = StorageLensAdvancedCostOptimizationMetricsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.advancedCostOptimizationMetrics = mapped
}
/**
* @param value This property contains the details of account-level advanced data protection metrics for S3 Storage Lens.
*/
@JvmName("uagsybbigjuwysvt")
public suspend fun advancedDataProtectionMetrics(`value`: StorageLensAdvancedDataProtectionMetricsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.advancedDataProtectionMetrics = mapped
}
/**
* @param argument This property contains the details of account-level advanced data protection metrics for S3 Storage Lens.
*/
@JvmName("ruudmkafrwdudsiw")
public suspend fun advancedDataProtectionMetrics(argument: suspend StorageLensAdvancedDataProtectionMetricsArgsBuilder.() -> Unit) {
val toBeMapped = StorageLensAdvancedDataProtectionMetricsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.advancedDataProtectionMetrics = mapped
}
/**
* @param value This property contains the details of the account-level bucket-level configurations for Amazon S3 Storage Lens.
*/
@JvmName("pnqsuouixvfqjkgb")
public suspend fun bucketLevel(`value`: StorageLensBucketLevelArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucketLevel = mapped
}
/**
* @param argument This property contains the details of the account-level bucket-level configurations for Amazon S3 Storage Lens.
*/
@JvmName("rtbctwxakwnkjuax")
public suspend fun bucketLevel(argument: suspend StorageLensBucketLevelArgsBuilder.() -> Unit) {
val toBeMapped = StorageLensBucketLevelArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.bucketLevel = mapped
}
/**
* @param value This property contains the details of account-level detailed status code metrics for S3 Storage Lens.
*/
@JvmName("otuqhssthyoqxocg")
public suspend fun detailedStatusCodesMetrics(`value`: StorageLensDetailedStatusCodesMetricsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.detailedStatusCodesMetrics = mapped
}
/**
* @param argument This property contains the details of account-level detailed status code metrics for S3 Storage Lens.
*/
@JvmName("xohiawccchjgsjhj")
public suspend fun detailedStatusCodesMetrics(argument: suspend StorageLensDetailedStatusCodesMetricsArgsBuilder.() -> Unit) {
val toBeMapped = StorageLensDetailedStatusCodesMetricsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.detailedStatusCodesMetrics = mapped
}
/**
* @param value This property determines the scope of Storage Lens group data that is displayed in the Storage Lens dashboard.
*/
@JvmName("ejidkqbkjgnpuenr")
public suspend fun storageLensGroupLevel(`value`: StorageLensGroupLevelArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageLensGroupLevel = mapped
}
/**
* @param argument This property determines the scope of Storage Lens group data that is displayed in the Storage Lens dashboard.
*/
@JvmName("nifvcfaretsaledb")
public suspend fun storageLensGroupLevel(argument: suspend StorageLensGroupLevelArgsBuilder.() -> Unit) {
val toBeMapped = StorageLensGroupLevelArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.storageLensGroupLevel = mapped
}
internal fun build(): StorageLensAccountLevelArgs = StorageLensAccountLevelArgs(
activityMetrics = activityMetrics,
advancedCostOptimizationMetrics = advancedCostOptimizationMetrics,
advancedDataProtectionMetrics = advancedDataProtectionMetrics,
bucketLevel = bucketLevel ?: throw PulumiNullFieldException("bucketLevel"),
detailedStatusCodesMetrics = detailedStatusCodesMetrics,
storageLensGroupLevel = storageLensGroupLevel,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy