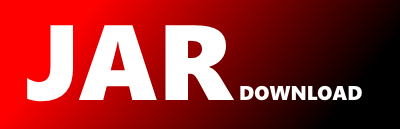
com.pulumi.awsnative.s3.kotlin.inputs.StorageLensGroupOrArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin.inputs
import com.pulumi.awsnative.s3.inputs.StorageLensGroupOrArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The Storage Lens group will include objects that match any of the specified filter values.
* @property matchAnyPrefix This property contains a list of prefixes. At least one prefix must be specified. Up to 10 prefixes are allowed.
* @property matchAnySuffix This property contains the list of suffixes. At least one suffix must be specified. Up to 10 suffixes are allowed.
* @property matchAnyTag This property contains the list of S3 object tags. At least one object tag must be specified. Up to 10 object tags are allowed.
* @property matchObjectAge This property filters objects that match the specified object age range.
* @property matchObjectSize This property contains the `BytesGreaterThan` and `BytesLessThan` values to define the object size range (minimum and maximum number of Bytes).
*/
public data class StorageLensGroupOrArgs(
public val matchAnyPrefix: Output>? = null,
public val matchAnySuffix: Output>? = null,
public val matchAnyTag: Output>? = null,
public val matchObjectAge: Output? = null,
public val matchObjectSize: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.s3.inputs.StorageLensGroupOrArgs =
com.pulumi.awsnative.s3.inputs.StorageLensGroupOrArgs.builder()
.matchAnyPrefix(matchAnyPrefix?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.matchAnySuffix(matchAnySuffix?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.matchAnyTag(
matchAnyTag?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.matchObjectAge(matchObjectAge?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.matchObjectSize(
matchObjectSize?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [StorageLensGroupOrArgs].
*/
@PulumiTagMarker
public class StorageLensGroupOrArgsBuilder internal constructor() {
private var matchAnyPrefix: Output>? = null
private var matchAnySuffix: Output>? = null
private var matchAnyTag: Output>? = null
private var matchObjectAge: Output? = null
private var matchObjectSize: Output? = null
/**
* @param value This property contains a list of prefixes. At least one prefix must be specified. Up to 10 prefixes are allowed.
*/
@JvmName("xxfxqesvauegtikb")
public suspend fun matchAnyPrefix(`value`: Output>) {
this.matchAnyPrefix = value
}
@JvmName("qthjebysbfowbdpv")
public suspend fun matchAnyPrefix(vararg values: Output) {
this.matchAnyPrefix = Output.all(values.asList())
}
/**
* @param values This property contains a list of prefixes. At least one prefix must be specified. Up to 10 prefixes are allowed.
*/
@JvmName("kddjdvebafhboxfs")
public suspend fun matchAnyPrefix(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy