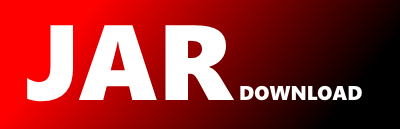
com.pulumi.awsnative.sagemaker.kotlin.inputs.ModelBiasJobDefinitionEndpointInputArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.ModelBiasJobDefinitionEndpointInputArgs.builder
import com.pulumi.awsnative.sagemaker.kotlin.enums.ModelBiasJobDefinitionEndpointInputS3DataDistributionType
import com.pulumi.awsnative.sagemaker.kotlin.enums.ModelBiasJobDefinitionEndpointInputS3InputMode
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The endpoint for a monitoring job.
* @property endTimeOffset Monitoring end time offset, e.g. PT0H
* @property endpointName An endpoint in customer's account which has enabled `DataCaptureConfig` enabled.
* @property featuresAttribute JSONpath to locate features in JSONlines dataset
* @property inferenceAttribute Index or JSONpath to locate predicted label(s)
* @property localPath Path to the filesystem where the endpoint data is available to the container.
* @property probabilityAttribute Index or JSONpath to locate probabilities
* @property probabilityThresholdAttribute The threshold for the class probability to be evaluated as a positive result.
* @property s3DataDistributionType Whether input data distributed in Amazon S3 is fully replicated or sharded by an S3 key. Defauts to FullyReplicated
* @property s3InputMode Whether the Pipe or File is used as the input mode for transfering data for the monitoring job. Pipe mode is recommended for large datasets. File mode is useful for small files that fit in memory. Defaults to File.
* @property startTimeOffset Monitoring start time offset, e.g. -PT1H
*/
public data class ModelBiasJobDefinitionEndpointInputArgs(
public val endTimeOffset: Output? = null,
public val endpointName: Output,
public val featuresAttribute: Output? = null,
public val inferenceAttribute: Output? = null,
public val localPath: Output,
public val probabilityAttribute: Output? = null,
public val probabilityThresholdAttribute: Output? = null,
public val s3DataDistributionType: Output? = null,
public val s3InputMode: Output? = null,
public val startTimeOffset: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.ModelBiasJobDefinitionEndpointInputArgs =
com.pulumi.awsnative.sagemaker.inputs.ModelBiasJobDefinitionEndpointInputArgs.builder()
.endTimeOffset(endTimeOffset?.applyValue({ args0 -> args0 }))
.endpointName(endpointName.applyValue({ args0 -> args0 }))
.featuresAttribute(featuresAttribute?.applyValue({ args0 -> args0 }))
.inferenceAttribute(inferenceAttribute?.applyValue({ args0 -> args0 }))
.localPath(localPath.applyValue({ args0 -> args0 }))
.probabilityAttribute(probabilityAttribute?.applyValue({ args0 -> args0 }))
.probabilityThresholdAttribute(probabilityThresholdAttribute?.applyValue({ args0 -> args0 }))
.s3DataDistributionType(
s3DataDistributionType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.s3InputMode(s3InputMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.startTimeOffset(startTimeOffset?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ModelBiasJobDefinitionEndpointInputArgs].
*/
@PulumiTagMarker
public class ModelBiasJobDefinitionEndpointInputArgsBuilder internal constructor() {
private var endTimeOffset: Output? = null
private var endpointName: Output? = null
private var featuresAttribute: Output? = null
private var inferenceAttribute: Output? = null
private var localPath: Output? = null
private var probabilityAttribute: Output? = null
private var probabilityThresholdAttribute: Output? = null
private var s3DataDistributionType:
Output? = null
private var s3InputMode: Output? = null
private var startTimeOffset: Output? = null
/**
* @param value Monitoring end time offset, e.g. PT0H
*/
@JvmName("tuqribxhgttmhvox")
public suspend fun endTimeOffset(`value`: Output) {
this.endTimeOffset = value
}
/**
* @param value An endpoint in customer's account which has enabled `DataCaptureConfig` enabled.
*/
@JvmName("mkqlrajyxerphyjy")
public suspend fun endpointName(`value`: Output) {
this.endpointName = value
}
/**
* @param value JSONpath to locate features in JSONlines dataset
*/
@JvmName("peyyrunmdclontov")
public suspend fun featuresAttribute(`value`: Output) {
this.featuresAttribute = value
}
/**
* @param value Index or JSONpath to locate predicted label(s)
*/
@JvmName("slmocwwwjpjooepn")
public suspend fun inferenceAttribute(`value`: Output) {
this.inferenceAttribute = value
}
/**
* @param value Path to the filesystem where the endpoint data is available to the container.
*/
@JvmName("rwharixgesdghmvw")
public suspend fun localPath(`value`: Output) {
this.localPath = value
}
/**
* @param value Index or JSONpath to locate probabilities
*/
@JvmName("jyhphlahxwqrmypi")
public suspend fun probabilityAttribute(`value`: Output) {
this.probabilityAttribute = value
}
/**
* @param value The threshold for the class probability to be evaluated as a positive result.
*/
@JvmName("ccrqbaexnwsjbjxj")
public suspend fun probabilityThresholdAttribute(`value`: Output) {
this.probabilityThresholdAttribute = value
}
/**
* @param value Whether input data distributed in Amazon S3 is fully replicated or sharded by an S3 key. Defauts to FullyReplicated
*/
@JvmName("otmfwfjirturtxwv")
public suspend fun s3DataDistributionType(`value`: Output) {
this.s3DataDistributionType = value
}
/**
* @param value Whether the Pipe or File is used as the input mode for transfering data for the monitoring job. Pipe mode is recommended for large datasets. File mode is useful for small files that fit in memory. Defaults to File.
*/
@JvmName("vqoqdvxjefypuvvt")
public suspend fun s3InputMode(`value`: Output) {
this.s3InputMode = value
}
/**
* @param value Monitoring start time offset, e.g. -PT1H
*/
@JvmName("cnmfoawmiepbgfvh")
public suspend fun startTimeOffset(`value`: Output) {
this.startTimeOffset = value
}
/**
* @param value Monitoring end time offset, e.g. PT0H
*/
@JvmName("sxpiugbxkaipivss")
public suspend fun endTimeOffset(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTimeOffset = mapped
}
/**
* @param value An endpoint in customer's account which has enabled `DataCaptureConfig` enabled.
*/
@JvmName("qakobsctjixvocws")
public suspend fun endpointName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpointName = mapped
}
/**
* @param value JSONpath to locate features in JSONlines dataset
*/
@JvmName("ijadifyfbioqunkv")
public suspend fun featuresAttribute(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.featuresAttribute = mapped
}
/**
* @param value Index or JSONpath to locate predicted label(s)
*/
@JvmName("qsfbjyaofqylqvau")
public suspend fun inferenceAttribute(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inferenceAttribute = mapped
}
/**
* @param value Path to the filesystem where the endpoint data is available to the container.
*/
@JvmName("qhmmmwkphbkwrvpk")
public suspend fun localPath(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.localPath = mapped
}
/**
* @param value Index or JSONpath to locate probabilities
*/
@JvmName("wpxrsixsarycegal")
public suspend fun probabilityAttribute(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.probabilityAttribute = mapped
}
/**
* @param value The threshold for the class probability to be evaluated as a positive result.
*/
@JvmName("sydsmorlgujgfyaa")
public suspend fun probabilityThresholdAttribute(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.probabilityThresholdAttribute = mapped
}
/**
* @param value Whether input data distributed in Amazon S3 is fully replicated or sharded by an S3 key. Defauts to FullyReplicated
*/
@JvmName("sacqtuvwumddurbx")
public suspend fun s3DataDistributionType(`value`: ModelBiasJobDefinitionEndpointInputS3DataDistributionType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3DataDistributionType = mapped
}
/**
* @param value Whether the Pipe or File is used as the input mode for transfering data for the monitoring job. Pipe mode is recommended for large datasets. File mode is useful for small files that fit in memory. Defaults to File.
*/
@JvmName("wykyqynfyffqpesb")
public suspend fun s3InputMode(`value`: ModelBiasJobDefinitionEndpointInputS3InputMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3InputMode = mapped
}
/**
* @param value Monitoring start time offset, e.g. -PT1H
*/
@JvmName("qqleaypgcrfgaegk")
public suspend fun startTimeOffset(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTimeOffset = mapped
}
internal fun build(): ModelBiasJobDefinitionEndpointInputArgs =
ModelBiasJobDefinitionEndpointInputArgs(
endTimeOffset = endTimeOffset,
endpointName = endpointName ?: throw PulumiNullFieldException("endpointName"),
featuresAttribute = featuresAttribute,
inferenceAttribute = inferenceAttribute,
localPath = localPath ?: throw PulumiNullFieldException("localPath"),
probabilityAttribute = probabilityAttribute,
probabilityThresholdAttribute = probabilityThresholdAttribute,
s3DataDistributionType = s3DataDistributionType,
s3InputMode = s3InputMode,
startTimeOffset = startTimeOffset,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy