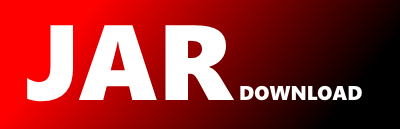
com.pulumi.awsnative.sagemaker.kotlin.inputs.ModelCardContentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.ModelCardContentArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The content of the model card.
* @property additionalInformation Additional information about the model.
* @property businessDetails Information about how the model supports business goals.
* @property evaluationDetails An overview about the model's evaluation.
* @property intendedUses The intended usage of the model.
* @property modelOverview An overview about the model
* @property modelPackageDetails
* @property trainingDetails An overview about model training.
*/
public data class ModelCardContentArgs(
public val additionalInformation: Output? = null,
public val businessDetails: Output? = null,
public val evaluationDetails: Output>? = null,
public val intendedUses: Output? = null,
public val modelOverview: Output? = null,
public val modelPackageDetails: Output? = null,
public val trainingDetails: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.ModelCardContentArgs =
com.pulumi.awsnative.sagemaker.inputs.ModelCardContentArgs.builder()
.additionalInformation(
additionalInformation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.businessDetails(businessDetails?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.evaluationDetails(
evaluationDetails?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.intendedUses(intendedUses?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.modelOverview(modelOverview?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.modelPackageDetails(
modelPackageDetails?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.trainingDetails(
trainingDetails?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ModelCardContentArgs].
*/
@PulumiTagMarker
public class ModelCardContentArgsBuilder internal constructor() {
private var additionalInformation: Output? = null
private var businessDetails: Output? = null
private var evaluationDetails: Output>? = null
private var intendedUses: Output? = null
private var modelOverview: Output? = null
private var modelPackageDetails: Output? = null
private var trainingDetails: Output? = null
/**
* @param value Additional information about the model.
*/
@JvmName("mxkjkolwnkbcempr")
public suspend fun additionalInformation(`value`: Output) {
this.additionalInformation = value
}
/**
* @param value Information about how the model supports business goals.
*/
@JvmName("sdlhanxejgyogvks")
public suspend fun businessDetails(`value`: Output) {
this.businessDetails = value
}
/**
* @param value An overview about the model's evaluation.
*/
@JvmName("tdwsnylrbgbggtuh")
public suspend fun evaluationDetails(`value`: Output>) {
this.evaluationDetails = value
}
@JvmName("ddfutiakxuubexpa")
public suspend fun evaluationDetails(vararg values: Output) {
this.evaluationDetails = Output.all(values.asList())
}
/**
* @param values An overview about the model's evaluation.
*/
@JvmName("guppinnidinnuctb")
public suspend fun evaluationDetails(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy