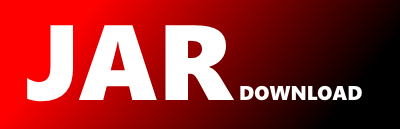
com.pulumi.awsnative.sagemaker.kotlin.inputs.ModelPackageAdditionalInferenceSpecificationDefinitionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.ModelPackageAdditionalInferenceSpecificationDefinitionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Additional Inference Specification specifies details about inference jobs that can be run with models based on this model package.AdditionalInferenceSpecifications can be added to existing model packages using AdditionalInferenceSpecificationsToAdd.
* @property containers The Amazon ECR registry path of the Docker image that contains the inference code.
* @property description A description of the additional Inference specification.
* @property name A unique name to identify the additional inference specification. The name must be unique within the list of your additional inference specifications for a particular model package.
* @property supportedContentTypes The supported MIME types for the input data.
* @property supportedRealtimeInferenceInstanceTypes A list of the instance types that are used to generate inferences in real-time
* @property supportedResponseMimeTypes The supported MIME types for the output data.
* @property supportedTransformInstanceTypes A list of the instance types on which a transformation job can be run or on which an endpoint can be deployed.
*/
public data class ModelPackageAdditionalInferenceSpecificationDefinitionArgs(
public val containers: Output>,
public val description: Output? = null,
public val name: Output,
public val supportedContentTypes: Output>? = null,
public val supportedRealtimeInferenceInstanceTypes: Output>? = null,
public val supportedResponseMimeTypes: Output>? = null,
public val supportedTransformInstanceTypes: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.ModelPackageAdditionalInferenceSpecificationDefinitionArgs =
com.pulumi.awsnative.sagemaker.inputs.ModelPackageAdditionalInferenceSpecificationDefinitionArgs.builder()
.containers(
containers.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.supportedContentTypes(supportedContentTypes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.supportedRealtimeInferenceInstanceTypes(
supportedRealtimeInferenceInstanceTypes?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.supportedResponseMimeTypes(
supportedResponseMimeTypes?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.supportedTransformInstanceTypes(
supportedTransformInstanceTypes?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
).build()
}
/**
* Builder for [ModelPackageAdditionalInferenceSpecificationDefinitionArgs].
*/
@PulumiTagMarker
public class ModelPackageAdditionalInferenceSpecificationDefinitionArgsBuilder internal constructor() {
private var containers: Output>? = null
private var description: Output? = null
private var name: Output? = null
private var supportedContentTypes: Output>? = null
private var supportedRealtimeInferenceInstanceTypes: Output>? = null
private var supportedResponseMimeTypes: Output>? = null
private var supportedTransformInstanceTypes: Output>? = null
/**
* @param value The Amazon ECR registry path of the Docker image that contains the inference code.
*/
@JvmName("scbgrosbbeboenwr")
public suspend fun containers(`value`: Output>) {
this.containers = value
}
@JvmName("vfbfbkcwkhuhkqgb")
public suspend fun containers(vararg values: Output) {
this.containers = Output.all(values.asList())
}
/**
* @param values The Amazon ECR registry path of the Docker image that contains the inference code.
*/
@JvmName("cpogmupfxmecoryh")
public suspend fun containers(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy