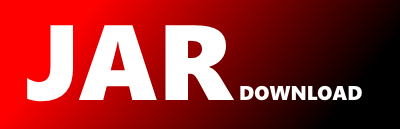
com.pulumi.awsnative.sagemaker.kotlin.inputs.SpaceSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.SpaceSettingsArgs.builder
import com.pulumi.awsnative.sagemaker.kotlin.enums.SpaceAppType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of settings that apply to spaces of Amazon SageMaker Studio. These settings are specified when the CreateSpace API is called.
* @property appType The type of app created within the space.
* @property codeEditorAppSettings The CodeEditor app settings.
* @property customFileSystems A file system, created by you, that you assign to a space for an Amazon SageMaker Domain. Permitted users can access this file system in Amazon SageMaker Studio.
* @property jupyterLabAppSettings The JupyterLab app settings.
* @property jupyterServerAppSettings The Jupyter server's app settings.
* @property kernelGatewayAppSettings The kernel gateway app settings.
* @property spaceStorageSettings Default storage settings for a space.
*/
public data class SpaceSettingsArgs(
public val appType: Output? = null,
public val codeEditorAppSettings: Output? = null,
public val customFileSystems: Output>? = null,
public val jupyterLabAppSettings: Output? = null,
public val jupyterServerAppSettings: Output? = null,
public val kernelGatewayAppSettings: Output? = null,
public val spaceStorageSettings: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.SpaceSettingsArgs =
com.pulumi.awsnative.sagemaker.inputs.SpaceSettingsArgs.builder()
.appType(appType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.codeEditorAppSettings(
codeEditorAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.customFileSystems(
customFileSystems?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.jupyterLabAppSettings(
jupyterLabAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.jupyterServerAppSettings(
jupyterServerAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.kernelGatewayAppSettings(
kernelGatewayAppSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.spaceStorageSettings(
spaceStorageSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [SpaceSettingsArgs].
*/
@PulumiTagMarker
public class SpaceSettingsArgsBuilder internal constructor() {
private var appType: Output? = null
private var codeEditorAppSettings: Output? = null
private var customFileSystems: Output>? = null
private var jupyterLabAppSettings: Output? = null
private var jupyterServerAppSettings: Output? = null
private var kernelGatewayAppSettings: Output? = null
private var spaceStorageSettings: Output? = null
/**
* @param value The type of app created within the space.
*/
@JvmName("vdrnpipdgbjyqmht")
public suspend fun appType(`value`: Output) {
this.appType = value
}
/**
* @param value The CodeEditor app settings.
*/
@JvmName("gkaboljrngdckkyp")
public suspend fun codeEditorAppSettings(`value`: Output) {
this.codeEditorAppSettings = value
}
/**
* @param value A file system, created by you, that you assign to a space for an Amazon SageMaker Domain. Permitted users can access this file system in Amazon SageMaker Studio.
*/
@JvmName("btohlptufburcrob")
public suspend fun customFileSystems(`value`: Output>) {
this.customFileSystems = value
}
@JvmName("qlfvkniuavbiohut")
public suspend fun customFileSystems(vararg values: Output) {
this.customFileSystems = Output.all(values.asList())
}
/**
* @param values A file system, created by you, that you assign to a space for an Amazon SageMaker Domain. Permitted users can access this file system in Amazon SageMaker Studio.
*/
@JvmName("kgavawhsndjvenpn")
public suspend fun customFileSystems(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy